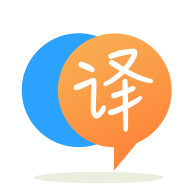
[英]Div is set to height 100% with scroll-y: hidden, BUT how do i get a child div to have scrollable?
[英]How get 2 divs same height using scroll-y and flexbox
我希望這個可滾動的 div 與它旁邊的 div 具有相同的高度。 這是我到目前為止得到的:
<div style="display: flex">
<div>
The height must be determined by this div.
</div>
<div style="overflow-y: scroll">
This div has a lot of content.
The height must follow the div next to me.
</div>
</div>
不幸的是,正確的 div 總是與其內容一樣大。 兩個div都有動態內容,所以我不知道確切的高度。
首先閱讀她之間的差異: overflow-y: scroll
到auto
(在您的情況下最好使用自動)。 https://developer.mozilla.org/en-US/docs/Web/CSS/overflow
再做一個前期的align-items: flex-start;
(像這樣 col 的高度將不匹配)。
.parent {
display: flex;
align-items: flex-start;
}
/* set max-height by code */ var colHeight = document.getElementById("child_1").getBoundingClientRect().height; console.log(colHeight); document.getElementById("child_2").style.maxHeight = colHeight+"px";
.parent { display: flex; } #child_1{ border: 3px solid orange; } #child_2 { overflow-y: auto; border: 2px solid violet; }
<main class='parent'> <div id="child_1"> <p> Fit to content in all cases - Fit to content in all cases - Fit to content in all cases - Fit to content in all cases </p> <p> Fit to content in all cases - Fit to content in all cases - Fit to content in all cases - Fit to content in all cases </p> </div> <div id="child_2"> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> </div> </main>
當您通過代碼設置max-height
- 您應該在每次瀏覽器調整大小時運行它(響應式網站的更安全方法): 如何在每次調整 HTML 元素大小時運行 JavaScript 函數?
function resize() { /* set max-height by code */ var colHeight = document.getElementById("child_1").getBoundingClientRect().height; console.log(colHeight); document.getElementById("child_2").style.maxHeight = colHeight+"px"; console.log('resized'); } resize(); window.onresize = resize;
.parent { display: flex; align-items: flex-start; } #child_1{ border: 3px solid orange; } #child_2 { overflow-y: auto; border: 2px solid violet; }
<main class='parent'> <div id="child_1"> <p> Fit to content in all cases - Fit to content in all cases - Fit to content in all cases - Fit to content in all cases </p> <p> Fit to content in all cases - Fit to content in all cases - Fit to content in all cases - Fit to content in all cases </p> </div> <div id="child_2"> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> </div> </main>
額外閱讀:
這個max-height
技巧在小屏幕上不是很有用。 一種解決方案(如果窗口寬度高於 X 運行函數)。
function resize() {
/* set max-height by code */
if (window.innerWidth > 960) {
var colHeight = document.getElementById("child_1").getBoundingClientRect().height;
console.log("window width" + window.innerWidth +"px");
document.getElementById("child_2").style.maxHeight = colHeight+"px";
}
}
resize();
window.onresize = resize;
請記住沒有任何溢出設置的“最大高度”=“無響應”站點。
選項 1 - 左列比右列短:
將右列高度設置為: max-height: 100px;
.parent { display: flex; } #child_1{ border: 1px solid lightgray; } #child_2 { overflow-y: scroll; max-height: 100px; border: 1px solid violet; }
<main class='parent'> <div id="child_1"> <p> Very short content </p> </div> <div id="child_2"> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> </div> </main>
選項 2 - 左列比右列長:
在這種情況下,一種選擇是為父級設置max-height
(非常非常無響應的方法 - 因為您應該為兩個列聲明溢出)+非常奇怪的 UI:
.parent { display: flex; max-height: 100px; } #child_1{ border: 3px solid orange; overflow-y: scroll; } #child_2 { overflow-y: scroll; border: 1px solid violet; }
<main class='parent'> <div id="child_1"> <p> Tall div </p> <p> Tall div </p> <p> Tall div </p> <p> Tall div </p> <p> Tall div </p> </div> <div id="child_2"> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> <p> This div has a lot of content. The height must follow the div next to me. </p> </div> </main>
一個簡單的解決方案是向父元素添加height
或max-height
。 這樣兩個孩子在任何寬度上都有相同的高度。
// HTML
<div id='container' class='parent'>
<div id='content' class='child'>
The height must be determined by this div.
</div>
<div class='child'>
This div has a lot of content.
The height must follow the div next to me.
</div>
</div>
// CSS
.parent {
display: flex;
max-height: 70px; // remove if using JavaScript
}
.child {
overflow: scroll;
}
或者,您可以使用 JavaScript 來確定第一個子元素的當前高度,並將其設為父元素的高度。
添加:
// JS
const container = document.getElementById('container');
const content = document.getElementById('content');
const updateContainer = () => {
let contentHeight = content.clientHeight + 'px';
container.style.height = contentHeight;
}
updateContainer();
這只是一個例子。 您需要使用事件偵聽器來觸發該函數。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.