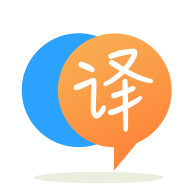
[英]How can I test React custom hook with RTL which uses react-query?
[英]How Can I test my custom Hook in React JS?
如何測試我的自定義 Hook? 謝謝您的幫助。
import { useEffect, useRef } from 'react';
export function useInterval(callback, delay) {
const savedCallback = useRef();
// Remember the latest callback.
useEffect(() => {
savedCallback.current = callback;
}, [callback]);
// Set up the interval.
useEffect(() => {
function tick() {
savedCallback.current();
}
if (delay !== null) {
const id = setInterval(tick, delay);
return () => {
clearInterval(id);
};
}
}, [delay]);
}
在我的組件中,我將它用作計時器 - 在依賴項中聲明的時間之后執行操作
這是我開始工作的一個例子:
import { mount } from 'enzyme';
import React from 'react';
import useInterval from 'YOUR CODE ABOVE'; // <------- Adjust this Import
function MockComponent({ timer, updateTimer }) {
const handleStartButton = () => {
updateTimer({ ...timer });
};
useInterval(
() => {
updateTimer({ ...timer });
},
timer.isTicking ? 1000 : null
);
return (
<button type="button" className="start" onClick={() => handleStartButton()}>
Start Countdown
</button>
);
}
describe('useInterval', () => {
const spyFunction = jest.fn();
const timer = {
isTicking: true,
};
let wrapper = null;
jest.useFakeTimers();
beforeEach(() => {
wrapper = mount(<MockComponent updateTimer={spyFunction} timer={timer} />);
});
afterEach(() => {
wrapper.unmount();
wrapper = null;
});
it('timer should run', () => {
expect(spyFunction).not.toHaveBeenCalled();
wrapper.find('button.start').simulate('click');
// works as expected
expect(spyFunction).toHaveBeenCalled();
// Increment
jest.advanceTimersByTime(1000);
// It should have been called at least twice.
expect(spyFunction).toHaveBeenCalledTimes(2);
});
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.