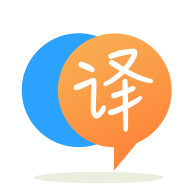
[英]Getting millisecond or microsecond-accurate boot time in Linux (C/C++)
[英]Elapsed time in my c++ application increases from 0 to 60 too fast (like in a millisecond, or a microsecond)
在我的交通模擬項目中,我想在一段時間內改變交通燈對象的狀態(燈的顏色)。 我編寫了 TrafficLightGroup class 將 7 個交通燈(T 燈)分成 3 組。 在這個 class 中,有一個模擬() function 將 t-light 添加到組並在一段時間內更改它們的狀態。 這里是 TrafficLightGroup class:
class TrafficLightGroup
{
TrafficLight* head, tail, greenLight;
float duration; //Period
public:
float time; //Current time elapsed in seconds
// duration: TrafficLight period
TrafficLightGroup(float duration): head(NULL), tail(NULL), greenLight(NULL), time(0){
this->duration = duration;
}
// light: Pointer to the traffic light object to be added to the group
void add(TrafficLight* light) {
if(head == NULL) {
head = light;
head->state = light->getState();
tail = head;
}
else {
tail->next = light;
tail = tail->next;
}
}
/* If the traffic light group timer reaches the switching period (i.e., duration member variable) of
the group, the next light in the group is turned into green while the others are turned into red
*/
void simulate(float timestep) { //timestep in seconds
this->duration = timestep;
this->time = timestep * 6;
TrafficLightGroup group1(timestep); //TTOP (road at t-top junction )
TrafficLightGroup group2(timestep); //TRIGHT (road at t-right junction )
TrafficLight* light1 = new TrafficLight(2*239 + 70, 250, 180, GREEN);
light1->setState(GREEN);
TrafficLight* light2 = new TrafficLight(2*239 + 240, 170, 90, RED);
light2->setState(RED);
group1.add(light1);
group2.add(light2);
//There are more t-lights but I don't include them
float i = 0;
while(i <= time) {
if(i == time - 5 * timestep) {
light1->setState(RED);
light2->setState(GREEN);
}
else if(i == time - 4 * timestep) {
light1->setState(GREEN);
light2->setState(RED);
}
else if(i == time - 3 * timestep) {
light1->setState(RED);
light2->setState(GREEN);
}
else if(i == time - 2 * timestep) {
light1->setState(GREEN);
light2->setState(RED);
}
else if(i == time - timestep) {
light1->setState(RED);
light2->setState(GREEN);
}
else if(i == time) {
light1->setState(GREEN);
light2->setState(RED);
}
i += 1;
}
}
};
如果您還想查看 TrafficLight class,我在此處添加:
typedef enum {
GREEN = 0,
RED = 1
}tLightState;
class TrafficLight {
float x, y;
float dir; //direction of the traffic light (determines the orientation of the traffic light on the map)
sf::Texture redTexture;
sf::Texture greenTexture;
sf::Sprite sprite;
public:
tLightState state; //current state of the light (either green or red). tLightState should be an enum
TrafficLight* next; //pointer to the next traffic light in the traffic light group
//x: x coordinate of the traffic light
//y: y coordinate of the traffic light
//dir: traffic light direction, i.e., orientation
//state: initial state of the traffic light
TrafficLight(float x, float y, float dir, tLightState state)
{
this->x=x; this->y=y; this->dir=dir;
if(state == GREEN){
greenTexture.loadFromFile("images/trafficlights/green.png");
sprite.setTexture(greenTexture);
}
else{
redTexture.loadFromFile("images/trafficlights/red.png");
sprite.setTexture(redTexture);
}
sprite.setPosition(sf::Vector2f(x,y));
sprite.setRotation(dir);
sprite.setOrigin(0, 0);
}
//Returns the position and the direction of the traffic light
//dir: orientation
void getPosition(float &x, float &y, float &dir) {x = this->x; y = this->y; dir = this->dir;}
//Draws the traffic lights
void draw(sf::RenderWindow *window) {window->draw(this->sprite);}
//Returns current traffic light state
tLightState getState() {return state;}
//Sets the traffic light state
void setState(tLightState state) {
this->state = state;
if(state == GREEN) {
greenTexture.loadFromFile("images/trafficlights/green.png");
sprite.setTexture(greenTexture);
}
else {
redTexture.loadFromFile("images/trafficlights/red.png");
sprite.setTexture(redTexture);
}
}
};
這是 main() function:
TrafficLight tlights[] = {
{2*239 + 70, 250, 180, GREEN}, {2*239 + 240, 170, 90, RED},
{4*239 + 70, 2*239 + 70, 180, RED}, {4*239 + 50, 2*239 + 170, 0, GREEN},
{2*239 + 40, 2*239 + 170, 90, GREEN}, {2*239 + 70, 2*239 + 70, 180, RED},
{2*239 + 200, 2*239 + 70, 270, RED}
};
TrafficLightGroup tlg1{10};
TrafficLightGroup tlg2{10};
TrafficLightGroup tlg3{10};
tlg1.add(new TrafficLight (2*239 + 70, 250, 180, GREEN));
tlg1.add(new TrafficLight (2*239 + 240, 170, 90, RED));
tlg2.add(new TrafficLight (4*239 + 70, 2*239 + 70, 180, RED));
tlg2.add(new TrafficLight (4*239 + 50, 2*239 + 170, 0, GREEN));
tlg3.add(new TrafficLight (2*239 + 40, 2*239 + 170, 90, GREEN));
tlg3.add(new TrafficLight (2*239 + 70, 2*239 + 70, 180, RED));
tlg3.add(new TrafficLight (2*239 + 200, 2*239 + 70, 270, RED));
tlg1.simulate(10); // these simulate calls are in *while (window.isOpen())* loop
tlg2.simulate(10);
tlg3.simulate(10);
我想模擬 10 秒周期的 t 燈。 在模擬() function 中,我在某個特定時間更改了 t 燈的狀態。 但是,當我運行應用程序時,我看不到這種變化,程序只是繪制了 t 燈。 出於這個原因,我在模擬() function 中添加了一個 std::cout 命令,以查看經過的時間如何增加。 然后,我看到經過的時間增加得太快了; 因此我看不到 t 燈狀態的變化。
我研究了 ctime header 以找到適合我的 function ,但我找不到任何適合這個問題的東西。
如何解決這個時間問題以正確模擬紅綠燈?
在 SFML 中有一個 sf::Clock class 可以解決您的確切問題。 您可以使用 restart() 啟動它,並以秒、毫秒或微秒為單位獲取經過的時間。 查看本教程以獲取更多信息。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.