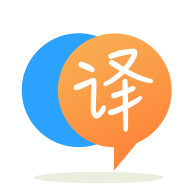
[英]After getting all documents in collection can't add field to ArrayList, Android Studio, Java, Firebase Firestore
[英]How to access Firebase Firestore collection by more than one registered users in android studio app using java?
我面臨的問題是:當用戶 2 嘗試訪問同一個數據庫集合時,而不是顯示數據庫中已經存在某些數據(要求是用戶 1 輸入的數據保存在同一個數據庫中),我得到數據庫中的 null。 我還可以弄清楚為什么會發生這種情況,但對如何以不同用戶在 BookingInformation 集合中保存文檔字段“StartTime”和“EndTimes”的唯一值的方式查詢 firestore 集合卻一無所知?
以下是我使用的代碼;
FirebaseAuth fAuth = FirebaseAuth.getInstance();
String userId = fAuth.getCurrentUser().getUid();
Log.d(TAG,"userID: "+userId);
FirebaseFirestore fstore = FirebaseFirestore.getInstance();
DocumentReference docRef =
fstore.collection("BookingInformation").document(userId);
之所以發生這種情況,是因為我使用 userId 作為“BookingInformation”集合的文檔 ID。 通過這樣做,每次新用戶登錄時都會創建一個新文檔。 我應該如何解決它以確保當用戶 2 輸入數據時,它首先檢查集合中是否存在某些文檔?
添加代碼
NewBookingFragment.java
newBookingViewModel.getBookingInformation().observe(getViewLifecycleOwner(), new Observer<BookingInfo>() {
@Override
public void onChanged(final BookingInfo bookingInfo) {
Log.d(TAG,"hi from bookingInfodatabase");
Log.d(TAG,"content of bookingInfodatabase"+bookingInfo);
if (bookingInfo == null) {
Log.d(TAG,"no data from database, so add as a new");
Toast toast = Toast.makeText(getActivity().getApplicationContext(), "No data from database, so add as a new booking information", Toast.LENGTH_SHORT);
toast.show();
searchBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast toast1 = Toast.makeText(getActivity().getApplicationContext(), "No data in database to compare!", Toast.LENGTH_SHORT);
toast1.show();
}
});
addBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.d(TAG, "user id in fragment class: "+currentUserId);
final Map<String, Object> reserveInfo = new HashMap<>();
reserveInfo.put("Date",date.getText().toString());
reserveInfo.put("StartTime",startTime.getText().toString());
reserveInfo.put("EndTime",endTime.getText().toString());
reserveInfo.put("UserId",currentUserId);
dataStore.collection("BookingInformation").document(currentUserId).set(reserveInfo)
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
Toast toast4 = Toast.makeText(getActivity().getApplicationContext(), "Area reserved successfully", Toast.LENGTH_SHORT);
toast4.show();
}
})
.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
Toast toast5 = Toast.makeText(getActivity().getApplicationContext(), "Reservation failed", Toast.LENGTH_SHORT);
toast5.show();
}
});
}
});
}else{
searchBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.d(TAG,"after clicking available button");
Log.d(TAG,"booking id"+bookingInfo.getBookingDocId());
Log.d(TAG,"database time: "+bookingInfo.getStartTime());
Log.d(TAG,"user input time: "+startTime.getText().toString());
Log.d(TAG,"comparing time: "+bookingInfo.getStartTime().equals(startTime.getText().toString()));
if (bookingInfo.getDate().equals(date.getText().toString()) ){
Log.d(TAG, "Date check ");
if (bookingInfo.getStartTime().equals(startTime.getText().toString()) && bookingInfo.getEndTime().equals(endTime.getText().toString())) {
Toast toast = Toast.makeText(getActivity().getApplicationContext(), "Select another time.", Toast.LENGTH_SHORT);
toast.show();
Log.d(TAG, "start and end time check ");
if (bookingInfo.getStartTime().equals(startTime.getText().toString())) {
Log.d(TAG, "start time check ");
if (bookingInfo.getEndTime().equals(endTime.getText().toString())) {
Toast toast2 = Toast.makeText(getActivity().getApplicationContext(), "Select another end time.", Toast.LENGTH_SHORT);
toast2.show();
Log.d(TAG, "end time check ");
}
Log.d(TAG, "end time not equal check ");
}
Log.d(TAG, "start time not equal check ");
}
else{
Toast toast4 = Toast.makeText(getActivity().getApplicationContext(), "Area available at this time,'Book' it.", Toast.LENGTH_SHORT);
toast4.show();
availabletextView.setText("Area available");
addBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.d(TAG, "user id in fragment class: "+currentUserId);
final Map<String, Object> reserveInfo = new HashMap<>();
reserveInfo.put("Date",date.getText().toString());
reserveInfo.put("StartTime",startTime.getText().toString());
reserveInfo.put("EndTime",endTime.getText().toString());
reserveInfo.put("UserId",currentUserId);
dataStore.collection("BookingInformation").document(currentUserId).set(reserveInfo)
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
Toast toast4 = Toast.makeText(getActivity().getApplicationContext(), "Area reserved successfully", Toast.LENGTH_SHORT);
toast4.show();
}
})
.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
Toast toast5 = Toast.makeText(getActivity().getApplicationContext(), "Reservation failed", Toast.LENGTH_SHORT);
toast5.show();
}
});
}
});
}
} else {
availabletextView.setText("Area available");
Log.d(TAG, "user id: "+currentUserId);
addBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.d(TAG, "user id in fragment class: "+currentUserId);
final Map<String, Object> reserveInfo = new HashMap<>();
reserveInfo.put("Date",date.getText().toString());
reserveInfo.put("StartTime",startTime.getText().toString());
reserveInfo.put("EndTime",endTime.getText().toString());
reserveInfo.put("UserId",currentUserId);
dataStore.collection("BookingInformation").document(currentUserId).set(reserveInfo)
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
Toast toast4 = Toast.makeText(getActivity().getApplicationContext(), "Area reserved successfully", Toast.LENGTH_SHORT);
toast4.show();
}
})
.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
Toast toast5 = Toast.makeText(getActivity().getApplicationContext(), "Reservation failed", Toast.LENGTH_SHORT);
toast5.show();
}
});
}
});
}
}
});
}
}
});
NewBookingViewModel.java
public class NewBookingViewModel extends ViewModel {
private static final String TAG = "Firelog";
public LiveData<BookingInfo> getBookingInformation() {
final MutableLiveData<BookingInfo> bookingInfoMutableLiveData = new MutableLiveData<>();
FirebaseAuth fAuth = FirebaseAuth.getInstance();
String userId = fAuth.getCurrentUser().getUid();
Log.d(TAG,"userID: "+userId);
FirebaseFirestore fstore = FirebaseFirestore.getInstance();
DocumentReference docRef = fstore.collection("BookingInformation").document(userId);
Log.d(TAG,"docRef"+docRef);
String docid = docRef.getId();
Log.d(TAG,"document id"+docid);
docRef.get().addOnCompleteListener(new OnCompleteListener<DocumentSnapshot>() {
@Override
public void onComplete(@NonNull Task<DocumentSnapshot> task) {
if (task.isSuccessful()) {
Log.d(TAG,"hi from viewmodel database");
DocumentSnapshot documentSnapshot = task.getResult();
Log.d(TAG, "Doc ID: "+documentSnapshot.getId());
Log.d(TAG,"document exist: "+documentSnapshot.exists());
if (documentSnapshot != null && documentSnapshot.exists()) {
String date = documentSnapshot.getString("Date");
String startTime = documentSnapshot.getString("StartTime");
String endTime = documentSnapshot.getString("EndTime");
BookingInfo bookingInfo = documentSnapshot.toObject(BookingInfo.class);
Log.d(TAG,"hi from viewmodel date: "+date);
bookingInfo.setDate(date);
bookingInfo.setStartTime(startTime);
bookingInfo.setEndTime(endTime);
bookingInfoMutableLiveData.postValue(bookingInfo);
} else {
bookingInfoMutableLiveData.postValue(null);
}
} else {
bookingInfoMutableLiveData.postValue(null);
}
}
});
return bookingInfoMutableLiveData;
}
}
謝謝。
我剛剛發現要允許 2 個或更多用戶在集合中輸入和保存數據而沒有任何重復數據 w.r.t startTime 和 endTime 字段,我必須確保文檔已經存在或不存在。 為此,作為解決方案,我計算了集合中的文檔。
以下是代碼“NewBookingViewModel.java”
fstore.collection("BookingInformation")
.get()
.addOnCompleteListener(new OnCompleteListener<QuerySnapshot>() {
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task) {
if (task.isSuccessful()) {
QuerySnapshot querySnapshot = task.getResult();
if (!querySnapshot.isEmpty()) {
for (QueryDocumentSnapshot queryDocumentSnapshot : task.getResult()) {
count++;
Log.d(TAG, "document count: "+count);
if (count != 0) {
String date = queryDocumentSnapshot.getString("Date");
String startTime = queryDocumentSnapshot.getString("StartTime");
String endTime = queryDocumentSnapshot.getString("EndTime");
BookingInfo bookingInfo = queryDocumentSnapshot.toObject(BookingInfo.class);
bookingInfo.setBookingDocId((queryDocumentSnapshot.getId()));
bookingInfo.setDate(date);
bookingInfo.setStartTime(startTime);
bookingInfo.setEndTime(endTime);
bookingInfoMutableLiveData.postValue(bookingInfo);
}else {
Log.d(TAG, "No booking data");
}
}
} else {
bookingInfoMutableLiveData.postValue(null);
}
} else {
bookingInfoMutableLiveData.postValue(null);
}
}
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.