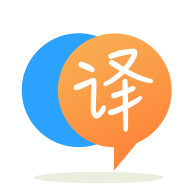
[英]Sorting an array of objects based on relevance of a key-value pair (JavaScript)
[英]How can I separate similar or alike items in an array of objects based on a specific key-value pair for each item?
我得到了一組對象。 數組中的每個對象都有一個關鍵的 PlanType...我需要確保沒有具有相同 PlanType 的對象彼此相鄰...
即使有時無法完全分離類似的項目,我該如何實現這一點。
[{Item: 1,PlanType: A}, {Item: 2,PlanType: B}, {Item: 3,PlanType: C}, {Item: 4,PlanType: C}, {Item: 5,PlanType: A}, {Item: 6,PlanType: A}, {Item: 7,PlanType: B}, {Item: 8,PlanType: A}, {Item: 9,PlanType: C}, {Item: 10,PlanType: A}]
預期結果...
[{Item: 1,PlanType: A}, {Item: 2,PlanType: B}, {Item: 3,PlanType: C}, {Item: 5,PlanType: A}, {Item: 4,PlanType: C}, {Item: 6,PlanType: A}, {Item: 7,PlanType: B}, {Item: 8,PlanType: A}, {Item: 9,PlanType: C}, {Item: 10,PlanType: A}]
這是我試過的代碼......
//Sort the array into an object of arrays by PlanType
let plan_types = {};
original_array.forEach(item => {
if(plan_types[item.plan_type] === undefined){
Object.assign(plan_types, {
[item.plan_types]: []
})
}
plan_types[item.plan_types].push(item);
});
//Loop through the list of Plan types and try to evenly space out the items across a new array of the same size
let new_array = new Array(original_array.length).fill(null);
Object.keys(program_types).forEach((item,index) => {
let new_array_index = 0;
let item_index = 0;
const frequency = Math.ceil(new_array.length / plan_types[item].length);
while(new_array_index < new_array.length){
if(new_array[new_array_index] !== null) new_array_index ++;
else{
new_array[new_array_index] = plan_types[item][item_index];
new_array_index += frequency;
item_index ++;
}
}
})
問題是你一直通過它,它錯過了填充一些項目。 留下空點和項目被遺漏。
您可以這樣做,這可能不是最有效的方法,但它可以幫助您解決問題。
let A='A', B='B', C='C', D='D';
let f = [{ Item: 1, PlanType: A }, { Item: 2, PlanType: B }, { Item: 3, PlanType: C }, { Item: 4, PlanType: C }, { Item: 5, PlanType: A }, { Item: 6, PlanType: A }, { Item: 7, PlanType: B }, { Item: 8, PlanType: A }, { Item: 9, PlanType: C }, { Item: 10, PlanType: A } ];
let prevItem=f[0];
let currentItem;
for(let i=1; i<f.length; i++){
currentItem = f[i];
if(currentItem.PlanType === prevItem.PlanType){
f.splice(i,1);
f.push(currentItem);
console.log("Item shifted");
};
prevItem = currentItem;
}
console.log(f);
我在解決算法方面很糟糕,但這就是我想出的。 它還處理具有相同鍵的多個對象的情況,一個接一個。
基本上我已經從一個數組中創建了另一個數組,我只是檢查當前迭代中的項目是否與我的新數組中的相同。 如果是,請將其添加到“堆棧”中。 每當第一個條件失敗時,它將繼續將當前項插入到新數組中。 這樣做之后,我只是檢查是否可以從該堆棧中獲取一個項目並將其插入到新數組中,並將其從重復項數組中刪除。
const items = [
{ Item: 1, PlanType: 'A' },
{ Item: 2, PlanType: 'B' },
{ Item: 3, PlanType: 'C' },
{ Item: 4, PlanType: 'C' },
{ Item: 5, PlanType: 'C' },
{ Item: 6, PlanType: 'A' },
{ Item: 7, PlanType: 'A' },
{ Item: 8, PlanType: 'B' },
{ Item: 9, PlanType: 'A' },
{ Item: 10, PlanType: 'C' },
{ Item: 11, PlanType: 'A' }
];
function normalizeList(items) {
if (items.length < 1) return items;
const result = [];
const duplicateItems = [];
items.forEach((item, index) => {
if (result.length && item.PlanType === result[result.length - 1].PlanType) {
if(index === items.length - 1) result.push(item);
else duplicateItems.push(item);
}
else {
result.push(item);
if(duplicateItems.length && duplicateItems[0].PlanType !== item.PlanType) {
result.push(duplicateItems.shift());
}
}
});
return result;
}
在我看來,將價值相等(或子財產價值相等)的相鄰物品可靠地相互遠離,不能通過基本比較來完成。 將要提出的方法不能稱為優雅,因為它在很大程度上基於直接數組變異和計數索引。 它旨在解決第一次迭代步驟中的任務,例如......
relocateEqualNeighboringItemValues(["A", "A", "B"]) => ["A", "B", "A"]
relocateEqualNeighboringItemValues(["A", "A", "B", "C", "C"]) => ["A", "C", "B", "A", "C"]
通過第二個迭代步驟,還可以傳遞一個getter
,例如,對於更復雜結構的項目,確實針對項目的特定屬性......
relocateEqualNeighboringItemValues([
{ item: 1, planType: "A" },
{ item: 2, planType: "A" },
{ item: 3, planType: "B" }
], (item => item.planType)) => [
{ item: 1, planType: "A" },
{ item: 3, planType: "B" },
{ item: 2, planType: "A" }
]
實現看起來像這樣......
function relocateEqualNeighboringItemValues(arr, getItemValue) {
if (Array.isArray(arr) && (arr.length >= 3)) {
const getValue = ((
(typeof getItemValue === 'function') &&
(item => (item && getItemValue(item)))
) || (item => item)); // getter default.
let isTerminateRelocation = false;
let isRelocateItem;
let offsetCount;
let itemCount;
let itemValue;
let leftToRightIndex;
let rightToLeftIndex = arr.length;
while (!isTerminateRelocation && (--rightToLeftIndex >= 0)) {
isRelocateItem = false;
itemValue = getValue(arr[rightToLeftIndex]);
// - Exercise repeatedly from right to left for each item a relocation lookup,
// and, if possible, try to relocated such an equal neighboring item (value).
if (itemValue === getValue(arr[rightToLeftIndex - 1])) {
offsetCount = 1;
isRelocateItem = true;
while (isRelocateItem && ((rightToLeftIndex - (++offsetCount)) >= 0)) {
if (
(itemValue !== getValue(arr[rightToLeftIndex - offsetCount])) &&
(itemValue !== getValue(arr[rightToLeftIndex - offsetCount - 1]))
) {
arr.splice((rightToLeftIndex - offsetCount), 0, arr.splice(rightToLeftIndex, 1)[0]);
++rightToLeftIndex; // reset ... start look-up from the former entering position.
isRelocateItem = false;
}
}
// - In case the right to left relocation for a specific item got stuck (reached array boundaries),
// change lookup direction exclusively for this item from left to right and try relocating it.
// - Does the relocation attempt fail yet again, nothing can be done. The process will terminate.
if (isRelocateItem && ((rightToLeftIndex - offsetCount) < 0)) {
offsetCount = 1;
itemCount = arr.length;
leftToRightIndex = Math.max(0, (rightToLeftIndex - 1));
itemValue = getValue(arr[leftToRightIndex]);
isRelocateItem = (itemValue === getValue(arr[leftToRightIndex + 1]));
while (isRelocateItem && ((leftToRightIndex + (++offsetCount)) < itemCount)) {
if (
(itemValue !== getValue(arr[leftToRightIndex + offsetCount])) &&
(itemValue !== getValue(arr[leftToRightIndex + offsetCount + 1]))
) {
arr.splice((leftToRightIndex + offsetCount), 0, arr.splice(leftToRightIndex, 1)[0]);
isRelocateItem = false;
}
}
if (isRelocateItem && ((leftToRightIndex + offsetCount) >= itemCount)) {
isTerminateRelocation = true;
}
}
}
}
}
return arr;
}
測試用例
基本的
function relocateEqualNeighboringItemValues(a,b){if(Array.isArray(a)&&3<=a.length){const c="function"==typeof b&&(a=>a&&b(a))||(a=>a);let d,e,f,g,h,i=!1,j=a.length;for(;!i&&0<=--j;)if(d=!1,g=c(a[j]),g===c(a[j-1])){for(e=1,d=!0;d&&0<=j-++e;)g!==c(a[je])&&g!==c(a[je-1])&&(a.splice(je,0,a.splice(j,1)[0]),++j,d=!1);if(d&&0>je){for(e=1,f=a.length,h=Math.max(0,j-1),g=c(a[h]),d=g===c(a[h+1]);d&&h+ ++e<f;)g!==c(a[h+e])&&g!==c(a[h+e+1])&&(a.splice(h+e,0,a.splice(h,1)[0]),d=!1);d&&h+e>=f&&(i=!0)}}}return a} console.log('["A", "A", "B"] => ', relocateEqualNeighboringItemValues(["A", "A", "B"])); console.log('["A", "B", "B"] => ', relocateEqualNeighboringItemValues(["A", "B", "B"])); console.log('["A", "A", "B", "C", "C"] => ', relocateEqualNeighboringItemValues(["A", "A", "B", "C", "C"])); console.log('["A", "A", "C", "B", "C", "C"] => ', relocateEqualNeighboringItemValues(["A", "A", "C", "B", "C", "C"])); console.log('["A", "A", "C", "C", "B", "C", "C"] => ', relocateEqualNeighboringItemValues(["A", "A", "C", "C", "B", "C", "C"]));
.as-console-wrapper { max-height: 100%!important; top: 0; }
復雜,具有 getter 功能但可解析
function relocateEqualNeighboringItemValues(a,b){if(Array.isArray(a)&&3<=a.length){const c="function"==typeof b&&(a=>a&&b(a))||(a=>a);let d,e,f,g,h,i=!1,j=a.length;for(;!i&&0<=--j;)if(d=!1,g=c(a[j]),g===c(a[j-1])){for(e=1,d=!0;d&&0<=j-++e;)g!==c(a[je])&&g!==c(a[je-1])&&(a.splice(je,0,a.splice(j,1)[0]),++j,d=!1);if(d&&0>je){for(e=1,f=a.length,h=Math.max(0,j-1),g=c(a[h]),d=g===c(a[h+1]);d&&h+ ++e<f;)g!==c(a[h+e])&&g!==c(a[h+e+1])&&(a.splice(h+e,0,a.splice(h,1)[0]),d=!1);d&&h+e>=f&&(i=!0)}}}return a} const items = [ { Item: 0, PlanType: 'B' }, { Item: 1, PlanType: 'B' }, { Item: 2, PlanType: 'A' }, { Item: 3, PlanType: 'C' }, { Item: 4, PlanType: 'C' }, { Item: 5, PlanType: 'C' }, { Item: 6, PlanType: 'A' }, { Item: 7, PlanType: 'A' }, { Item: 8, PlanType: 'B' }, { Item: 9, PlanType: 'A' }, { Item: 10, PlanType: 'A' }, { Item: 11, PlanType: 'A' }, { Item: 12, PlanType: 'A' } // { Item: 13, PlanType: 'A' } ]; console.log(items, ' => ', relocateEqualNeighboringItemValues(Array.from(items).reverse(), (item => item.PlanType)).reverse()); console.log(items, ' => ', relocateEqualNeighboringItemValues(Array.from(items).reverse(), (item => item.PlanType))); console.log(items, ' => ', relocateEqualNeighboringItemValues(Array.from(items), (item => item.PlanType)).reverse()); console.log(items, ' => ', relocateEqualNeighboringItemValues(Array.from(items), (item => item.PlanType)));
.as-console-wrapper { max-height: 100%!important; top: 0; }
復雜,具有 getter 函數但不再可解析
function relocateEqualNeighboringItemValues(a,b){if(Array.isArray(a)&&3<=a.length){const c="function"==typeof b&&(a=>a&&b(a))||(a=>a);let d,e,f,g,h,i=!1,j=a.length;for(;!i&&0<=--j;)if(d=!1,g=c(a[j]),g===c(a[j-1])){for(e=1,d=!0;d&&0<=j-++e;)g!==c(a[je])&&g!==c(a[je-1])&&(a.splice(je,0,a.splice(j,1)[0]),++j,d=!1);if(d&&0>je){for(e=1,f=a.length,h=Math.max(0,j-1),g=c(a[h]),d=g===c(a[h+1]);d&&h+ ++e<f;)g!==c(a[h+e])&&g!==c(a[h+e+1])&&(a.splice(h+e,0,a.splice(h,1)[0]),d=!1);d&&h+e>=f&&(i=!0)}}}return a} const items = [ { Item: 0, PlanType: 'B' }, { Item: 1, PlanType: 'B' }, { Item: 2, PlanType: 'A' }, { Item: 3, PlanType: 'C' }, { Item: 4, PlanType: 'C' }, { Item: 5, PlanType: 'C' }, { Item: 6, PlanType: 'A' }, { Item: 7, PlanType: 'A' }, { Item: 8, PlanType: 'B' }, { Item: 9, PlanType: 'A' }, { Item: 10, PlanType: 'A' }, { Item: 11, PlanType: 'A' }, { Item: 12, PlanType: 'A' }, { Item: 13, PlanType: 'A' } ]; console.log(items, ' => ', relocateEqualNeighboringItemValues(Array.from(items), (item => item.PlanType)));
.as-console-wrapper { max-height: 100%!important; top: 0; }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.