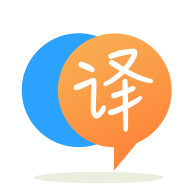
[英]How are CPython extensions able to call functions defined inside CPython?
[英]How are we able to call functions before they are defined in Python?
在 Python 函數式編程中,此代碼有效並按預期運行:
def increment_5(num):
number = 5
number += num
disp_result(num)
def disp_result(num):
print(f"5 was increased by {num}")
increment_5(6) # -> 5 was increased by 6
我在調用它后創建了disp_result()
function。 我在其他人的代碼中看到(我也以這種方式編寫),他們在定義函數后調用函數,我相信這是慣例和良好實踐。 但是,如圖所示,我們也可以在實際定義之前調用某個 function。 在 OOP 中,我可以在准確定義此方法之前使用self.certain_method()
調用 class 方法。 那么它是如何實現的,它是否僅在 Python 中發生?
我知道這個問題以前可能被問過。 如果是這樣,請知道我找不到它並且鏈接到它也會有幫助。
在定義之前,您不能調用 function。 在調用increment_5
之前不會調用disp_result
。
例如,這將失敗:
def increment_5(num):
number = 5
number += num
disp_result(num)
increment_5() # -> NameError: name 'disp_result' is not defined
def disp_result(num):
print(f"5 was increased by {num}")
雖然這會成功:
def increment_5(num):
number = 5
number += num
disp_result(num)
def disp_result(num):
print(f"5 was increased by {num}")
increment_5(4) # -> 5 was increased by 4
與類相反,如果沒有顯式調用 function,則不會執行 function 主體。 跟着:
>>> class A:
... print('hello')
...
hello
>>> def f():
... print('hello')
...
# <nothing happens>
Since Python does not execute the function body until you call that function, you can populate the scopes where that function might look for specific names after defining it.
像這樣的代碼有效的原因:
class Foo():
def method1(self):
self.method2()
def method2(self):
print("hello")
f = Foo()
f.method1()
是 Foo.method1 中的變量self.method2
僅在Foo.method1
中的代碼實際執行Foo.method1
被評估(並且它指向的 function 僅被調用),而不是在它被定義時。
Before class Foo
can be instantiated, the class definition must first have completed (ie the class object must have been created and it must have been populated with all of its methods). 因此,當實例f
存在並f.method1()
(或等效地, Foo.method1(f)
)時,實例method2
(通過其self
參數傳遞給方法)已經具有繼承自class,到那時已經完全填充。
因此,在 class 中聲明方法的順序無關緊要。
這與在定義之前嘗試調用 function 完全不同。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.