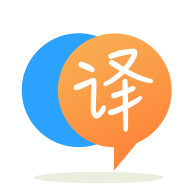
[英]Finding the largest palindrome of the product of two three digit numbers problem
[英]Finding largest palindrome from product of two 3 digit numbers (Project Euler problem)
我了解此問題存在各種解決方案,但查看解決方案后,我不確定我的代碼在哪里失敗。 這是我的參考代碼:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *strrev(char *str)
{
if (!str || ! *str)
return str;
int i = strlen(str) - 1, j = 0;
char ch;
while (i > j)
{
ch = str[i];
str[i] = str[j];
str[j] = ch;
i--;
j++;
}
return str;
}
int main(){
long int a,b, ab, maxA, maxB, pal;
long int maxPal = 0;
char string[6];
char revString[6];
for(a=999; a>100; a--){
for(b=999; b>100; b--){
ab = a*b;
sprintf(string,"%ld",ab);
strcpy(revString, strrev(string));
strrev(string);
if((revString[0] != '0') && (strcmp(string, revString)== 0)){
printf("Palindrome %ld found!\n", ab);
pal = ab;
if(pal > maxPal){
maxPal = pal;
maxA = a;
maxB = b;
}
}
}
}
printf("Maximim palindrome is %ld from the product of a = %ld and b = %ld \n", maxPal, maxA, maxB);
return 1;
}
我正在通過蠻力方法嘗試此操作,方法是遍歷從 100 到 999 的所有數字對,將乘積轉換為字符串,然后查看字符串是否向后相同。 但是這樣做我只找到一個 5 位數字作為最大回文而不是 6 位數字。 我認為我的邏輯似乎很簡單,但我得出了錯誤的結論。 我想了解我的邏輯不正確之處,而不是正確答案。
我已修改您的代碼以計算找到的回文數:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *strrev(char *str)
{
if (!str || ! *str)
return str;
int i = strlen(str) - 1, j = 0;
char ch;
while (i > j)
{
ch = str[i];
str[i] = str[j];
str[j] = ch;
i--;
j++;
}
return str;
}
int main(){
long int a,b, ab, maxA, maxB, pal;
long int maxPal = 0;
char string[6];
char revString[6];
int n6dp = 0;
int n5dp = 0;
for(a=999; a>100; a--){
for(b=999; b>100; b--){
ab = a*b;
sprintf(string,"%ld",ab);
strcpy(revString, strrev(string));
strrev(string);
if((revString[0] != '0') && (strcmp(string, revString)== 0)){
if (strlen(string) == 6)
{
n6dp++;
}
if (strlen(string) == 5)
{
n5dp++;
}
pal = ab;
if(pal > maxPal){
maxPal = pal;
maxA = a;
maxB = b;
}
}
}
}
printf("number of 5 digits palindroms found = %d\n", n5dp);
printf("number of 6 digits palindroms found = %d\n", n6dp);
printf("Max. palindrome is %ld from the product of a = %ld and b = %ld \n", maxPal, maxA, maxB);
return 0;
}
這是我得到的:
$ ./pal
number of 5 digits palindroms found = 1493
number of 6 digits palindroms found = 977
Max. palindrome is 906609 from the product of a = 993 and b = 913
您的代碼找到了 6 位回文。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.