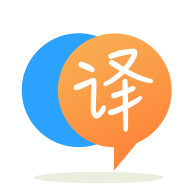
[英]java.lang.AssertionError: Status expected:<200> but was:<400>
[英]I'm getting a java.lang.AssertionError: Status expected:<200> but was:<401> when trying to do unit tests
測試方法
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.http.MediaType;
import org.springframework.test.context.junit.jupiter.SpringExtension;
import org.springframework.test.web.servlet.MockMvc;
import java.util.Optional;
import static org.mockito.Mockito.*;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.post;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
@ExtendWith(SpringExtension.class)
@WebMvcTest(value = RoleController.class)
class RoleControllerTest {
@MockBean
RoleRepoService roleRepoService;
@Autowired
private MockMvc mvc;
private ObjectMapper mapper = new ObjectMapper();
//Error I keep getting
//java.lang.AssertionError: Status expected:<200> but was:<401>
@Test
void addRole_thenReturnsRole_andStatus200() throws Exception{
Role manager = new Role(1,"Administrator", "Administrative Privileges");
when(roleRepoService.saveRole(manager)).thenReturn(manager);
this.mvc.perform(post("/addRole")
.contentType(MediaType.APPLICATION_JSON)
.characterEncoding("UTF-8")
.content(mapper.writeValueAsString(manager)))
.andExpect(status().isOk())
.andExpect(jsonPath("$.roleID").value("1"))
.andExpect(jsonPath("$.roleType").value("Administrator"))
.andExpect(jsonPath("$.role_description ").value("Administrative Privileges"));
verify(roleRepoService, times(1)).saveRole(manager);
}
//Error I keep getting
//java.lang.AssertionError: Status expected:<200> but was:<401>
@Test
void getRoleByID_andReturnsRole__andStatus200() throws Exception {
Role manager = new Role(1,"Administrator", "Administrative Privileges");
when(roleRepoService.selectRoleById(1)).thenReturn(Optional.of(manager));
this.mvc.perform(get("/getRole/1")
.characterEncoding("UTF-8"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON))
.andExpect(jsonPath("$.roleID").value("1"))
.andExpect(jsonPath("$.roleType").value("Administrator"))
.andExpect(jsonPath("$.role_description ").value("Administrative Privileges"));
verify(roleRepoService, times(1)).selectRoleById(1);
}
我的角色POJO
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.persistence.*;
import javax.validation.constraints.NotBlank;
@Entity
public class Role {
@GeneratedValue
@Id
@Column(insertable = false, name = "roleID")
private int roleID;
@NotBlank
@Column(name = "roleType")
private String roleType;
@NotBlank
@Column(name = "role_description")
private String role_description;
public Role(@NotBlank @JsonProperty String roleType, @NotBlank @JsonProperty String role_description) {
this.roleType = roleType;
this.role_description = role_description;
}
public Role(@JsonProperty int roleID, @NotBlank @JsonProperty String roleType, @NotBlank @JsonProperty String role_description) {
this.roleID = roleID;
this.roleType = roleType;
this.role_description = role_description;
}
//useful for serializing
public Role() {
}
public int getRoleID() {
return roleID;
}
public void setRoleID(int roleID) {
this.roleID = roleID;
}
public String getRoleType() {
return roleType;
}
public String getRole_description() {
return role_description;
}
}
我的角色控制器
import com.cellulant.absalife.models.Role;
import com.cellulant.absalife.service.role.RoleRepoService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping("api/v1/role")
public class RoleController {
public RoleRepoService roleRepoService;
@Autowired
public RoleController(RoleRepoService roleRepoService) {
this.roleRepoService = roleRepoService;
}
//adds Role to database
@PostMapping(path = "/addRole", produces = ("application/json"))
@ResponseBody
public Role addRole(@Valid @RequestBody @NotNull Role role){
return roleRepoService.saveRole(role);
}
//gets single Role based on id
@GetMapping(path = "/getRole/{id}")
@ResponseBody
public Optional<Role> getRoleByID(@Valid @NotNull @PathVariable("id") int id){
return roleRepoService.selectRoleById(id);
}
//this role should have the id in the body, otherwise it'll add a new record
@PutMapping(path = "/updateRole")
public Role updateRole (@Valid @NotNull @RequestBody Role role){
return roleRepoService.saveRole(role);
}
//deletes single Role based on ID
@DeleteMapping(path = "/deleteRole/{id}")
public void deleteRoleByID(@Valid @NotNull @PathVariable("id") int id){ roleRepoService.deleteRoleByID(id);
}
//gets all Role in database
@GetMapping(path ="/getAll" )
@ResponseBody
public List<Role> getAllRoles(){ return roleRepoService.getAll(); }
//deletes all Role in database
@DeleteMapping(path = "/deleteAll")
@ResponseBody
public void deleteAllRoles (){roleRepoService.deleteAllRoles();}
}
我的網絡安全配置器
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class WebSecurityConfigurer extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers().permitAll()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
我的pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.cellulant</groupId>
<artifactId>absalife</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>absalife</name>
<description>Absa Life USSD Implementation</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-jpa</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
測試運行失敗后的堆棧跟蹤
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.2.5.RELEASE)
2020-03-26 11:54:40.514 WARN 4201 --- [ main] o.s.boot.StartupInfoLogger : InetAddress.getLocalHost().getHostName() took 5004 milliseconds to respond. Please verify your network configuration (macOS machines may need to add entries to /etc/hosts).
2020-03-26 11:54:45.524 INFO 4201 --- [ main] c.c.a.controllers.RoleControllerTest : Starting RoleControllerTest on Angelas-MacBook-Pro.local with PID 4201 (started by angelamutua in /Users/angelamutua/Desktop/absalife)
2020-03-26 11:54:45.526 INFO 4201 --- [ main] c.c.a.controllers.RoleControllerTest : No active profile set, falling back to default profiles: default
2020-03-26 11:54:47.216 INFO 4201 --- [ main] o.s.s.concurrent.ThreadPoolTaskExecutor : Initializing ExecutorService 'applicationTaskExecutor'
2020-03-26 11:54:47.542 INFO 4201 --- [ main] .s.s.UserDetailsServiceAutoConfiguration :
Using generated security password: 3c0525c4-c927-48c6-b77f-0263118021f0
2020-03-26 11:54:47.689 INFO 4201 --- [ main] o.s.s.web.DefaultSecurityFilterChain : Creating filter chain: any request, [org.springframework.security.web.context.request.async.WebAsyncManagerIntegrationFilter@53e76c11, org.springframework.security.web.context.SecurityContextPersistenceFilter@1697f2b3, org.springframework.security.web.header.HeaderWriterFilter@221a2068, org.springframework.security.web.authentication.logout.LogoutFilter@109f8c7e, org.springframework.security.web.authentication.www.BasicAuthenticationFilter@72503b19, org.springframework.security.web.savedrequest.RequestCacheAwareFilter@3134153d, org.springframework.security.web.servletapi.SecurityContextHolderAwareRequestFilter@659feb22, org.springframework.security.web.authentication.AnonymousAuthenticationFilter@42cc183e, org.springframework.security.web.session.SessionManagementFilter@1192b58e, org.springframework.security.web.access.ExceptionTranslationFilter@793d163b, org.springframework.security.web.access.intercept.FilterSecurityInterceptor@49cb1baf]
2020-03-26 11:54:47.727 INFO 4201 --- [ main] o.s.b.t.m.w.SpringBootMockServletContext : Initializing Spring TestDispatcherServlet ''
2020-03-26 11:54:47.727 INFO 4201 --- [ main] o.s.t.web.servlet.TestDispatcherServlet : Initializing Servlet ''
2020-03-26 11:54:47.741 INFO 4201 --- [ main] o.s.t.web.servlet.TestDispatcherServlet : Completed initialization in 14 ms
2020-03-26 11:54:47.777 INFO 4201 --- [ main] c.c.a.controllers.RoleControllerTest : Started RoleControllerTest in 17.571 seconds (JVM running for 18.831)
MockHttpServletRequest:
HTTP Method = POST
Request URI = /addRole
Parameters = {}
Headers = [Content-Type:"application/json;charset=UTF-8", Content-Length:"86"]
Body = {"roleID":1,"roleType":"Administrator","role_description":"Administrative Privileges"}
Session Attrs = {SPRING_SECURITY_SAVED_REQUEST=DefaultSavedRequest[http://localhost/addRole]}
Handler:
Type = null
Async:
Async started = false
Async result = null
Resolved Exception:
Type = null
ModelAndView:
View name = null
View = null
Model = null
FlashMap:
Attributes = null
MockHttpServletResponse:
Status = 401
Error message = Unauthorized
Headers = [WWW-Authenticate:"Basic realm="Realm"", X-Content-Type-Options:"nosniff", X-XSS-Protection:"1; mode=block", Cache-Control:"no-cache, no-store, max-age=0, must-revalidate", Pragma:"no-cache", Expires:"0", X-Frame-Options:"DENY"]
Content type = null
Body =
Forwarded URL = null
Redirected URL = null
Cookies = []
java.lang.AssertionError: Status expected:<200> but was:<401>
Expected :200
Actual :401
<Click to see difference>
at org.springframework.test.util.AssertionErrors.fail(AssertionErrors.java:59)
at org.springframework.test.util.AssertionErrors.assertEquals(AssertionErrors.java:122)
at org.springframework.test.web.servlet.result.StatusResultMatchers.lambda$matcher$9(StatusResultMatchers.java:627)
at org.springframework.test.web.servlet.MockMvc$1.andExpect(MockMvc.java:196)
at com.cellulant.absalife.controllers.RoleControllerTest.addRole_thenReturnsRole_andStatus200(RoleControllerTest.java:51)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at org.junit.platform.commons.util.ReflectionUtils.invokeMethod(ReflectionUtils.java:675)
at org.junit.jupiter.engine.execution.MethodInvocation.proceed(MethodInvocation.java:60)
at org.junit.jupiter.engine.execution.InvocationInterceptorChain$ValidatingInvocation.proceed(InvocationInterceptorChain.java:125)
at org.junit.jupiter.engine.extension.TimeoutExtension.intercept(TimeoutExtension.java:132)
at org.junit.jupiter.engine.extension.TimeoutExtension.interceptTestableMethod(TimeoutExtension.java:124)
at org.junit.jupiter.engine.extension.TimeoutExtension.interceptTestMethod(TimeoutExtension.java:74)
at org.junit.jupiter.engine.execution.ExecutableInvoker$ReflectiveInterceptorCall.lambda$ofVoidMethod$0(ExecutableInvoker.java:115)
at org.junit.jupiter.engine.execution.ExecutableInvoker.lambda$invoke$0(ExecutableInvoker.java:105)
at org.junit.jupiter.engine.execution.InvocationInterceptorChain$InterceptedInvocation.proceed(InvocationInterceptorChain.java:104)
at org.junit.jupiter.engine.execution.InvocationInterceptorChain.proceed(InvocationInterceptorChain.java:62)
at org.junit.jupiter.engine.execution.InvocationInterceptorChain.chainAndInvoke(InvocationInterceptorChain.java:43)
at org.junit.jupiter.engine.execution.InvocationInterceptorChain.invoke(InvocationInterceptorChain.java:35)
at org.junit.jupiter.engine.execution.ExecutableInvoker.invoke(ExecutableInvoker.java:104)
at org.junit.jupiter.engine.execution.ExecutableInvoker.invoke(ExecutableInvoker.java:98)
at org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.lambda$invokeTestMethod$6(TestMethodTestDescriptor.java:202)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.invokeTestMethod(TestMethodTestDescriptor.java:198)
at org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.execute(TestMethodTestDescriptor.java:135)
at org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.execute(TestMethodTestDescriptor.java:69)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$5(NodeTestTask.java:135)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$7(NodeTestTask.java:125)
at org.junit.platform.engine.support.hierarchical.Node.around(Node.java:135)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$8(NodeTestTask.java:123)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.executeRecursively(NodeTestTask.java:122)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.execute(NodeTestTask.java:80)
at java.util.ArrayList.forEach(ArrayList.java:1257)
at org.junit.platform.engine.support.hierarchical.SameThreadHierarchicalTestExecutorService.invokeAll(SameThreadHierarchicalTestExecutorService.java:38)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$5(NodeTestTask.java:139)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$7(NodeTestTask.java:125)
at org.junit.platform.engine.support.hierarchical.Node.around(Node.java:135)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$8(NodeTestTask.java:123)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.executeRecursively(NodeTestTask.java:122)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.execute(NodeTestTask.java:80)
at java.util.ArrayList.forEach(ArrayList.java:1257)
at org.junit.platform.engine.support.hierarchical.SameThreadHierarchicalTestExecutorService.invokeAll(SameThreadHierarchicalTestExecutorService.java:38)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$5(NodeTestTask.java:139)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$7(NodeTestTask.java:125)
at org.junit.platform.engine.support.hierarchical.Node.around(Node.java:135)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$8(NodeTestTask.java:123)
at org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.executeRecursively(NodeTestTask.java:122)
at org.junit.platform.engine.support.hierarchical.NodeTestTask.execute(NodeTestTask.java:80)
at org.junit.platform.engine.support.hierarchical.SameThreadHierarchicalTestExecutorService.submit(SameThreadHierarchicalTestExecutorService.java:32)
at org.junit.platform.engine.support.hierarchical.HierarchicalTestExecutor.execute(HierarchicalTestExecutor.java:57)
at org.junit.platform.engine.support.hierarchical.HierarchicalTestEngine.execute(HierarchicalTestEngine.java:51)
at org.junit.platform.launcher.core.DefaultLauncher.execute(DefaultLauncher.java:229)
at org.junit.platform.launcher.core.DefaultLauncher.lambda$execute$6(DefaultLauncher.java:197)
at org.junit.platform.launcher.core.DefaultLauncher.withInterceptedStreams(DefaultLauncher.java:211)
at org.junit.platform.launcher.core.DefaultLauncher.execute(DefaultLauncher.java:191)
at org.junit.platform.launcher.core.DefaultLauncher.execute(DefaultLauncher.java:128)
at com.intellij.junit5.JUnit5IdeaTestRunner.startRunnerWithArgs(JUnit5IdeaTestRunner.java:69)
at com.intellij.rt.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:33)
at com.intellij.rt.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:230)
at com.intellij.rt.junit.JUnitStarter.main(JUnitStarter.java:58)
2020-03-26 11:54:47.992 INFO 4201 --- [extShutdownHook] o.s.s.concurrent.ThreadPoolTaskExecutor : Shutting down ExecutorService 'applicationTaskExecutor'
Process finished with exit code 255
我一直試圖解決這個問題一天了。 我曾嘗試在我的測試類中禁用 SecurityConfiguration 類,但這導致 ObjectPostProcessor bean 類型出錯。 對代碼轉儲表示歉意,但我不確定如何繼續。 任何幫助將不勝感激!
您嘗試在方法addRole_thenReturnsRole_andStatus200()
測試的資源的 URL 似乎是錯誤的。
請嘗試將其更改為api/v1/role/addRole
而不是僅/addRole
。
您也可以考慮先嘗試使用 Postman 或 Soap UI 等工具。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.