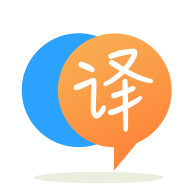
[英]How to dynamically loop through an arraylist and add each nth element
[英]How to compare each element in my arraylist to find the nth smallest of the two arrays?
import java.util.*;
public class Main {
public static void main(String[] args) {
// this section of code will require user input to have the value of n to be set
System.out.println(("What number would you like to set n equal to ?"));
Scanner sc = new Scanner(System.in);
System.out.print(("n= "));
int value = sc.nextInt();
System.out.println((""));
// this section of code set the two array only to hold the value of n
Random rand = new Random();
ArrayList<Integer> setA = new ArrayList<Integer>();
for (int i = 0; i < value; i++) {
int picks = rand.nextInt(1000);
setA.add(picks);
}
Collections.sort(setA);
System.out.println(setA);
ArrayList<Integer> setX = new ArrayList<Integer>();
for (int k = 0; k < value; k++) {
int picks = rand.nextInt(1000);
setX.add(picks);
}
Collections.sort(setX);
System.out.println(setX);
solution(setA,setX,value);
}
private static int solution(ArrayList<Integer> A1, ArrayList<Integer> X1, int value) {
// This section of code is where the arrays will be compared to find the nth smallest.
ArrayList<Integer> setF = new ArrayList<Integer>();
for (int c = 0; c < A1.size(); c++) {
for(int k = 0; k < X1.size(); k++) {
if(A1.get(c) < X1.get(k)) {
}
}
}
System.out.print(setF);
return value;
}
}
到目前為止,我已將程序設置為讓用戶輸入一個將用於數組大小的數字。 輸入數字后,arrays 將使用將按順序放置的隨機數創建。 接下來我想通過我的 arrays 的每個元素 go 並比較看看哪些數字可以放在我的最終數組中。 在我的最終數組中,將返回第 n 個最小的數字。 我無法將兩個數組合並在一起。
例如,如果下面的 n = 10 是我的兩個 arrays
[124、264、349、450、487、641、676、792、845、935]
B [2, 159, 241, 323, 372, 379, 383, 475, 646, 836]
124 < 2 這個語句是錯誤的,所以 2 將被添加到我的最終數組列表中。 數組 B 應該移動到列表中的下一個元素。
124 < 159 這是真的,所以 124 被添加到我的最終數組列表中。 數組 A 應該移動到列表中的下一個元素。 264 < 159 這個陳述是錯誤的所以 159。
最終數組[2,124, 159,...]
n 最小的是383
。
希望這個例子能給你一個我想要完成的理想。如果你有更好的東西請告訴我..
您的解決方案可以工作,但您可以使解決方案的時間復雜度為 o(n) 而不是 o(n^2)。
您可以做的是,由於 arrays 的排序相同,您可以比較 position 為零的兩個元素(就像您正在做的那樣),然后無論哪個元素更小,從數組中彈出該元素並將其添加到最終數組中。 繼續測試零(第)索引元素,直到 arrays 之一為空。 一旦它為空,您可以只 append 剩余的其他數組放在最終數組的末尾,這應該可以實現您想要的。
所以在一些java代碼實現中:
private ArrayList<Integer> sortTwoArrays(ArrayList<Integer> arrayA, ArrayList<Integer> arrayB) {
ArrayList<Integer> finalArray = new ArrayList<>();
while(!arrayA.isEmpty() && !arrayB.isEmpty()) {
if (arrayA.get(0) < arrayB.get(0)) {
// remove element and store
finalArray.add(arrayA.remove(0));
}
else {
finalArray.add(arrayB.remove(0));
}
}
// Find out which array is not empty
// Adds remaining contents of non-empty array to end of finalArray
if (!arrayA.isEmpty()) {
finalArray.addAll(arrayA);
}
else if (!arrayB.isEmpty()) {
finalArray.addAll(arrayB);
}
return finalArray;
}
要獲得第 n 個最小值,只需將用戶作為參數傳入的值添加到 function,然后當您返回 function 時,只需返回finalArray.get(nthIndex)
示例代碼展示在這里。
注意:原來的兩個 ArrayLists 會被這個方法銷毀
如果要保留兩個 arrays,我建議跟蹤變量內部列表中的兩個索引,然后根據一個項目小於另一個項目的時間遞增。 此外,將whie-loop之后的If語句檢查從isEmpty()檢查更改為像indexOfArrayA == arrayA.size() - 1這樣的比較。
無論如何,我希望這會有所幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.