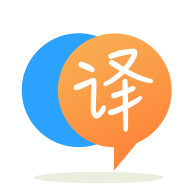
[英]How do I call a member function inside a non-member function in C++ with an object created outside the non-member function?
[英]How do you call a non-member function with a template in c++, where the typename is only in the return?
我的目標是讓非成員 function 使用模板作為返回值。 這樣我就可以返回一個浮點數組、一個雙精度數組等。我收到“無法推斷模板參數'T'”錯誤。
這是我嘗試使用的方法:
template<typename T>
T* make(double magnitude, double frequency, double radians, double seconds,
int samplesPerSecond) {
long samples = length(seconds, samplesPerSecond);
T *wave = new T[samples];
for (int i = 0; i < samples; i++) {
wave[i] = magnitude
* sin(
(2.0 * (M_PI) * frequency * i / samplesPerSecond)
+ radians);
}
return wave;
}
我首先嘗試正常調用該方法。
double *ds = make(magnitude, frequency, radians, seconds, samplesPerSecond);
我嘗試在 function 名稱之后添加類型名稱。
double *ds = make<double>(magnitude, frequency, radians, seconds, samplesPerSecond);
我查看了 web 上的幾頁,到目前為止它們已經涵蓋了所有成員函數,並解釋了不能從返回類型推斷出類型,那么你如何告訴編譯器類型呢?
您可以使用非成員函數執行此操作,如下例所示,它將模板化類型的兩個值相加:
#include <iostream>
template<typename T> T add(const T &val1, const T &val2) {
return val1 + val2;
}
int main() {
auto eleven = add<int>(4, 7);
std::cout << "4 + 7 = " << eleven << '\n';
auto threePointFour = add<double>(1.1, 2.3);
std::cout << "1.1 + 2.3 = " << threePointFour << '\n';
}
正如預期的那樣,該代碼的 output 是:
4 + 7 = 11
1.1 + 2.3 = 3.4
您的案例可能不起作用,因為模板化 function 的定義可能不正確 - 因為您沒有提供,所以很難確定。 因此,您可能想看看它與我自己add
的 function 相比如何,如上所示。
順便說一句(因為您的標題表明這可能是您正在嘗試的),您也可以在不使用參數列表中的模板類型(僅返回類型)的情況下執行此操作:
#include <iostream>
template<typename T> T addAndHalve(const double &val1, const double &val2) {
auto x = (val1 + val2) / 2;
std::cout << "debug " << x << ": ";
return x;
}
int main() {
auto eleven = addAndHalve<int>(4, 7);
std::cout << "(4 + 7 ) / 2 = " << eleven << '\n';
auto threePointFour = addAndHalve<double>(1.1, 2.3);
std::cout << "(1.1 + 2.3) / 2 = " << threePointFour << '\n';
}
您可以看到這僅影響返回值,對所有參數使用double
精度:
debug 5.5: (4 + 7 ) / 2 = 5
debug 1.7: (1.1 + 2.3) / 2 = 1.7
我注意到 c++ 標准庫有類似 lround() 的函數,其中 l 表示 function 返回一個 long,所以我最終也創建了多個方法。
int16_t* makei(double magnitude, double frequency, double radians,
double seconds, int samplesPerSecond);
float* makef(double magnitude, double frequency, double radians, double seconds,
int samplesPerSecond);
這違反了我經常聽到的“避免不必要的重載”規則,下面的代碼確實有效。
double *ds = make<double>(magnitude, frequency, radians, seconds, samplesPerSecond);
我有另一個未知錯誤阻止它工作。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.