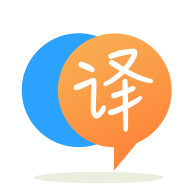
[英]Trying to create a C program that finds the reverse of a string word by word
[英]Trying to create a multi threaded C program that prints a string in reverse order
我正在為我的 C 編碼類做一個作業,它要求我們創建運行不同功能的多個線程。 為了緩解我的困惑,我試圖一次一個線程執行該程序,但遇到了一些麻煩。 這是我的代碼:
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <string.h>
void * print_string_in_reverse_order(void *str)
{
// This function is called when the new thread is created
printf("%s","In funciton start_routine(). Your string will be printed backwards.");
char *word[50];
strcpy (*word, (char *)str);
int length = strlen(*word);
for (int x = length-1; x >= 0; x--){
printf("%c",&word[x] );
}
pthread_exit(NULL); // exit the thread
}
int main(int argc, char *argv[])
{
/* The main program creates a new thread and then exits. */
pthread_t threadID;
int status;
char input[50];
printf("Enter a string: ");
scanf("%s", input);
printf("In function main(): Creating a new thread\n");
// create a new thread in the calling process
// a function name represents the address of the function
status = pthread_create(&threadID, NULL, print_string_in_reverse_order, (void *)&input);
// After the new thread finish execution
printf("In function main(): The new thread ID = %d\n", threadID);
if (status != 0) {
printf("Oops. pthread create returned error code %d\n", status);
exit(-1);
}
printf("\n");
exit(0);
}
目前,雖然我沒有編譯器錯誤,但它不會打印任何內容,也不會以任何方式反轉字符串。 我對 C 很陌生,所以我認為這類似於我的指針錯誤,但即使經過多次更改和嘗試,我似乎也無法弄清楚出了什么問題。 有沒有人有想法?
如果word
應該是一個保存字符串的char
數組,則行
char *word[50];
應改為:
char word[50];
此外,該行
strcpy (*word, (char *)str);
應改為:
strcpy (word, (char *)str);
此外,該行
printf("%c",&word[x] );
應改為:
printf("%c", word[x] );
此外,在從函數main()
返回之前,您必須等待創建的線程完成執行,添加以下行:
pthread_join( threadID, NULL );
否則,您的線程可能會提前終止。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.