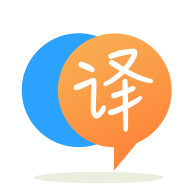
[英]failing to display the display window due to the pygame.display.update()
[英]How to make the pygame.display.update () method keep displaying the window?
我使用 pygame 模塊制作了一個黑白棋游戲。 這個腳本有效,但有一個小問題。 我要實現的效果是在玩家移動后顯示此招式的結果,暫停一段時間(例如2秒),然后顯示電腦的招式和結果。 但是在運行我的腳本后,計算機的移動和結果會在玩家移動后立即顯示。 我試過 time.sleep() 但沒有用。 經過測試,我找到了問題的原因。 pygame.display.update() 只能顯示一幀畫面。 如果沒有事件循環,屏幕將無法連續顯示。 如何解決這個問題呢?
我是編程初學者,知道自己寫的代碼不夠流暢高效。 這是我的代碼。 我認為問題在於最后幾行代碼。
import pygame, os, time, random
from pygame.locals import *
def initBoardList():
boardList = []
for x in range(8):
boardList.append([])
for y in range(8):
boardList[x].append(' ')
boardList[3][3] = 'X'
boardList[3][4] = 'O'
boardList[4][3] = 'O'
boardList[4][4] = 'X'
return boardList
def move(boardList, coordinate, symbol):
if isValidMove(boardList, coordinate, symbol):
x = coordinate[0] - 1
y = coordinate[1] - 1
boardList[x][y] = symbol
flipableTiles = detectaAll(boardList, coordinate, symbol)
flipTile(boardList, flipableTiles)
def drawBoard(boardList, boardSurface, tileColour1, tileColour2):
for x in range(8):
for y in range(8):
if boardList[x][y] == 'O':
pygame.draw.circle(boardSurface, tileColour1, (80 * x + 120, 80 * y + 120), 30, 0)
if boardList[x][y] == 'X':
pygame.draw.circle(boardSurface, tileColour2, (80 * x + 120, 80 * y + 120), 30, 0)
def isFree(boardList, coodinate):
x = coodinate[0] - 1
y = coodinate[1] - 1
return boardList[x][y] == ' '
def isFull(boardList):
for x in range(8):
for y in range(8):
if boardList[x][y] == ' ':
return False
return True
def flipTile(boardList, flipableTiles):
for coordinate in flipableTiles:
x = coordinate[0] - 1
y = coordinate[1] - 1
if boardList[x][y] == 'X':
boardList[x][y] = 'O'
elif boardList[x][y] == 'O':
boardList[x][y] = 'X'
def reverseTile(symbol):
if symbol == 'O':
return 'X'
if symbol == 'X':
return 'O'
def detectOnedirection(boardList, coordinate, symbol, delta):
flipableTiles = []
deltaX = delta[0]
deltaY = delta[1]
x = coordinate[0] -1
y = coordinate[1] -1
while True:
x += deltaX
y += deltaY
if x < 0 or x > 7 or y < 0 or y > 7 or boardList[x][y] == ' ':
return []
elif boardList[x][y] == reverseTile(symbol):
flipableTiles.append((x+1, y+1))
elif boardList[x][y] == symbol:
return flipableTiles
def detectaAll(boardList, coordinate, symbol):
deltas = [(0, 1),(0, -1),(1, 1),(1, -1),(1, 0),(-1, 0),(-1, 1),(-1, -1)]
flipableTiles = []
for delta in deltas:
flipableTiles += detectOnedirection(boardList, coordinate, symbol, delta)
return flipableTiles
def getComputerMove(boardList,symbol):
bestMove = None
highestScore = 0
cMoveable = moveable(boardList, symbol)
for coordinate in cMoveable:
if coordinate in [(1,1),(1,8),(8,1),(8,8)]:
return coordinate
else:
score = len(detectaAll(boardList, coordinate, symbol))
if score > highestScore:
bestMove = coordinate
highestScore = score
return bestMove
def isValidMove(boardList, coordinate, symbol):
x = coordinate[0] -1
y = coordinate[1] -1
return 0 < coordinate[0] < 9 and 0 < coordinate[1] < 9 and \
isFree(boardList, coordinate) and len(detectaAll(boardList, coordinate, symbol)) != 0
def countScore(boardList, symbol):
score = 0
for x in range(8):
for y in range(8):
if boardList[x][y] == symbol:
score += 1
return score
def moveable(boardList, symbol):
moveable = []
for y in range(1,9):
for x in range(1,9):
if detectaAll(boardList, (x, y), symbol) != [] and isFree(boardList, (x,y)):
moveable.append((x, y))
random.shuffle(moveable)
return moveable
boardList = initBoardList()
black = (0, 0, 0)
white = (255, 255, 255)
blue = (0, 0, 255)
turn = 'player'
pygame.init()
boardSurface = pygame.display.set_mode((800,800), 0, 32)
boardSurface.fill(blue)
pygame.display.set_caption('Reversi')
for i in range(9):
pygame.draw.line(boardSurface, black, (80, 80 + 80*i), (720, 80 + 80*i), 2)
pygame.draw.line(boardSurface, black, (80 + 80*i, 80), (80 + 80*i, 720), 2)
drawBoard(boardList, boardSurface, black, white)
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
os._exit(1)
if event.type == KEYUP:
if event.key == K_ESCAPE:
pygame.quit()
os._exit(1)
if event.type == MOUSEBUTTONUP and turn == 'player':
x = (event.pos[0] - 80)// 80 + 1
y = (event.pos[1] - 80)// 80 + 1
if isValidMove(boardList, (x, y), 'O'):
move(boardList, (x, y), 'O')
turn = 'computer'
drawBoard(boardList, boardSurface, black, white)
pygame.display.update()
if turn == 'computer':
cm = getComputerMove(boardList, 'X')
move(boardList, cm, 'X')
turn = 'player'
drawBoard(boardList, boardSurface, black, white)
pygame.display.update()
你最初把 time.sleep() 放在哪里? 我把它放在這里:
if turn == 'computer':
cm = getComputerMove(boardList, 'X')
move(boardList, cm, 'X')
turn = 'player'
drawBoard(boardList, boardSurface, black, white)
time.sleep(2)
pygame.display.update()
它工作正常。 計算機不再在您之后立即播放。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.