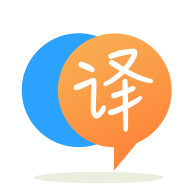
[英]Python: How to access the dictionary that has specific key-values in a list
[英]How to append one python dictionary to another while preserving their key-values as it is?
如何將 append 一個 python 字典轉換為另一個字典,如果兩者中的不同鍵具有重疊值,則數據不會被修改而是按原樣保留? 這也必須在 for 循環中完成,每次迭代都會返回一個新字典。 所以這個想法是創建一個包含循環返回的所有字典的最終主字典。
就像是:
finaldict = dict()
for - some loop condition:
dict1 = function-call()
finaldict = finaldict + dict1
access 'finaldict' outside the loop. 'finaldict' should now contain all the returned 'dict1's.
需要連接 2 個字典的方式如下:
finaldict = {22: [7, 9, 8]}
dict1 = {23: [7, 9, 8, 6, 12]}
期望的結果是:
finaldict = {22: [7, 9, 8], 23: [7, 9, 8, 6, 12]}
如何在 python 中以最快的方式實現這一點?
您可以使用更新 function:
In [21]: finaldict = {22: [7, 9, 8]}
...: dict1 = {23: [7, 9, 8, 6, 12]}
In [22]:
In [22]: finaldict.update(dict1)
In [23]: finaldict
Out[23]: {22: [7, 9, 8], 23: [7, 9, 8, 6, 12]}
In [24]:
如果您看到列表值發生變化,則很可能您的 function 始終返回相同的list
object,而不是在不同時間返回單獨的列表。 即使您將列表保留在要合並的字典之外,您也會發現舊列表中的值與您預期的不同。
您可以使用以下代碼看到這種行為:
_lst = [] # this is an implementation detail of the function below
def foo():
"""returns a steadily longer list of "foo" strings"""
_lst.append('foo')
return _lst
print(foo()) # prints ['foo']
print(foo()) # prints ['foo', 'foo'], all as expected so far
x = foo()
print(x) # prints ['foo', 'foo', 'foo'], fine
y = foo()
print(y) # prints ['foo', 'foo', 'foo', foo'], fine here too
print(x) # prints ['foo', 'foo', 'foo', foo'], unexpectedly changed!
當再次調用foo
來分配y
時,您可能會驚訝x
的值是如何變化的。 但這只是在您沒有意識到x
和y
都引用同一個列表 object _lst
的情況下(如果您不知道foo
function 是如何實現的,您可能不知道這一點)。
在您的代碼中,您的 function 以與foo
相同的方式返回相同的列表(盡管它周圍有一個字典,所以它不太明顯)。 如果您控制 function,您也許可以對其進行更改,以便每次都返回一個新列表。 在我的示例中,這可能如下所示:
def foo():
_lst.append('foo')
return list(_lst) # return a shallow copy of the list!
您的代碼可能會更復雜一些,但可能不會太多。 只需確保為您返回的每本詞典創建一個新列表即可。
另一方面,如果您無法修改 function(可能是因為它是庫的一部分或其他東西),您可以在 go 合並字典時自己制作副本。
finaldict = dict()
while some_condition():
dict1 = function_call()
finaldict.update((key, list(value)) for key, value in dict1.items())
我們傳遞給dict.update
的生成器表達式產生key, value
元組,但值是我們從function_call
獲得的字典中列表的淺表副本。
帶有前綴運算符**
的簡單解決方案(Python3.5),用於按鍵值(PEP 448)解包字典。
>>> {**dict1, **finaldict}
{23: [7, 9, 8, 6, 12], 22: [7, 9, 8]}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.