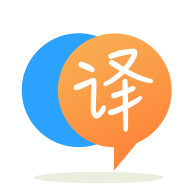
[英]How to convert a date a user selects in MM-dd-yyyy format to the format yyyy-MM-dd in Java
[英]How to return a user input date as dd/mm/yyyy in Java?
用戶需要輸入任務的名稱、日期、位置等。 然后,該程序旨在列出每個任務,輸出名稱、日期、位置等。
當用戶輸入日期時,日期的格式不是我需要的。
例子:
Enter a date: 21/07/2020
Output:
Tue Jul 21 00:00:00 AEST 2020
所需的 output:
21/07/2020
到目前為止,這是我的代碼:-我正在使用多個類
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Scanner;
class Task{
private String theTitle;
private Date theDate;
private String theTime;
private String theLocation;
private String theDuration;
private String theCategory;
SimpleDateFormat format=new SimpleDateFormat("dd/MM/yyyy");
Task(String title, Date date, String time, String location, String duration, String category) {
theTitle = title;
theDate = date;
theTime = time;
theLocation = location;
theDuration = duration;
theCategory = category;
}
public String getTitle() {
return theTitle;
}
public Date getDate() {
return theDate;
}
public String getTime() {
return theTime;
}
public String getLocation() {
return theLocation;
}
public String getDuration() {
return theDuration;
}
public String getCategory() {
return theCategory;
}
public String getItem() {
return theTitle + ", " + theDate + ", " + theTime + ", " + theLocation + ", " + theDuration + ", " + theCategory;
}
}
public class ToDoList2 {
public Task myTaskObj;
SimpleDateFormat format=new SimpleDateFormat("dd/MM/yyyy");
private static List<String> currentList = new ArrayList<String>();
public ToDoList2() {
}
public static void main (String[] args) throws ParseException {
ToDoList listObj = new ToDoList();
int menuItem = -1;
while (menuItem != 3) {
menuItem = listObj.printMenu();
switch (menuItem) {
case 1:
listObj.addItem();
break;
case 2:
listObj.showList();
break;
case 3:
System.out.println("Goodbye!");
default:
System.out.println("Enter a valid option");
}
}
}
public int printMenu() {
Scanner scanner = new Scanner(System.in);
System.out.println();
System.out.println("----------------------");
System.out.println("Main Menu");
System.out.println("----------------------");
System.out.println("1. Add a task");
System.out.println("2. List tasks");
System.out.println("3. Leave the program");
System.out.println();
System.out.print("Enter choice: ");
int choice = scanner.nextInt();
return choice;
}
public void showList() {
System.out.println();
System.out.println("----------------------");
System.out.println("To-Do List");
System.out.println("----------------------");
int number = 0;
for (String item : currentList) {
System.out.println(++number + ". " + item);
}
System.out.println("----------------------");
}
public void addItem() throws ParseException {
System.out.println("Add a task");
System.out.println("----------------------");
System.out.print("Enter the task title: ");
Scanner scanner = new Scanner(System.in);
String title = scanner.nextLine();
System.out.print("Enter the task date (dd/mm/yyyy): ");
Scanner scanner2 = new Scanner(System.in);
Date date=format.parse(scanner2.next());
System.out.print("Enter the task time: ");
Scanner scanner3 = new Scanner(System.in);
String time = scanner3.nextLine();
System.out.print("Enter the task location: ");
Scanner scanner4 = new Scanner(System.in);
String location = scanner4.nextLine();
System.out.println("Enter the task duration (optional - press enter to skip): ");
Scanner scanner5 = new Scanner(System.in);
String duration = scanner5.nextLine();
System.out.println("Enter the task category (optional - press enter to skip): ");
Scanner scanner6 = new Scanner(System.in);
String category = scanner6.nextLine();
myTaskObj = new Task(title, date, time, location, duration, category);
String theItem = myTaskObj.getItem();
currentList.add(theItem);
System.out.println("Task Added!");
}
}
我對 java 非常陌生,因此我們將不勝感激!
您已經定義了 simpleDateFormat 但似乎沒有使用它。 它將專門滿足您的需求。
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy");
System.out.println(sdf.format(yourDate));
你需要在里面添加這個邏輯
public String getItem() {
return theTitle + ", " + sdf.format(theDate) + ", " + theTime + ", " + theLocation + ", " + theDuration + ", " + theCategory;
}
由於您沒有在那里指定任何內容,因此它在字符串連接時調用 Date.toString() 方法。
我建議您從過時且容易出錯的java.util
日期時間 API 切換到豐富的現代日期時間 API 集。
定義一個格式化程序 object:
DateTimeFormatter.ofPattern("dd/MM/yyyy")
…並使用相同的DateTimeFormatter
object 來解析和生成文本。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Scanner;
public class Main {
public static void main(final String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter date in the format dd/MM/yyyy: ");
String dateStr = scanner.nextLine();
// Define a formatter
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
// Parse the date string to LocalDate using the formatter
LocalDate theDate = LocalDate.parse(dateStr, formatter);
// Whenever you need to display it, display it using the same formatter
String backToStr = formatter.format(theDate);
System.out.println(backToStr);
}
}
示例運行:
Enter date in the format dd/MM/yyyy: 21/07/2020
21/07/2020
附注:我可以看到您為每個輸入創建了一個新的Scanner
實例。 您應該只創建一個Scanner
實例,並為每個輸入使用相同的實例。
將此添加到您的代碼中以獲得正確的格式
String pattern = "dd/MM/yyyy";
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern);
String formatedDate = simpleDateFormat.format(date);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.