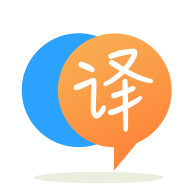
[英]how can i manipulate style of a html element in functional react component?
[英]How can I manipulate HTML elements with React and style them
我目前正在嘗試以下操作。
class MyComponent extends React.Component {
changeColor() {
document.getElementbyId("test").style.color = "green";
}
render() {
return (
<button id={MainStyles.test} onClick={this.changeColor} >
Hello World
</button>
);
}
}
export { MyComponent };
現在這適用於諸如window.alert("hello world")
之類的事件,但它不適用於上面的代碼,並最終產生此錯誤
“TypeError:無法讀取 null 的屬性‘樣式’”
我相信這是由於 document.getElementbyId 引起的, ref
解決此問題? 如果是這樣我會怎么 go 關於它?
Mainstyles.test 可能不會生成字符串“test”。 不看更多代碼是不可能知道的。 此外,還有一種更簡單的方法來引用。 對於傳遞到 onClick 屬性的每個 function,都有一個事件。 這個事件是一個 object,你可以這樣重構:
class MyComponent extends React.Component {
changeColor(event) {
event.target.style.color = "green";
}
render() {
return (
<button onClick={event => this.changeColor(event)} >
Hello World
</button>
);
}
}
export { MyComponent };
React 中有許多方法可以操作和設置 HTML 元素的樣式(通常不使用getElementbyId 、 querySelector或任何其他 Document 方法。)
正如卡斯帕在他的回答中提到的
每個傳入 onClick 屬性的 function 都有一個事件。
因此,您可以很容易地從點擊事件中獲取目標以訪問 HTML 元素。
import React from "react"
const MyComponent = () => {
const changeColor = e => {
e.target.style.color = "green"
}
return <button onClick={changeColor}>Hello World</button>
}
export { MyComponent }
也許您不想操縱按鈕本身,而是要操縱另一個 HTML 元素。 Refs 可能是實現此目的的一種方式,但React 文檔建議如果您可以以聲明方式進行,請避免這種情況。
import React, { useRef } from "react"
const MyComponent = () => {
const myRef = useRef()
const handleClick = () => {
myRef.current.style.color = "green"
}
return (
<div>
<button onClick={handleClick}>Hello World</button>
<p ref={myRef}>Hello world!</p>
</div>
)
}
export { MyComponent }
在某個階段,您的組件將達到需要使用 state 跟蹤事物的程度。
在這種情況下,我們傾向於不直接操作 HTML 元素,但允許 HTML 元素反映 state 中的值。
import React, { useState } from "react"
const MyComponent1 = () => {
const [colorIndex, setColorIndex] = useState(0)
const myColors = [
"fuchsia",
"cornflowerblue",
"firebrick",
"deepskyblue",
"MediumAquamarine",
"goldenrod",
"OliveDrab",
"darkmagenta",
"orangered",
]
const handleClick = () => {
setColorIndex(colorIndex >= myColors.length - 1 ? 0 : colorIndex + 1)
}
return (
<button style={{ color: myColors[colorIndex] }} onClick={handleClick}>
Hello World
</button>
)
}
export { MyComponent }
如果您還沒有,現在可能是查看 React 文檔的好時機,尤其是Thinking in React 。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.