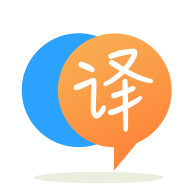
[英]How can i random.choice a question without the answer for a discord robot quiz game?
[英]I would like to create a quiz game that picks random question from list without repetition
此代碼將讓 2 名玩家從列表中隨機回答一個問題,然后比較他們的分數。
問題:
randint
問題不斷重復,嘗試使用random.shuffle
- 沒有運氣。if guess.lower() == answers[a].lower():
。elif guess.lower() != answers[a].lower():
print("Wrong!")
break
我的代碼
from random import randint
print("====== Quiz Game ======")
print("")
questions = [
"What star is in the center of the solar system?:",
"What is the 3rd planet of the solar system?:",
"What can be broken, but is never held?:",
"What part of the body you use to smell?:",
"How many days in one year?:",
"How many letters in the alphabet?:",
"Rival of Intel?:",
"No.8 element on periodic element?:",
"What is the galaxy that contains our solar system?:",
"What animal is the king of the jungle?:"
]
answers = [
"Sun",
"Earth",
"Promise",
"Nose",
"365",
"26",
"AMD",
"Oxygen",
"Milky Way",
"Lion"
]
p1score = 0
p2score = 0
def playerOne():
global p1score
for i in range(5):
q = randint(0,9)
guess = input(questions[q])
for a in range(len(answers)):
if guess.lower() == answers[a].lower():
print("Correct!")
p1score += 1
break
else:
continue
print("Your score is:",p1score)
print("")
def playerTwo():
global p2score
for i in range(5):
q = randint(0,9)
guess = input(questions[q])
for a in range(len(answers)):
if guess.lower() == answers[a].lower():
print("Correct!")
p2score += 1
break
else:
continue
print("Your score is:",p2score)
print("")
def quiz():
global p1score
global p2score
print("======Student no.1======")
playerOne()
print("======Student no.2======")
playerTwo()
if p1score > p2score:
print("Student 1 has the highest score!")
elif p1score < p2score:
print("Student 2 has the highest score!")
elif p1score == p2score:
print("Students are tied!")
else:
print("Invalid")
quiz()
您應該將問題和答案放在一起,然后您可以使用random.shuffle(list)
以隨機順序創建包含項目的列表。 然后你可以使用普通的for
-loop 來獲取問題和答案 - 它們將按隨機順序排列並且不會重復。
你有問題和答案,所以沒有問題用正確的答案檢查用戶的輸入。
import random
data = [
["What star is in the center of the solar system?:", "Sun"],
["What is the 3rd planet of the solar system?:","Earth"],
["What can be broken, but is never held?:", "Promise"],
["What part of the body you use to smell?:", "Nose"],
["How many days in one year?:","365"],
["How many letters in the alphabet?:", "26"],
["Rival of Intel?:", "AMD"],
["No.8 element on periodic element?:", "Oxygen"],
["What is the galaxy that contains our solar system?:","Milky Way"],
["What animal is the king of the jungle?:", "Lion"],
]
random.shuffle(data) # run only once
for item in data:
print('question:', item[0])
print(' answer:', item[1])
print('---')
順便說一句:你也可以這樣寫循環
for question, answer in data:
print('question:', question)
print(' answer:', answer)
print('---')
編輯:工作代碼
import random
data = [
["What star is in the center of the solar system?:", "Sun"],
["What is the 3rd planet of the solar system?:","Earth"],
["What can be broken, but is never held?:", "Promise"],
["What part of the body you use to smell?:", "Nose"],
["How many days in one year?:","365"],
["How many letters in the alphabet?:", "26"],
["Rival of Intel?:", "AMD"],
["No.8 element on periodic element?:", "Oxygen"],
["What is the galaxy that contains our solar system?:","Milky Way"],
["What animal is the king of the jungle?:", "Lion"],
]
# TODO: read data from text file or CSV file
def player(number, data):
score = 0
print("====== Student no.", number, "======")
for question, answer in data:
guess = input(question)
if guess.lower() == answer.lower():
print("Correct!\n")
score += 1
else:
print("Wrong\n")
print("Your score is: ", score, "\n")
return score
# --- main ---
random.shuffle(data) # run only once
score1 = player(1, data)
#score1 = player(1, data[:5])
random.shuffle(data) # next player will have questions in different order
score2 = player(2, data)
#score1 = player(2, data[:5])
#score3 = player(3, data) # you can have more players (but you could use list for scores)
#score4 = player(4, data) # you can have more players (but you could use list for scores)
# best_score, best_player = max([score1, 1], [score2, 2], [score3, 3], [score4, 4])
# results_in_order = sort( [[score1, 1], [score2, 2], [score3, 3], [score4, 4]], reverse=True )
if score1 > score2:
print("Student 1 has the highest score!")
elif score1 < score1:
print("Student 2 has the highest score!")
else:
print("Students are tied!")
question_prompts = [
[“蘋果是什么顏色?\\n(a)紅/綠\\n(b)橙\\n(c)紫/綠\\n(d)黑/橙”, "a"],
[“香蕉是什么顏色?\\n(a)紅/綠\\n(b)黃\\n(b)橙\\n(a)紫/綠”, 'b'],
[“聖誕節是幾月?\\n(a)九月\\n(b)十二月\\n(c)十月\\n(d)十一月”, 'b'],
[“大米是什么顏色?\\n(a)紅/綠\\n(b)黃\\n(c)紅/綠\\n(d)黃”, 'b'],
[“什么動物跑得比獅子快?\\n(a)Man\\n(b)Dear\\n(c)Red/Green\\n(d)Yellow”, 'b'],
[“世界上有多少個大陸?\\n(a) 七塊\\n(b) 九塊\\n(c)紅/綠\\n(d)黃”, 'a'],
["彩虹有多少種顏色?\\n(a)七\\n(b)五\\n(c)紅/綠\\n(d)黃", 'a'],
[“我叫什么名字?\\n(a) Nick\\n(b)Chioma\\n(c)Tiger\\n(d)Greatness”, 'a'],
[“大流行發生在哪一年?\\n(a)2020\\n(b)2019\\n(c)2021\\n(d)2023”, 'a'],
[“地球大約有多少歲?\\n(a)6b 年\\n(b)6.4b 年\\n(c)6b 年\\n(d)6.4b 年”,'b']
]
計數 = 0
樣本 = 樣本(問題提示,4)
對於樣本中的 i, j:
print(i)
answer = input("Enter your answer: ").lower()
if answer == j:
count += 1
print("Right, Your score: ", count)
print('')
else:
print("Wrong, correct answer is: ", j)
print()
打印(“你有”,計數,“出”,len(樣本),“問題”)
這對我有用。 晚點再謝我
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.