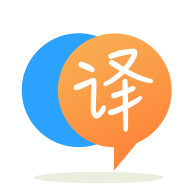
[英]How to make text appear in next line when it overflows the parent div using css or javascript?
[英]How to display show more only when text overflows from a div using pure css javascript in reactjs
我有一個獲取動態文本的組件。 當文本溢出 div 的寬度時,我顯示省略號。 現在,我正在show more
,當用戶點擊它時,我將顯示整個文本並show less
鏈接。
import React from "react";
import "./styles.css";
export default function App(props) {
const [showMore, setShowMore] = React.useState(true);
const onClick = () => {
setShowMore(!showMore);
}
return (
<>
<div className={showMore ? '' : "container"}>{props.text}</div>
<span className="link" onClick={onClick}>{showMore ? 'show less' : 'show more'}</span>
</>
);
}
.container {
overflow-x: hidden;
text-overflow: ellipsis;
white-space: nowrap;
display: block !important;
width: 250px;
background-color: #eaeaea;
}
即使文本沒有溢出,上面的代碼也會show more
鏈接。
但我只想在文本溢出時show more
鏈接。 我怎樣才能做到這一點?
編輯
從 crays 的評論中,我發現了這個stackoverflow anwser
現在,我嘗試使用 ref 訪問樣式如下
import React from "react";
import "./styles.css";
export default function App(props) {
const [showMore, setShowMore] = React.useState(false);
const onClick = () => {
setShowMore(!showMore);
};
const checkOverflow = () => {
const el = ref.current;
const curOverflow = el.style.overflow;
if ( !curOverflow || curOverflow === "visible" )
el.style.overflow = "hidden";
const isOverflowing = el.clientWidth < el.scrollWidth
|| el.clientHeight < el.scrollHeight;
el.style.overflow = curOverflow;
return isOverflowing;
};
const ref = React.createRef();
return (
<>
<div ref={ref} className={showMore ? "container-nowrap" : "container"}>
{props.text}
</div>
{(checkOverflow()) && <span className="link" onClick={onClick}>
{showMore ? "show less" : "show more"}
</span>}
</>
)
}
我也試過使用forwardref
export const App = React.forwardRef((props, ref) => {
const [showMore, setShowMore] = React.useState(false);
const onClick = () => {
setShowMore(!showMore);
};
const checkOverflow = () => {
const el = ref.current;
const curOverflow = el.style.overflow;
if (!curOverflow || curOverflow === "visible") el.style.overflow = "hidden";
const isOverflowing =
el.clientWidth < el.scrollWidth || el.clientHeight < el.scrollHeight;
el.style.overflow = curOverflow;
return isOverflowing;
};
return (
<>
<div ref={ref} className={showMore ? "container-nowrap" : "container"}>
{props.text}
</div>
{checkOverflow() && (
<span className="link" onClick={onClick}>
{showMore ? "show less" : "show more"}
</span>
)}
</>
);
});
但我收到錯誤Cannot read property 'style' of null
看看這篇文章,它使用“復選框黑客”來做到這一點。
我認為您可能會采用另一種方法,而不是取決於瀏覽器內部視覺上發生的事情
假設文本僅在您有超過 N 個字符(由您自己定義此數字)時才會溢出,例如 100 個字符
import {useState} from 'react';
export const Accordion = ({ text, limit = 100 }) => {
const isExpandable = text.length > limit;
const [viewText, setViewText] = useState(
isExpandable ? text.slice(0, limit) : text
);
const isExpanded = text.length === viewText.length;
return (
<>
<p>{viewText}</p>
{isExpandable && (
<button
onClick={() => setViewText(isExpanded ? text.slice(0, limit) : text)}
>
{isExpanded ? "show less" : "show more"}
</button>
)}
</>
);
};
代碼沙盒鏈接在這里
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.