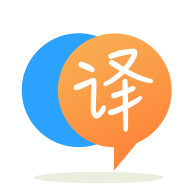
[英]logical multiplication of matrices A and B by the operation “AND” and “OR” in C
[英]logical multiplication of matrices 1 and 2 by the operation “AND” and “OR” in C
這是問題:
邏輯矩陣是其所有元素為 0 或 1 的矩陣。
我們通過下面定義的運算來定義矩陣 A 和 B 的邏輯乘法,其中“·”是邏輯與運算,“+”是邏輯或運算。
在此作業中,您將創建兩個 5x5 邏輯矩陣並找到將從“乘”這兩個矩陣創建的相應矩陣
定義全局 SIZE 等於 5(已在模板中定義)
編寫一個 function 獲取矩陣引用並從用戶那里讀取矩陣的輸入。 如果輸入非零,則將其替換為 1。如果用戶在行尾之前沒有輸入足夠的值,則矩陣中的剩余單元格將填充為零。 還要確保如果用戶輸入的字符過多,您只需要輸入所需的內容並丟棄剩余的輸入。 (例如:1509 是一個值為 1101 的 2x2 矩陣,'1 5' 也是一個值為 1111 的 2x2 矩陣,如上所述,突出顯示的空白被視為 1。)
Function 簽名:
void read_mat(int mat[][SIZE])
編寫一個 function,將兩個矩陣相乘,如上所述,並將結果輸入到具有合適維度的第三個矩陣中。
Function 簽名:
void mult_mat(int mat1[][SIZE],int mat2[][SIZE], int result_mat[][SIZE])
編寫一個 function 將矩陣打印到屏幕上。 請使用“%3d”作為打印格式,使其看起來更好看,如下圖所示。
Function 簽名:
void print_mat(int mat[][SIZE])
編寫使用上述功能的主程序。 程序從用戶那里讀取矩陣值,將它們相乘並在屏幕上打印結果矩陣。
給出的 function 定義是有意的,返回語句為 void。 不要改變它們。 Arrays 作為引用而不是像變量這樣的原語在函數之間傳輸。 所以 function 定義是完全有效的。 此外,對來自用戶的輸入沒有限制。 您可以只讀取所需的數字,然后停止讀取,並丟棄剩余的輸入。
這是我的代碼:
#include <stdio.h>
#define SIZE 5
void read_mat(int mat[][SIZE],int size)
{
int i = 0, j = 0, k = 0;
char c;
c=getchar();
while(c!='\n' && k<size*size){
if(c!='0'){
mat[i][j]=1;
j++;
}
else{
mat[i][j]=0;
j++;
}
if (j >= size){
j = 0;
i++;
}
if (i >= size){
return;
}
c=getchar();
k++;
}
}
void mult_mat(int mat1[][SIZE], int mat2[][SIZE], int result_mat[][SIZE])
{
int i,j,k;
for (i = 0; i <SIZE; ++i){
for (j = 0; j <SIZE; ++j)
{
result_mat[i][j] = 0;
for (k = 0; k < SIZE; ++k)
result_mat[i][j] += mat1[i][k] * mat2[k][j];
if(result_mat[i][j]!=0){
result_mat[i][j]=1;
}
}
}
}
void print_mat(int mat[][SIZE],int size)
{
int i, j;
for (i = 0; i < SIZE; i++) {
for (j = 0; j < SIZE; j++)
printf("%3d", mat[i][j]);
printf("\n");
}
//Please use the "%3d" format to print for uniformity.
}
int main()
{
int mat1[SIZE][SIZE]={ 0 }, mat2[SIZE][SIZE]={ 0 }, res_mat[SIZE][SIZE]={0};
printf("Please Enter Values For Matrix 1\n");
read_mat(mat1,SIZE);
printf("Please Enter Values For Matrix 2\n");
read_mat(mat2,SIZE);
mult_mat(mat1,mat2,res_mat);
printf("The First Matrix Is :- \n");
print_mat(mat1,SIZE);
printf("The Second Matrix Is :- \n");
print_mat(mat2,SIZE);
printf("The Resultant Matrix Is :- \n");
print_mat(res_mat,SIZE);
return 0;
}
輸入和 output 應該是這樣的:
Please Enter Values For Matrix 1
111000654987010
Please Enter Values For Matrix 2
11 53
The First Matrix Is :-
1 1 1 0 0
0 1 1 1 1
1 1 0 1 0
0 0 0 0 0
0 0 0 0 0
The Second Matrix Is :-
1 1 1 1 1
1 0 0 0 0
0 0 0 0 0
0 0 0 0 0
0 0 0 0 0
The Resultant Matrix Is :-
1 1 1 1 1
1 0 0 0 0
1 1 1 1 1
0 0 0 0 0
0 0 0 0 0
問題:
當我向 mat1 輸入一個大字符串時,它直接計算而不讓我向 mat2 輸入一個字符串,我該如何解決這個問題?
Please Enter Values For Matrix 1
6475465176547650654605836574657625846071654047
Please Enter Values For Matrix 2
The First Matrix Is :-
1 1 1 1 1
1 1 1 1 1
1 1 1 1 1
0 1 1 1 1
0 1 1 1 1
The Second Matrix Is :-
1 1 1 1 1
1 1 1 1 1
1 0 1 1 1
1 1 0 1 1
0 0 0 0 0
The Resultant Matrix Is :-
1 1 1 1 1
1 1 1 1 1
1 1 1 1 1
1 1 1 1 1
1 1 1 1 1
if (i >= size){
return;
}
c=getchar();
一旦您閱讀了足夠的字符,您就可以從 function 返回,而不會消耗額外的字符。 您需要更改邏輯以繼續調用getchar()
直到點擊'\n'
,但如果i >= size
則丟棄這些字符。
while(c!='\n'){
if(i < size){
if(c!='0'){
mat[i][j]=1;
j++;
}
else{
mat[i][j]=0;
j++;
}
if (j >= size){
j = 0;
i++;
}
}
c=getchar();
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.