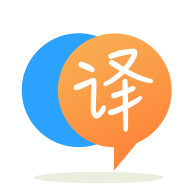
[英]How can I index child object properties in an array using ydn-db-fulltext?
[英]How can I index object properties?
我有一個包含人名及其服務時間的對象列表。 這些名稱在整個列表中可能出現多次,並且每次出現的服務時間可能不同。 我想要做的是創建一個名稱的 HashSet 並附加一個索引,這樣我就可以將它引用回更大的列表,而不必創建嵌套的 for 循環。 例如,如果我有列表:
[{Name: 'john doe', ServiceTime: '20min'}, {Name: 'john doe', ServiceTime: '25min'},
{Name: 'john doe', ServiceTime: '31min'}, {Name: 'billy bob', ServiceTime: '1min'},
{Name: 'billy bob', ServiceTime: '12min'}, {Name: 'john doe', ServiceTime: '19min'}]
我創建了我的 HashSet 看起來像這樣:
{'john doe': 0, 'billy bob': 1}
這是我的做法:
let count = 0;
list.map(obj => arr[obj.Name] = arr[obj.Name] ? arr[obj.Name] : count++)
每次找到新人時,鍵的值都會增加,如果這是有道理的。 完成后,我遍歷我創建的新名稱對象,以創建一個對象數組,該數組將保存人員姓名和一個數組來保存他們的服務時間,如下所示:
[{Name: 'john doe', ServiceTimes:[]}, {Name: 'billy bob', ServiceTimes:[]}]
有了這些信息,我可以返回並迭代我的初始列表,更新索引以通過我的 HashSet 的鍵值對進行搜索,如下所示:
list.map(obj => {
let index = arr[obj.Name];
if(temp[index] !== undefined){
temp[index].ServiceTimes.push(obj)
}
})
我面臨的問題是,在創建我的 HashSet 之后,我注意到我的索引出現了 1 個錯誤。 例如,我上面提供的列表給了我 HashSet:
{'john doe': 1, 'billy bob': 2}
和其他列表我得到了 HashSet
{'john doe': 0, 'billy bob': 1}
我顯然希望我的 HashSet 看起來像最后一個,但似乎無法理解為什么我的計數在某些情況下被添加了兩次,而在其他情況下卻沒有。 如果有人能指出我正確的方向或了解我可能做錯了什么導致了這個問題,我將不勝感激。 謝謝!
PS 抱歉問了這么長的問題。
您可以只使用reduce()
來迭代數組。 當您運行減速器時,您可以在其中執行任何操作。 如下所示。
let data = [ {Name: 'john doe', ServiceTime: '20min'}, {Name: 'john doe', ServiceTime: '25min'}, {Name: 'john doe', ServiceTime: '31min'}, {Name: 'billy bob', ServiceTime: '1min'}, {Name: 'billy bob', ServiceTime: '12min'}, {Name: 'john doe', ServiceTime: '19min'}]; let mappedData = data.reduce((accumulator, currentItem, index) => { const {Name, ServiceTime} = currentItem; if(!accumulator.hasOwnProperty(Name)) { accumulator[Name] = { firstMatchedAtindex: index, count: 0, serviceTimes: [] }; } accumulator[Name].count += 1 ; accumulator[Name].serviceTimes.push({index, ServiceTime}); return accumulator; }, {}) console.log(mappedData);
更簡單的腳本...
let data = [ {Name: 'john doe', ServiceTime: '20min'}, {Name: 'john doe', ServiceTime: '25min'}, {Name: 'john doe', ServiceTime: '31min'}, {Name: 'billy bob', ServiceTime: '1min'}, {Name: 'billy bob', ServiceTime: '12min'}, {Name: 'john doe', ServiceTime: '19min'}]; let mappedData = data.reduce((accumulator, currentItem, index) => { const {Name, ServiceTime} = currentItem; if(!accumulator.hasOwnProperty(Name)) { accumulator[Name] = index; } return accumulator; }, {}) console.log(mappedData);
另請注意數組map
回調函數可讓您訪問索引。 例如
const list = [ {Name: 'john doe', ServiceTime: '20min'}, {Name: 'john doe', ServiceTime: '25min'}, {Name: 'john doe', ServiceTime: '31min'}, {Name: 'billy bob', ServiceTime: '1min'}, {Name: 'billy bob', ServiceTime: '12min'}, {Name: 'john doe', ServiceTime: '19min'}]; const transformed = list.map((obj, index) => { return { ...obj, index } }); console.log(transformed); // Same with forEach alternative to for loop list.forEach((obj, index) => { console.log(obj, index); });
let count = 0;
list.map(obj => arr[obj.Name] = arr[obj.Name] ? arr[obj.Name] : count++)
您永遠不會重置count
變量。 您應該在找到新人名時重置計數變量。
let data = [
{Name: 'john doe', ServiceTime: '20min'},
{Name: 'john doe', ServiceTime: '25min'},
{Name: 'john doe', ServiceTime: '31min'},
{Name: 'billy bob', ServiceTime: '1min'},
{Name: 'billy bob', ServiceTime: '12min'},
{Name: 'john doe', ServiceTime: '19min'}
];
let final = {};
let found = 0;
data.forEach((obj, index) => {
if (!final.hasOwnProperty(obj.Name)) {
final[obj.Name] = found;
found += 1;
return;
}
})
console.log(final);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.