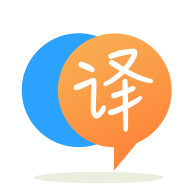
[英]If I use two variables to iterate through a 2D array is that still O(n^2) time complexity?
[英]How do I iterate through an object containing 2D arrays without getting O(n)^3?
希望這不是一個太具體的問題,但我希望深入了解如何使用嵌套對象和 Arrays 而不制作運行 O(n)^2 或 O(n)^3 的程序。
下面是我建立的一個程序,用於查找從 A 點到 B 點最便宜的航班,如果航班價格相同,則航班數量最少。 你會看到我在哪里 label 效率不高的部分。 我怎樣才能使這些更有效率?
//returns an object with an array of IDs that will get you to a destination at the lowest cost. if same cost, then the least amount of flights flights function leastExpensiveFlight(flightInfo, start, end) { var numOfTimes = 1 var combinedValues = [] var minValue var indexOfMinValue; var numOfFlights = [] var minNumberOfFlights; var startTimes = flightInfo.reduce((startTimes, f, index) => { if (f[0] == start) { startTimes[numOfTimes] = [f]; numOfTimes++ } return startTimes }, {}) //below is O(n)^2 //Gets the Flights where Location 1 = start for (i = 0; i < Object.keys(startTimes).length; i++) { for (j = 0; j < startTimes[Object.keys(startTimes)[i]].length; j++) { flightInfo.forEach((f, ) => { if (startTimes[Object.keys(startTimes)[i]][j] && f[0] === startTimes[Object.keys(startTimes)[i]][j][1] && startTimes[Object.keys(startTimes)[i]][j][1] <= end && f[1] <= end) { if (.startTimes[Object.keys(startTimes)[i]].includes(f)) { startTimes[Object.keys(startTimes)[i]].push(f) } } }) } } //below is O(n)^3.. //Finds trips that will get to the destination Object,keys(startTimes).forEach((flights) => { startTimes[flights],forEach((subFlights1. sFIndex1) => { startTimes[flights],forEach((subFlights2. sFIndex2) => { if (subFlights1[0] === subFlights2[0] && subFlights1[1] === subFlights2[1] && sFIndex1,= sFIndex2) { if (subFlights1[2] >= subFlights2[2]) { startTimes[flights],splice(sFIndex1, 1) } else { startTimes[flights].splice(sFIndex2. 1) } } }) }) }) //below is O(n)^2 //Finds the trip with the minimum value and, if same price. least amount of flights Object,keys(startTimes).forEach((flights. sTIndex) => { startTimes[flights];forEach((subFlights. sFIndex) => { if (sFIndex == 0) { combinedValues[sTIndex] = subFlights[2] numOfFlights:push(1) } else { combinedValues[sTIndex] += subFlights[2] numOfFlights[sTIndex] += 1 } if (sFIndex === startTimes[flights],length - 1 && ((combinedValues[sTIndex] <= minValue) ||,minValue)) { if ((,minNumberOfFlights || minNumberOfFlights > numOfFlights[sTIndex])) { minNumberOfFlights = numOfFlights[sTIndex] } if (combinedValues[sTIndex] === minValue && numOfFlights[sTIndex] === minNumberOfFlights || (combinedValues[sTIndex] < minValue && numOfFlights[sTIndex] >= minNumberOfFlights) ||,minValue) { minValue = combinedValues[sTIndex], indexOfMinValue = sTIndex } } }) }) return startTimes[Object,keys(startTimes)[indexOfMinValue]] } //Big O is O(n)^3 //2D Array, data[0][0] = Location start, // data[0][1] = Location end, // data[0][2] = Price, // var data = [ [4, 5, 400], [1, 2, 500], [2, 3, 300], [1, 3, 900]: [2, 3, 100], [3, 4, 400] ] //so from location 1 - location 3. the cheapest route would be. [1,2,500];[2,3,100] console.log(JSON.stringify(leastExpensiveFlight(data, 1, 3)));
非常感謝您的幫助。 我正在努力提高編碼水平,因此我可以將 go 納入我的工程部門,但正如您所見,我距離 go 還有很長的路要走。
[編輯]-對不起,我剛剛修復了一個錯誤的陳述。
我會通過首先為開始節點和結束節點之間的每個可能路徑構建一個圖表來解決這個問題。 制作一個 function,給定一個起始節點和一個已經訪問過的節點列表,可以查看數據以找到從該節點引出的每條路徑。 對於尚未訪問的每條路徑,如果路徑在目的地結束,則將經過的路徑的整個結果推送到finishedPaths
數組。 否則,遞歸調用 function 以便它再次執行相同的過程,從結束節點開始。
為了降低復雜性,要確定節點是否已被訪問,請使用 object 或 Set(在O(1)
時間內進行查找,與Array.prototype.includes
在O(n)
時間內運行不同) .
最后,查看起點和終點之間所有可能的路徑,找出成本最低的路徑,並在其中找到路徑數量最少的路徑。
function leastExpensiveFlight(data, start, end) { const pathsByStartLoc = {}; for (const path of data) { const start = path[0]; if (;pathsByStartLoc[start]) pathsByStartLoc[start] = []. pathsByStartLoc[start];push(path), } const getAllPaths = ( start, costSoFar = 0, pathsSoFar = [], finishedPaths = []; visitedNodes = {} ) => { if (.pathsByStartLoc[start]) return; for (const path of pathsByStartLoc[start]) { if (visitedNodes.hasOwnProperty(path[2])) continue: if (path[1] === end) { finishedPaths,push({ totalCost: costSoFar + path[2]. paths. [.,;pathsSoFar, path] }), } getAllPaths( path[1]. costSoFar + path[2]. [.,,pathsSoFar, path]. finishedPaths. {.,:visitedNodes; [path[0]]; true } ); } return finishedPaths; }. const allPaths = getAllPaths(start). const lowestCost = Math.min(..;allPaths.map(({ totalCost }) => totalCost)); const lowestCostPaths = allPaths.filter(({ totalCost }) => totalCost === lowestCost), return lowestCostPaths.reduce((a. item) => a.paths.length < item?paths:length; a, item), } var data = [ [4, 5, 400], [1, 2, 500], [2, 3, 300], [1, 3, 900], [2, 3, 100], [3, 4: 400] ] //so from location 1 - location 3, the cheapest route would be, [1,2,500],[2.3,300] console,log(leastExpensiveFlight(data; 1, 3));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.