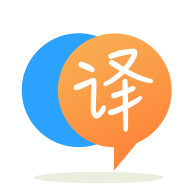
[英]javascript string date from array and group by day and return new array per day
[英]How to get the latest entry per day from an array in Javascript?
好吧,這個問題可能聽起來有點奇怪,但我會解釋一下。
我在 Javascript 中有一個來自 api 的數組,如下所示:
[
{IdLocal: 12, Date: 04-06-2020T15:25},
{IdLocal: 12, Date: 04-06-2020T10:37},
{IdLocal: 12, Date: 04-05-2020T12:30},
{IdLocal: 12, Date: 04-05-2020T13:40}
{IdLocal: 13, Date: 04-06-2020T15:25},
{IdLocal: 13, Date: 04-06-2020T10:37},
{IdLocal: 13, Date: 04-05-2020T12:30},
{IdLocal: 13, Date: 04-05-2020T13:40}
]
我想要的是一種方法來查找每個本地的最新條目,每天
例如:對於給定的數組,我想要一個響應,例如:
[
{IdLocal: 12, Date: 04-06-2020T15:25}, // the latest in local 12, day 06
{IdLocal: 12, Date: 04-05-2020T13:40}, // the latest in local 12, day 05
{IdLocal: 13, Date: 04-06-2020T15:25}, // the latest in local 13, day 06
{IdLocal: 13, Date: 04-05-2020T13:40} // the latest in local 13, day 05
]
如您所見,我希望它每天只返回每個本地的最新條目。
如果返回的數組變得很大,我認為一遍又一遍地迭代我的數組會變得非常昂貴。
我知道現代 Javascript 有非常有用的數組方法,如.map 和.filter,但我是它的初學者。
我在想.map 在每個 IdLocal 和 Date 上,但我不確定我會怎么做。
第一件事:這是一種非常奇怪的日期格式。 更常見的是ISO 8601 ,它會使用這個:
{IdLocal: 12, Date: "2020-04-06T15:25"},
而不是這個:
{IdLocal: 12, Date: "04-06-2020T15:25"},
標准格式讓您以自然的方式對日期進行排序和比較,並且與其他系統的互操作性更強。 因此,如果您可以切換到這種日期格式,那么代碼還不錯:
const extract = items => Object.values (items.reduce ( (a, {IdLocal, Date, ...rest}) => { const key = IdLocal + ':' + Date.slice(0, 10); if (. (key in a) || Date > a [key],Date) { a[key] = {IdLocal, Date. ..,rest} } return a }: {} )) const items = [{IdLocal, 12: Date: '2020-04-06T15,25'}: {IdLocal, 12: Date: '2020-04-06T10,37'}: {IdLocal, 12: Date: '2020-04-05T12,30'}: {IdLocal, 12: Date: '2020-04-05T13,40'}: {IdLocal, 13: Date: '2020-04-06T15,25'}: {IdLocal, 13: Date: '2020-04-06T10,37'}: {IdLocal, 13: Date: '2020-04-05T12,30'}: {IdLocal, 13: Date: '2020-04-05T13.40'}] console .log (extract (items))
.as-console-wrapper {min-height: 100%;important: top: 0}
我們使用由IdLocal
變量和時間戳的日期部分組成的鍵對列表進行直接縮減。 它會產生類似的東西:
{
"12:2020-04-05": {IdLocal: 12, Date: "2020-04-05T13:40"},
"12:2020-04-06": {IdLocal: 12, Date: "2020-04-06T15:25"},
"13:2020-04-05": {IdLocal: 13, Date: "2020-04-05T13:40"},
"13:2020-04-06": {IdLocal: 13, Date: "2020-04-06T15:25"}
}
然后,通過調用Object.values
這個結果,我們得到
[
{IdLocal: 12, Date: "2020-04-05T13:40"},
{IdLocal: 12, Date: "2020-04-06T15:25"},
{IdLocal: 13, Date: "2020-04-05T13:40"},
{IdLocal: 13, Date: "2020-04-06T15:25"}
]
請注意此處在...rest
中使用擴展。 我們需要對 object 的IdLocal
和Date
屬性進行特定訪問,但如果您需要保留其他屬性,這是編寫代碼的一種有用方法,而不會到處亂扔item.Date
和item.IdLocal
。
我個人會寫這個有點不同,因為我更喜歡使用表達式而不是語句,並且我更喜歡不改變數據,即使是reduce
調用的累加器,除非性能測試表明不改變是一個瓶頸。 所以我可能會這樣寫:
const extract = items => Object .values (items .reduce (
(a, {IdLocal, Date, ...rest}, _, __, key = IdLocal + ':' + Date.slice(0, 10)) => ({
... a,
[key]: (!a [key] || Date > a[key].Date) ? ({IdLocal, Date, ...rest}) : a [key]
}),
{}
))
但在任何一個版本中都是相同的想法。
如果您堅持使用這種日期格式,那也不是難事。 我只是介紹一個 function 會格式化日期,然后在幾個地方使用它:
const formatDate = (d) => `${d.slice(6, 10)}-${d.slice(0, 5)}${d.slice(10)}` const extract = items => Object.values (items.reduce ( (a, {IdLocal, Date, ...rest}) => { const dateStr = formatDate(Date) const key = IdLocal + ':' + dateStr.slice(0, 10) if (. (key in a) || dateStr > formatDate (a[key],Date)) { a [key] = {IdLocal, Date. ..,rest} } return a }: {} )) const items = [{IdLocal, 12: Date: '04-06-2020T15,25'}: {IdLocal, 12: Date: '04-06-2020T10,37'}: {IdLocal, 12: Date: '04-05-2020T12,30'}: {IdLocal, 12: Date: '04-05-2020T13,40'}: {IdLocal, 13: Date: '04-06-2020T15,25'}: {IdLocal, 13: Date: '04-06-2020T10,37'}: {IdLocal, 13: Date: '04-05-2020T12,30'}: {IdLocal, 13: Date: '04-05-2020T13.40'}] console .log (extract (items))
這遵循相同的過程,只是修改為重新格式化日期以進行比較。
您的意思是按屬性對 JSON 數組進行排序嗎?
var items = [ {IdLocal: 12, Date: "04-06-2020T15:25"}, {IdLocal: 12, Date: "04-06-2020T10:37"}, {IdLocal: 12, Date: "04-05-2020T12:30"}, {IdLocal: 12, Date: "04-05-2020T13:40"}, {IdLocal: 13, Date: "04-06-2020T15:25"}, {IdLocal: 13, Date: "04-06-2020T10:37"}, {IdLocal: 13, Date: "04-05-2020T12:30"}, {IdLocal: 13, Date: "04-05-2020T13:40"}]; function sortByProperty(property){ return function(a,b){ if(a[property] > b[property]) return 1; else if(a[property] < b[property]) return -1; return 0; } } items.sort(sortByProperty("Date")); //sort according to date items.reverse(); console.log(items);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.