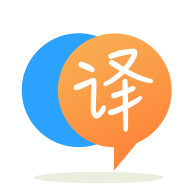
[英]Spring Data Rest - How to prevent update of specific fields through PATCH?
[英]Update Specific Fields with Spring Data Rest and MongoDB
I'm using Spring Data MongoDB and Spring Data Rest to create a REST API which allows GET, POST, PUT and DELETE operations on my MongoDB database and it's all working fine except for the update operations (PUT). 只有當我在請求正文中發送完整的 object 時它才有效。
例如,我有以下實體:
@Document
public class User {
@Id
private String id;
private String email;
private String lastName;
private String firstName;
private String password;
...
}
要更新 lastName 字段,我必須發送所有用戶 object,包括密碼! 這顯然是非常錯誤的。 如果我只發送該字段進行更新,則所有其他字段在我的數據庫中都設置為 null。 我什至嘗試在這些字段上添加@NotNull 約束,現在更新甚至不會發生,除非我發送所有用戶對象的字段。
我嘗試在這里搜索解決方案,但只找到以下帖子但沒有解決方案: 如何使用 MongoRepository 接口更新 mongo db 中的特定字段?
有沒有辦法實現這個?
Spring Data Rest uses Spring Data repositories to automatically retrieve and manipulate persistent data using Rest calls (check out https://docs.spring.io/spring-data/rest/docs/current/reference/html/#reference ).
使用 Spring 數據 MongoDB 時,您擁有MongoOperations
接口,該接口用作 Rest 端點的存儲庫。 但是 MongoOperations 目前不支持特定字段更新!
PS:如果他們在 Spring 數據 JPA 中添加像 @DynamicUpdate 這樣的功能,那就太棒了
但這並不意味着可以做到,這是我遇到此問題時所做的解決方法。
首先讓我解釋一下我們要做什么:
注意:我們不想為應用程序中的每個 model 重新執行這些步驟,因此我們將檢索要動態更新的 model。 為此,我們將創建一個實用程序 class。 [這是可選的]
讓我們首先將 org.reflections api 添加到我們的項目依賴項中,這允許我們獲取所有具有特定注釋的類(在我們的例子中為@Document
):
<dependency>
<groupId>org.reflections</groupId>
<artifactId>reflections</artifactId>
<version>0.9.12</version>
</dependency>
然后創建一個名為UpdateUtility的新 class 並添加以下方法,並將MODEL_PACKAGE
屬性替換為您自己的包含您的實體的 package:
public class UpdateUtility {
private static final String MODEL_PACKAGE = "com.mycompany.myproject.models";
private static boolean initialized = false;
private static HashMap<String, Class> classContext = new HashMap<>();
private static void init() {
if(!initialized) {
Reflections reflections = new Reflections(MODEL_PACKAGE);
Set<Class<?>> classes = reflections.getTypesAnnotatedWith(Document.class); // Get all the classes annotated with @Document in the specified package
for(Class<?> model : classes) {
classContext.put(model.getSimpleName().toLowerCase(), model);
}
initialized = true;
}
}
public static Class getClassFromType(String type) throws Exception{
init();
if(classContext.containsKey(type)) {
return classContext.get(type);
}
else {
throw new Exception("Type " + type + " does not exists !");
}
}
}
使用此實用程序 class 我們可以檢索 model class 以更新其類型。 例如: UpdateUtility.getClassFromType()
將返回User.class
現在讓我們創建我們的 controller:
public class UpdateController {
@Autowired
private MongoTemplate mongoTemplate;
@PutMapping("/{type}/{id}")
public Object update(@RequestBody HashMap<String, Object> fields,
@PathVariable(name = "type") String type,
@PathVariable(name = "id") String id) {
try {
Class classType = UpdatorUtility.getClassFromType(type); // Get the domain class from the type in the request
Query query = new Query(Criteria.where("id").is(id)); // Update the document with the given ID
Update update = new Update();
// Iterate over the send fields and add them to the update object
Iterator iterator = fields.entrySet().iterator();
while(iterator.hasNext()) {
HashMap.Entry entry = (HashMap.Entry) iterator.next();
String key = (String) entry.getKey();
Object value = entry.getValue();
update.set(key, value);
}
mongoTemplate.updateFirst(query, update, classType); // Do the update
return mongoTemplate.findById(id, classType); // Return the updated document
} catch (Exception e) {
// Handle your exception
}
}
}
現在我們能夠在不更改調用的情況下更新指定的字段。 所以在你的情況下,電話是:
PUT http://MY-DOMAIN/user/MY-USER-ID { lastName: "My new last name" }
PS:您可以通過添加更新嵌套對象中的特定字段的可能性來改進它...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.