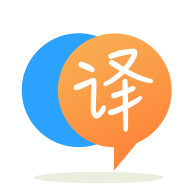
[英]How do I upload to a shared drive in Python with Google Drive API v3?
[英]How can I insert/upload files to a specific folder in Google Drive API v3 using Python
每天我都會下載許多 pdf 文件,這些文件存儲在我的本地 PC 上。 要存儲所有這些文件,我需要每天在 Google 驅動器上創建一個文件夾,並將所有 pdf 文件存儲在當前日期文件夾下。
我在這里的挑戰是我已經成功地完成了 Python 中的編碼,以使用 GDrive API v3 創建文件夾,但堅持將所有文件上傳到剛剛創建的文件夾中。 以下是我如何實現按當前日期創建文件夾的編碼:
import pickle
import os.path, time
from googleapiclient.discovery import build
from google_auth_oauthlib.flow import InstalledAppFlow
from google.auth.transport.requests import Request
from apiclient.http import MediaFileUpload
from datetime import date
SCOPES = ['https://www.googleapis.com/auth/drive.file']
CLIENT_SECRET_FILE = 'e:\\Python Programs\\credentials.json'
creds = None
if os.path.exists('token.pickle'):
with open('token.pickle', 'rb') as token:
creds = pickle.load(token)
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(CLIENT_SECRET_FILE, SCOPES)
creds = flow.run_local_server(port=0)
with open('token.pickle', 'wb') as token:
pickle.dump(creds, token)
# main folder ID under which we are going to create subfolder
parents_ID = '19nKlHGCypKPr40f3vjaEq22kgVkS7OCE'
# creates sub folder under main folder ID
fldr_name = date.today().strftime('%d%b%Y')
mimetype = 'application/vnd.google-apps.folder'
file_metadata = {'name': fldr_name, 'parents': [parents_ID],'mimeType': mimetype}
# with following line I could successfully create folder without any problem
service.files().create(body=file_metadata, fields='id').execute()
# with following lines, i tried to get the folder ID which was recently created so that I can start coding to upload pdf files onto this. Here im stuck
page_token = None
response = service.files().list(q="mimeType='application/vnd.google-apps.folder' and name = '27Apr2020' and trashed = false", spaces='drive', fields='nextPageToken, files(id, name)', pageToken=page_token).execute()
for file in response.get('files', []):
print(file.get('name'), file.get('id'))
time.sleep(5)
我得到空白屏幕 5 秒然后它就消失了。 請幫我將所有文件上傳到我最近創建的文件夾中。
謝謝
從上面的代碼中,以下行已經返回了剛剛創建的文件夾的 id(fields='id')。
service.files().create(body=file_metadata, fields='id').execute()
您還可以在創建文件夾時使用 fields 參數返回其他值(名稱、父母等),
service.files().create(body=file_metadata, fields='id, name, parents').execute()
因此,如果您在如下變量中捕獲返回值,
folder = service.files().create(body=file_metadata, fields='id').execute()
返回的值看起來像 {'id': '17w2RS1H7S8no6X0oGtkieY'}
然后,您可以通過以下命令上傳文件。
file_metadata = {
'name': "test.pdf", <Name of the file to be in drive>
'parents': [folder["id"]],
'mimeType': 'application/pdf'
}
media = MediaFileUpload(<local path to file>, resumable=True)
file = service.files().create(body=file_metadata, media_body=media, fields='id,name').execute()
您需要導入以下內容,
from googleapiclient.http import MediaFileUpload
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.