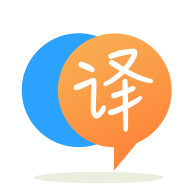
[英]How to unpack numbers in cpp if they are pack at python using struct.pack(fmt, v1, v2, …)
[英]Suppose we have two std::vectors v1 and v2 and we dont want to combine these in a struct. How to transform v2 the same way v1 was transformed by sort?
這是這個問題的后續。 唯一的區別是限制兩個向量不能組合在一個結構中。
假設我們有一個向量
std::vector<double> v1 = {9.0,5.0,3.0,2.0,1.0};
現在我們對向量v1
排序。 讓v2
由
std::vector<std::string> v2 = {"you?","are","how","there","hello"};
如何以與通過排序轉換 v1 相同的方式轉換 v2?
基於此答案,您可以使用索引數組對雙精度向量進行“排序”,並僅使用生成的索引數組來索引字符串向量。
#include <algorithm>
#include <iostream>
#include <string>
#include <numeric>
int main()
{
std::vector<double> v1 = {5.0,9.0,3.0,2.0,1.0};
std::vector<std::string> v2 = {"are", "you?","how","there","hello"};
// Create an array of indices, starting from 0
std::vector<int> index(v1.size());
std::iota(index.begin(), index.end(), 0);
// "Sort" the index array according to the value in the vector of doubles
std::sort(index.begin(), index.end(),
[&](int n1, int n2){ return v1[n1] < v1[n2]; });
// Output results
for (auto i : index )
std::cout << v2[i] << " " << v1[i] << ", index is " << i << "\n";
}
輸出:
hello 1, index is 4
there 2, index is 3
how 3, index is 2
are 5, index is 0
you? 9, index is 1
筆記:
我更改了原始數據以說明索引數組的工作原理。
您缺少的抽象是將向量視為一項的能力。 這就是索引向量在另一個答案中的作用。
我認為值得一提的是,有些庫提供了這樣的概念(通常以“zip”為名)。 例如,使用 range-v3:
std::vector<double> v1 = {5, 9, 3, 2, 1};
std::vector<std::string> v2 = {"are", "you?", "how", "there", "hello"};
// Sort the vectors
ranges::actions::sort(ranges::views::zip(v1, v2));
// Output results
for (std::size_t i = 0; i < v1.size(); ++i)
std::cout << v2[i] << " " << v1[i] << ", index is " << i << "\n";
一個可能的解決方案使用輔助std::vector<int>
:
#include <iostream>
#include <vector>
#include <algorithm>
#include <stdexcept>
template<typename T>
void MySort(std::vector<T> t, std::vector<int>& helper)
{
struct StructHelper
{
T t1;
int num;
StructHelper(T t, int i): t1{t}, num{i} {};
bool operator<(const StructHelper& other) const
{ return t1 < other.t1; }
};
std::vector<StructHelper> shVector;
for(int i=0; i<t.size(); ++i)
{
shVector.emplace_back(t[i], i);
}
std::sort(shVector.begin(), shVector.end());
helper = std::vector<int>(t.size());
for(int i=0; i<t.size(); ++i)
{
helper[i] = shVector[i].num;
}
}
template<typename T>
void MySortUsingHelper(std::vector<T>& t1, const std::vector<int>& helper)
{
if(t1.size() != helper.size()) throw std::out_of_range("not same size");
std::vector<T> t2(t1.size());
for(int i=0; i<helper.size(); ++i)
{
t2[i] = t1[helper[i]];
}
t1 = t2;
}
int main() {
std::vector<double> v1 = {9.0,5.0,3.0,2.0,1.0};
std::vector<int> helper;
MySort(v1, helper);
std::vector<std::string> v2 = {"you?","are","how","there","hello"};
MySortUsingHelper(v2, helper);
for(auto elem : v2)
{
std::cout << elem << " ";
}
return 0;
}
可以在線運行上面的代碼,看到如下輸出:
hello there how are you?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.