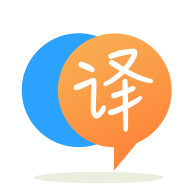
[英]React Native: how do you include constructor with functional components?
[英]What is solution for constructor in functional components in react native?
讓我帶你解決我的問題。 我正在制作一個計時器功能組件,我將 startValue 傳遞給組件,然后該組件將使用通過 props 傳遞的 startValue 啟動計時器(以秒為單位減少一秒)。
const FunctionalComponent = (props: any) => {
const [timerValue, setTimerValue] = useState(props.initialValue)
console.log('Set State')
useEffect(() => {
console.log('UseEffects called')
setInterval(() => {
setTimerValue(timerValue - 1)
}, 1000)
}, [])
return <View><Text style={styles.textStyle}>{timerValue}</Text></View>
}
我在 Parent 中渲染 function。
render() {
return <View style={styles.mainView}>
<FunctionalComponent initialValue={30} />
</View>
}
現在,每次 react 重新渲染父組件時,FunctionalComponent 都會被調用並重置 timerValue 值。 我使用 class 組件構造函數解決了這個問題,但我想知道在功能組件中是否有任何解決方案可以做到這一點。
class OTPTimer extends Component {
constructor(props: any) {
super(props)
this.state = {
timeLeft: props.fromStart
}
if (props.startTimer) {
this.startTimer()
}
}
componentDidUpdate(prevProps: any) {
if (!prevProps.startTimer && this.props.startTimer) {
this.startTimer()
this.setState({
timeLeft: this.props.fromStart
})
}
}
startTimer = () => {
var interval = setInterval(() => {
this.setState({
timeLeft: this.state.timeLeft - 1
})
if (this.state.timeLeft === 0) {
clearInterval(interval)
}
}, 1000)
}
render() {
return <Text style={globalStyles.testStyleThree}>{`00:${this.state.timeLeft > 9 ? this.state.timeLeft : `0${this.state.timeLeft}`}`}</Text>
}
}
這就是使用React.memo的意義所在,以防止在子組件的 props 不變時重新渲染它們。
React.memo 是一個高階組件。 它與 React.PureComponent 類似,但用於 function 組件而不是類。
如果您的 function 組件在給定相同的 props 的情況下呈現相同的結果,您可以將其包裝在對 React.memo 的調用中,以便在某些情況下通過記憶結果來提高性能。 這意味着 React 將跳過渲染組件,並重用上次渲染的結果。
const FunctionalComponent = React.memo<{initialValue: number}>({initialValue}) => {
const [timerValue, setTimerValue] = useState(initialValue)
console.log('Set State')
useEffect(() => {
console.log('UseEffects called')
setInterval(() => {
setTimerValue(timerValue - 1)
}, 1000)
}, [])
return <View><Text style={styles.textStyle}>{timerValue}
</Text></View>
};
簽出 React.memo ,如果子組件的 props 沒有改變,女巫將阻止重新渲染
const FunctionalComponent = React.memo((props: any) => { .... } )
人們建議useEffect
但它會在渲染后調用。
使用useMemo
代替:
useMemo(() => {
console.log('This is useMemo')
}, []);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.