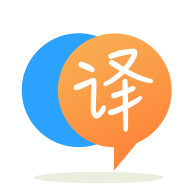
[英]JavaScript - Count and remove duplicates from array of objects ES6
[英]remove and count duplicates from an groupedBy array in Javascript
我有一個數組,它應該以某種方式進行修改,以便刪除和計算每個房間的重復燈。 目前我有以下數組:
我想讓它們按room
分組。 我lodash
使用了lodash
函數:
groupByAndCount(lamps) {
const groupedArray = groupBy(lamps, function(n) {
return n.pivot.room;
});
return groupedArray;
},
基本上每個分組的Object
看起來都與第一個數組中的對象相同。 但現在我想使用我寫的函數來計算和刪除重復項:
removeAndCountDuplicates(lamps) {
// map to keep track of element
// key : the properties of lamp (e.g name, fitting)
// value : obj
let map = new Map();
// loop through each object in Order.
lamps.forEach(data => {
// loop through each properties in data.
let currKey = JSON.stringify(data.name);
let currValue = map.get(currKey);
// if key exists, increment counter.
if (currValue) {
currValue.count += 1;
map.set(currKey, currValue);
} else {
// otherwise, set new key with in new object.
let newObj = {
id: data.id,
name: data.name,
fitting: data.fitting,
light_color_code: data.light_color_code,
dimmability: data.dimmability,
shape: data.shape,
price: data.price,
watt: data.watt,
lumen: data.lumen,
type: data.type,
article_number: data.article_number,
count: 1,
image: data.image
// room: data.pivot.room
};
map.set(currKey, newObj);
}
});
// Make an array from map.
const res = Array.from(map).map(e => e[1]);
return res;
},
結果應該是一個像第一個數組一樣的數組。 但應該包含這個:
所以對象應該是這樣的:
id
name:
fitting:
light_color_code:
dimmability:
shape:
price:
watt:
lumen:
type:
article_number:
room:
count:
但是我無法讓這些函數一起工作,所以它返回這個數組。 任何人都可以在正確的方向上幫助我嗎? 提前致謝,任何幫助表示贊賞。 感覺就像我快到了。
一些示例數據:
{
"id": 3,
"name": "Noxion Lucent LED Spot PAR16 GU10 4W 827 36D | Extra Warm Wit - Vervangt 50W",
"fitting": "GU10",
"light_color_code": "2700K - 827 - Zeer warm wit",
"dimmability": 0,
"shape": "Spot",
"price": 2.44,
"watt": 4,
"lumen": 370,
"type": "LED ",
"article_number": 234987,
"pivot": {
"order_id": 2,
"lamp_id": 3,
"room": "Garage"
},
"image": {
"id": 3,
"lamp_id": 3,
"name": "234987",
"path": "/storage/234987.jpg"
}
}
由於在 group by 中,您正在創建一個以鍵和數組作為值的對象,因此您必須首先迭代該對象(您可以使用Object.entries()
),然后是數組。
嘗試將分組對象傳遞給下面的函數,您應該得到具有正確計數的數組。 這是一個可以玩的示例 - https://stackblitz.com/edit/js-d2xcn3
注意:我使用了pivot.lamp_id
(並將其添加到對象中)用作地圖的鍵。 您可以選擇將其更改為其他一些獨特的屬性。
const removeDuplicates = (lamps) => {
let map = new Map();
Object.entries(lamps).forEach(([key, value])=> {
// loop through each object in Order.
lamps[key].forEach(data => {
// loop through each properties in data.
let currKey = JSON.stringify(data.pivot.lamp_id);
let currValue = map.get(currKey);
// if key exists, increment counter.
if (currValue) {
currValue.count += 1;
map.set(currKey, currValue);
} else {
// otherwise, set new key with in new object.
let newObj = {
lamp_id: data.pivot.lamp_id,
id: data.id,
name: data.name,
fitting: data.fitting,
light_color_code: data.light_color_code,
dimmability: data.dimmability,
shape: data.shape,
price: data.price,
watt: data.watt,
lumen: data.lumen,
type: data.type,
article_number: data.article_number,
count: 1,
image: data.image
// room: data.pivot.room
};
map.set(currKey, newObj);
}
});
})
// Make an array from map.
const res = Array.from(map).map(e => e[1]);
return res;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.