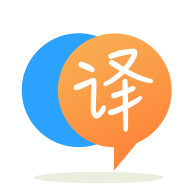
[英]How to continue to next loop iteration only after a button press in javascript?
[英]Javascript forEach loop won't continue to next iteration
我正在嘗試完成這個挑戰,當元素兩側的值之和相等時,function 應該返回元素的索引值。 例如,[1,2,3,4,3,2,1] 應該返回 3,因為在 '4' 的另一側,值加到 6 (1+2+3) 和 (3+2+1)。 此外,如果沒有這樣的值,那么 function 應該返回 -1。
function findEvenIndex(arr) {
arr.forEach((element, index) => {
let a = arr.splice(index + 1, arr.length); //array of values after current value
let b = arr.splice(arr[0], index); //array of values before current value
let suma = a.reduce((accumulator, currentValue) => { //Sum of array of after values
return accumulator + currentValue;
}, 0);
let sumb = b.reduce((accumulator, currentValue) => { //Sum of array of before values
return accumulator + currentValue;
}, 0);
if (suma === sumb) { //comparing the two sums to check if they are equal
return index;
};
});
};
我的理解是,如果 suma 和 sumb 不相等,則 forLoop 的下一次迭代將開始,但是這不會發生,我不明白為什么。
如果不存在這樣的值,function 應該返回 -1,我目前還沒有實現這部分代碼。
謝謝
您的代碼有兩個問題:
Array.prototype.slice
會在原地改變/更改數組,當您同時迭代數組時,這是一個壞主意。 因此,在拼接之前制作一個數組的淺拷貝,通過使用擴展運算符,即[...arr].splice()
findEvenIndex()
function 返回。 更好的解決方案是簡單地使用for
循環:一旦找到索引,我們可以使用break
來短路並跳出循環,因為我們不想執行進一步的分析。 我們將索引存儲在for
循環之外的變量中,然后返回它:
function findEvenIndex(arr) { let foundIndex = -1; for(let index = 0; index < arr.length; index++) { const a = [...arr].splice(index + 1, arr.length); //array of values after current value const b = [...arr].splice(0, index); //array of values before current value const suma = a.reduce((accumulator, currentValue) => { //Sum of array of after values return accumulator + currentValue; }, 0); const sumb = b.reduce((accumulator, currentValue) => { //Sum of array of before values return accumulator + currentValue; }, 0); if (suma === sumb) { //comparing the two sums to check if they are equal foundIndex = index; break; }; }; return foundIndex; }; console.log(findEvenIndex([1,2,3,4,3,2,1]));
您應該使用 slice 方法而不是 splice 並在循環外返回索引
function findEvenIndex(arr) {
var result = -1;
arr.forEach((element, index) => {
let a = arr.slice(index + 1);
let b = arr.slice(0, index);
let suma = a.reduce((accumulator, currentValue) => {
//Sum of array of after values
return accumulator + currentValue;
}, 0);
let sumb = b.reduce((accumulator, currentValue) => {
//Sum of array of before values
return accumulator + currentValue;
}, 0);
if (suma === sumb) {
//comparing the two sums to check if they are equal
result = index;
}
});
return result;
}
你也可以使用 findIndex 方法
const sum = (a,b)=> a+b;
const findEvenIndex = (TestArr) =>
TestArr.findIndex(
(_, i) =>
TestArr.slice(0, i).reduce(sum, 0) === TestArr.slice(i + 1).reduce(sum, 0)
); ;
一些筆記。 利用內置的.findIndex()
。 使用slice
,因為它返回數組的更改副本。 slice
/ splice
將索引作為 arguments,所以不要在這些方法中使用arr[0]
。
function findEvenIndex(arr) {
return arr.findIndex((element, index) => {
let a = arr.slice(index + 1); //array of values after current value
let b = arr.slice(0, index); //array of values before current value
let suma = a.reduce((accumulator, currentValue) => { //Sum of array of after values
return accumulator + currentValue;
}, 0);
let sumb = b.reduce((accumulator, currentValue) => { //Sum of array of before values
return accumulator + currentValue;
}, 0);
return suma===sumb;
});
};
您可以在不更改數組的情況下采用快速方法,並為實際delta
使用兩個索引和一個變量,該增量是通過添加左側值和減去右側值來構建的。
如果索引不按順序退出循環。
然后檢查delta
。 如果delta
為零,則返回左索引或-1
表示未找到分離索引。
function getIndex(array) { let delta = 0, i = 0, j = array.length - 1; while (i < j) { if (delta <= 0) { delta += array[i++]; continue; } delta -= array[j--]; } return delta? -1: i; } console.log(getIndex([1, 2])); // -1 console.log(getIndex([1, 2, 3, 4, 3, 2, 1])); // 3 console.log(getIndex([1, 2, 2, 2, 4, 3, 2, 2])); // 4
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.