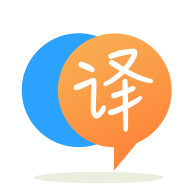
[英]Convert JSONObject to List<JSONObject> or a String to List<JSONObject>
[英]Convert string with special characters to JSONObject
我的輸入字符串為"{"notes_text": "someString"}"
。 someString 可以包含 \ 或 "。我需要創建一個 JSONObject 來表示這個字符串。
如果它包含特殊字符,只需使用將字符串作為輸入的構造函數將引發異常。
我已經嘗試了幾種方法來做到這一點
"{\"notes_text\":\"\"\"}"
或"{\"notes_text\":\"\\\"}"
。我需要將它們分別更改為"{\"notes_text\":\"\\\"\"}"
和"{\"notes_text\":\"\\\\\"}"
否則它會在轉換為 JSON Object 時再次拋出異常。 在字符串中使用 replace 方法將轉換輸入字符串中的所有 \"我嘗試使用正則表達式,但無法str.replaceAll("\\\".*\\\".*\\\"", replacemntString)
中的替換字符串
這是解決 json 損壞的初始問題的兩個類:
public class FixedJson {
private final String target;
private final Pattern pattern;
public FixedJson(String target) {
this(
target,
Pattern.compile("\"(.+?)\"[^\\w\"]")
);
}
public FixedJson(String target, Pattern pattern) {
this.target = target;
this.pattern = pattern;
}
public String value() {
return this.pattern.matcher(this.target).replaceAll(
matchResult -> {
StringBuilder sb = new StringBuilder();
sb.append(
matchResult.group(),
0,
matchResult.start(1) - matchResult.start(0)
);
sb.append(
new Escaped(
new Escaped(matchResult.group(1)).value()
).value()
);
sb.append(
matchResult.group().substring(
matchResult.group().length() - (matchResult.end(0) - matchResult.end(1))
)
);
return sb.toString();
}
);
}
}
public class Escaped {
private final String target;
private final Pattern pattern;
public Escaped(String target) {
this(
target,
Pattern.compile("[\\\\\"]")
);
}
public Escaped(String target, Pattern pattern) {
this.target = target;
this.pattern = pattern;
}
public String value() {
return this.pattern
.matcher(this.target)
.replaceAll("\\\\$0");
}
}
我已經編寫了單元測試來證明正確性:
import java.util.regex.Pattern;
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class FixedJsonTest {
@Test
public void normalValue() {
assertEquals(
"{\"notes_text\": \"someString\"}",
new FixedJson("{\"notes_text\": \"someString\"}").value()
);
}
@Test
public void valueWithOneDoubleQuotes() {
assertEquals(
"{\"notes_text\": \"\\\"\"}",
new FixedJson("{\"notes_text\": \"\"\"}").value()
);
}
@Test
public void valueWithOneDoubleQuotesAndAnotherValue() {
assertEquals(
"{\"notes_text\": \"\\\"\", \"hello\": \"world\"}",
new FixedJson("{\"notes_text\": \"\"\", \"hello\": \"world\"}").value()
);
}
@Test
public void valueWithTwoDoubleQuotes() {
assertEquals(
"{\"notes_text\": \"\\\"\\\"\"}",
new FixedJson("{\"notes_text\": \"\"\"\"}").value()
);
}
@Test
public void valueWithTwoDoubleQuotesAndAnotherValue() {
assertEquals(
"{\"notes_text\": \"\\\"\\\"\", \"hello\": \"world\"}",
new FixedJson("{\"notes_text\": \"\"\"\", \"hello\": \"world\"}").value()
);
}
@Test
public void valueWithOneSlash() {
assertEquals(
"{\"notes_text\": \"\\\\\"}",
new FixedJson("{\"notes_text\": \"\\\"}").value()
);
}
@Test
public void valueWithOneSlashAndAnotherValue() {
assertEquals(
"{\"notes_text\": \"\\\\\", \"hello\": \"world\"}",
new FixedJson("{\"notes_text\": \"\\\", \"hello\": \"world\"}").value()
);
}
@Test
public void valueWithTwoSlashes() {
assertEquals(
"{\"notes_text\": \"\\\\\\\\\"}",
new FixedJson("{\"notes_text\": \"\\\\\"}").value()
);
}
@Test
public void valueWithTwoSlashesAndAnotherValue() {
assertEquals(
"{\"notes_text\": \"\\\\\\\\\", \"hello\": \"world\"}",
new FixedJson("{\"notes_text\": \"\\\\\", \"hello\": \"world\"}").value()
);
}
}
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class EscapedTest {
@Test
public void doubleQuotesTest() {
assertEquals(
new Escaped("\"").value(),
"\\\""
);
assertEquals(
new Escaped("abc\"def").value(),
"abc\\\"def"
);
}
@Test
public void slashesTest() {
assertEquals(
new Escaped("\\").value(),
"\\\\"
);
assertEquals(
new Escaped("abc\\def").value(),
"abc\\\\def"
);
}
@Test
public void mixedTest() {
assertEquals(
new Escaped("\\\"").value(),
"\\\\\\\""
);
assertEquals(
new Escaped("abc\\\"def").value(),
"abc\\\\\\\"def"
);
}
}
import java.util.regex.Pattern;
public class FixedJsonExample {
public static void main(String[] args) {
String invalidJson = "{\"notes_text\":\"\"\"}";
final String fixedJson = new FixedJson(invalidJson).value();
System.out.println("fixedJson = " + fixedJson);
}
public static class FixedJson {
private final String target;
private final Pattern pattern;
public FixedJson(String target) {
this(
target,
Pattern.compile("\"(.+?)\"[^\\w\"]")
);
}
public FixedJson(String target, Pattern pattern) {
this.target = target;
this.pattern = pattern;
}
public String value() {
return this.pattern.matcher(this.target).replaceAll(
matchResult -> {
StringBuilder sb = new StringBuilder();
sb.append(
matchResult.group(),
0,
matchResult.start(1) - matchResult.start(0)
);
sb.append(
new Escaped(
new Escaped(matchResult.group(1)).value()
).value()
);
sb.append(
matchResult.group().substring(
matchResult.group().length() - (matchResult.end(0) - matchResult.end(1))
)
);
return sb.toString();
}
);
}
}
public static class Escaped {
private final String target;
private final Pattern pattern;
public Escaped(String target) {
this(
target,
Pattern.compile("[\\\\\"]")
);
}
public Escaped(String target, Pattern pattern) {
this.target = target;
this.pattern = pattern;
}
public String value() {
return this.pattern
.matcher(this.target)
.replaceAll("\\\\$0");
}
}
}
及其標准輸出:
fixedJson = {"notes_text":"\""}
此 JSON 可以使用以下工具進行驗證: https://jsonlint.com/
FixedJson
第一個 class FixedJson
使用正則表達式匹配 JSON 引理:雙引號之間的所有內容(包括放錯位置的雙引號)。
有關更多詳細信息,請參見此處的交互式示例: https://regexr.com/54blf
Escaped
class 第二個 class Escaped
使用正則表達式來匹配斜杠或雙引號。 他們的 escaping 需要它。
有關更多詳細信息,請參見此處的交互式示例: https://regexr.com/54bm1
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.