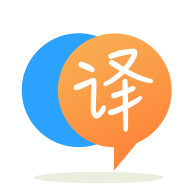
[英]How to merge two arrays of objects based on a object attribute OrderNo? - Javascript
[英]How to merge two nested objects in javascript based on the keys of the destination object?
我有兩個對象,一個可以被認為是超集,另一個可以被認為是子集。 保證子集具有與超集相似的結構,只是子集將缺少超集中的某些屬性。
var superset = {
car: {
type: 'SUV',
brand: 'Volvo',
color: 'Black'
},
features: {
interior: {
seats: 'leather',
color: 'black'
},
exterior: {
windows: 'tinted'
},
doors: 5
},
warranty: '5 yrs'
}
var subset = {
car: {
type: true,
brand: true
},
features: {
interior: {
seats: true
},
exterior: {
windows: true
},
doors: true
}
}
我想合並這兩個對象,使用超集中的值,但僅用於子集中存在的屬性。 結果應如下所示:
{
car: {
type: 'SUV',
brand: 'Volvo'
},
features: {
interior: {
seats: 'leather'
},
exterior: {
windows: 'tinted'
},
doors: 5
}
}
來自mergeWith
的 mergeWith 幾乎可以完成這項工作,但它似乎只適用於如下所示的第一級屬性。 想法是,一旦我為所有不需要的屬性分配了一個唯一值,我就可以循環並在以后擺脫它們。
_.mergeWith(subset, superset, function(a, b) {return a ? b : 'DELETE'})
Result:
{
car: {
type: 'SUV',
brand: 'Volvo',
color: 'Black'
},
features: {
interior: {
seats: 'leather',
color: 'black'
},
exterior: {
windows: 'tinted'
},
doors: 5
},
warranty: 'DELETE'
}
任何幫助表示贊賞。
這個解決方案只是遍歷subset
(我稱之為schema
)並從superset
(我稱之為input
)復制值(如果它們存在)。 如果兩個值都是 object,則 function 調用自身來處理嵌套屬性:
function merge(input, schema) { var result = {} for (var k in schema) { if (input[k] && typeof input[k] === 'object' && schema[k] && typeof schema[k] === 'object') { result[k] = merge(input[k], schema[k]) } else if (k in input) { result[k] = input[k] } } return result } var superset = { car: { type: 'SUV', brand: 'Volvo', color: 'Black' }, features: { interior: { seats: 'leather', color: 'black' }, exterior: { windows: 'tinted' }, doors: 5 }, warranty: '5 yrs' } var subset = { car: { type: true, brand: true }, features: { interior: { seats: true }, exterior: { windows: true }, doors: true } } var result = merge(superset, subset) console.log(result)
A simple recursive function that runs through the object with the wanted keys and if each exists in the data object (the superset) then either assigns the value to result if it is not an object, otherwise calls itself to do exactly the same for the subobject在那position。 如果鍵 object 格式錯誤,即如果它包含不在超集中任何位置的鍵,也可以改進它以返回錯誤。
var superset = {
car: {
type: 'SUV',
brand: 'Volvo',
color: 'Black'
},
features: {
interior: {
seats: 'leather',
color: 'black'
},
exterior: {
windows: 'tinted'
},
doors: 5
},
warranty: '5 yrs'
};
var subset = {
car: {
type: true,
brand: true
},
features: {
interior: {
seats: true
},
exterior: {
windows: true
},
doors: true
}
};
var merge = function(data, keys_obj, result)
{
for (key in keys_obj)
{
if (data.hasOwnProperty(key))
{
if (typeof(data[key]) == "object")
{
result[key] = {};
merge(data[key], keys_obj[key], result[key]);
}
else if (data[key])
result[key] = data[key];
}
//else you can throw an exception here: malformed subset
}
};
function test()
{
var result = {};
merge(superset, subset, result);
console.log(result);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.