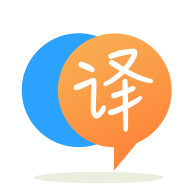
[英]Using python and boto3 for aws to describe-spot-fleet-instances?
[英]AWS Python boto3 describe all instances in all regions and output to CSV
我開始使用 boto3,我想知道如何獲取所有區域中具有自定義屬性的所有 ec2 實例的清單,並將其放入 CSV 文件。 對於單個區域,它看起來很簡單:
import boto3
import jmespath
import csv
client = boto3.client('ec2')
response = client.describe_instances()
myData = jmespath.search("Reservations[].Instances[].[NetworkInterfaces[0].OwnerId, InstanceId, InstanceType, State.Name, Placement.AvailabilityZone, PrivateIpAddress, PublicIpAddress, KeyName, [Tags[?Key=='Name'].Value] [0][0]]", response)
myFile = open('inventory.csv', 'w')
with myFile:
writer = csv.writer(myFile)
writer.writerows(myData)
但我無法弄清楚如何為所有地區實現類似的結果。 我試過這樣的事情:
all_regions = client.describe_regions()
RegionList = []
for r in all_regions['Regions']:
RegionList.append(r['RegionName'])
for r in RegionList:
client = boto3.client('ec2', region_name = r)
response = client.describe_instances()
myData = jmespath.search("Reservations[].Instances[].[NetworkInterfaces[0].OwnerId, InstanceId, InstanceType, State.Name, Placement.AvailabilityZone, PrivateIpAddress, PublicIpAddress, KeyName, [Tags[?Key=='Name'].Value] [0][0]]", response)
myFile = open('inventory.csv', 'w')
with myFile:
writer = csv.writer(myFile)
writer.writerows(myData)
但我得到的只是一個空列表。
這對我有用:
import csv
import jmespath
import boto3
import itertools
import configparser
import os
# Get list of profiles
config = configparser.ConfigParser()
path = os.path.join(os.path.expanduser('~'), '.aws/credentials')
config.read(path)
profiles = config.sections()
# Get list of regions
ec2_client = boto3.client('ec2')
regions = [region['RegionName']
for region in ec2_client.describe_regions()['Regions']]
# Get list of EC2 attributes from all profiles and regions
myData = []
for profile in profiles:
for region in regions:
current_session = boto3.Session(profile_name = profile, region_name = region)
client = current_session.client('ec2')
response = client.describe_instances()
output = jmespath.search("Reservations[].Instances[].[NetworkInterfaces[0].OwnerId, InstanceId, InstanceType, \
State.Name, Placement.AvailabilityZone, PrivateIpAddress, PublicIpAddress, KeyName, [Tags[?Key=='Name'].Value] [0][0]]", response)
myData.append(output)
# Write myData to CSV file with headers
output = list(itertools.chain(*myData))
with open("ec2-inventory-latest.csv", "w", newline="") as f:
writer = csv.writer(f)
writer.writerow(['AccountID','InstanceID','Type','State','AZ','PrivateIP','PublicIP','KeyPair','Name'])
writer.writerows(output)
它遍歷八個帳戶和所有區域,但大約需要五分鍾才能完成。 作為對比,在 bash 上只需要一分鍾。 有沒有辦法提高執行時間的速度?
嘗試這個...
import boto3
regions= [
#'ap-east-1',
'ap-northeast-1',
'ap-northeast-2',
'ap-south-1',
'ap-southeast-1',
'ap-southeast-2',
'ca-central-1',
'eu-central-1',
'eu-north-1',
'eu-west-1',
'eu-west-2',
'eu-west-3',
#'me-south-1',
'sa-east-1',
'us-east-1',
'us-east-2',
'us-west-1',
'us-west-2'
]
for region_name in regions:
print(f'region_name: {region_name}')
ec2= boto3.resource('ec2', region_name=region_name)
instances= ec2.meta.client.describe_instances()
for instance in instances['Reservations']:
print(instance)
希望能幫助到你
干杯
搖滾
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.