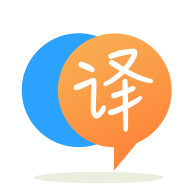
[英]React Native: Can i add items into flatlist that is in another screen,
[英]Add items to FlatList dynamically in React Native
我有一個包含兩個項目的 FlatList。 我需要 append 這個列表與另一個元素。 當用戶單擊按鈕時,來自文本輸入的數據應出現在 FlatList 的末尾。 因此,我嘗試將數據 object 推送到列表數組的末尾,但新項目替換了最后一個。
import React, { useState } from 'react';
import { Text, View, StyleSheet, Button } from 'react-native';
import { FlatList } from 'react-native-gesture-handler';
export default function HomeScreen() {
var initialElements = [
{ id : "0", text : "Object 1"},
{ id : "1", text : "Object 2"},
]
const [exampleState, setExampleState] = useState(initialElements);
const [idx, incr] = useState(2);
const addElement = () => {
var newArray = [...initialElements , {id : toString(idx), text: "Object " + (idx+1) }];
initialElements.push({id : toString(idx), text: "Object " + (idx+1) });
incr(idx + 1);
setExampleState(newArray);
}
return (
<View style={styles.container}>
<FlatList
keyExtractor = {item => item.id}
data={exampleState}
renderItem = {item => (<Text>{item.item.text}</Text>)} />
<Button
title="Add element"
onPress={addElement} />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
width: '100%',
borderWidth: 1
},
});
import React, { useState } from 'react'; import { Text, View, StyleSheet, Button } from 'react-native'; import { FlatList } from 'react-native-gesture-handler'; export default function HomeScreen() { var initialElements = [ { id: "0", text: "Object 1"}, { id: "1", text: "Object 2"}, ] const [exampleState, setExampleState] = useState(initialElements) const addElement = () => { var newArray = [...initialElements, {id: "2", text: "Object 3"}]; setExampleState(newArray); } return ( <View style={styles.container}> <FlatList keyExtractor = {item => item.id} data={exampleState} renderItem = {item => (<Text>{item.item.text}</Text>)} /> <Button title="Add element" onPress={addElement} /> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', width: '100%', borderWidth: 1 }, });
您只是在更改 listElements 數組。 這不會觸發組件的重新渲染,因此平面列表將保持不變。
在組件中創建一個state變量並將您的數據存儲在其中,以便任何修改都會導致重新渲染。
我通過將數組更改為 state 變量來解決替換元素的問題。
import React, { useState } from 'react';
import { Text, View, StyleSheet, Button } from 'react-native';
import { FlatList } from 'react-native-gesture-handler';
export default function HomeScreen() {
const [initialElements, changeEl] = useState([
{ id : "0", text : "Object 1"},
{ id : "1", text : "Object 2"},
]);
const [exampleState, setExampleState] = useState(initialElements);
const [idx, incr] = useState(2);
const addElement = () => {
var newArray = [...initialElements , {id : idx, text: "Object " + (idx+1) }];
incr(idx + 1);
console.log(initialElements.length);
setExampleState(newArray);
changeEl(newArray);
}
return (
<View style={styles.container}>
<FlatList
keyExtractor = {item => item.id}
data={exampleState}
renderItem = {item => (<Text>{item.item.text}</Text>)} />
<Button
title="Add element"
onPress={addElement} />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
width: '100%',
borderWidth: 1
},
});
我通過在導出 function 之外定義數組來修復它
import React, { useState } from 'react'
import { StyleSheet, View, TextInput, TouchableOpacity, Text, FlatList } from 'react-native'
let tipArray = [
{key: '1', tip: 20},
{key: '2', tip: 12}
]
const screen = function tipInputScreen( {navigation} ) {
const [ tip, setTip ] = useState('')
const addTip = ()=>{
if(tip == "")return
tipArray.push({key: (tipArray.length + 1).toString(), tip})
setTip('')
}
const logInput = (input)=>{
setTip(input)
}
const renderTip = ({ item }) => {
return(
<TouchableOpacity style={styles.listItem}>
<Text style={styles.buttonText}>{`${item.tip} $`}</Text>
</TouchableOpacity>)
}
return (
<View
style={styles.background}>
<TextInput
style={styles.input}
keyboardType={'number-pad'}
keyboardAppearance={'dark'}
onChangeText={logInput}
value={tip}
/>
<TouchableOpacity
style={styles.redButton}
onPress={addTip}>
<Text style={styles.buttonText}>Add Tip</Text>
</TouchableOpacity>
<FlatList
data={tipArray}
renderItem={renderTip}
style={styles.flatList}
/>
</View>
)
}
const styles = StyleSheet.create({
background: {
backgroundColor: 'grey',
paddingTop: Platform.OS === "android" ? 25:0,
width: '100%',
height: '100%',
alignItems: 'center'
},
input: {
marginTop:40,
color:'white',
fontSize:30,
backgroundColor: "#2e2a2a",
height: 50,
width: '90%',
textDecorationColor: "white",
borderColor: 'black',
borderWidth: 2
},
flatList:{
width: "100%"
},
listItem: {
width: "90%",
height: 50,
backgroundColor: "#2e2e2e",
borderRadius: 25,
marginVertical: 4,
marginHorizontal: "5%",
justifyContent: "center"
},
listItemTitle: {
color: "white",
textAlign: "center",
fontSize: 18
},
redButton: {
justifyContent: "center",
width: "90%",
height: 50,
backgroundColor: "red",
borderRadius: 25,
marginHorizontal: 20,
marginVertical: 10
},
buttonText: {
color: "white",
textAlign: "center",
fontSize: 18
}
})
export default screen;
這是一個更大的應用程序的一部分,但它應該可以解決問題,我希望它有所幫助
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.