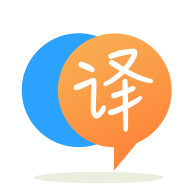
[英]Dynamically select the element from the list of elements based on child index
[英]Remove item from the list dynamically if there are no child elements
我有 3 個數組列表,如下所示:
groups = [ { grpId: 1, grpName: "Vegetables" }, { grpId: 2, grpName: "Fruits" }, { grpId: 3, grpName: "Staples" }];
subGrp = [ { subGrpId: "V1", grpId: 1, subGrpName: "Carrot" }, { subGrpId: "F1", grpId: 2, subGrpName: "Apple" },
{ subGrpId: "S1", grpId: 3, subGrpName: "Dal" }];
quantity = [ { quantityValue: "1kg", subGrpId: "V1" }, { quantityValue: "1kg", subGrpId: "S1" }];
條件:如果沒有任何與“subGrp”數組相關的“數量”,那么我們需要從自定義列表中刪除整個條目。
使用上面的數組列表,我正在嘗試構建一個自定義列表,如下所示:
const grpSet = new Set(this.groups.map(i => ({ id: i.grpId, name: i.grpName })));
grpSet.forEach(g =>
this.groceryList.push({
grp: g["name"],
grpTypeList: this.subGrp.filter(el => {
if (el.grpId === g["id"] && this.quantity) {
el["quantities"] = this.quantity.filter(
v => v.subGrpId === el["subGrpId"]
);
if (el["quantities"].length > 0) {
return el;
}
}
})
})
);
// above logic will lead us to below custom list.
從此,我需要刪除 Fruits 條目,因為 grpTypeList 是空的。 但我的邏輯不允許我這樣做。
[{
"grp": "Vegetables",
"grpTypeList": [{
"subGrpId": "V1",
"grpId": 1,
"subGrpName": "Carrot",
"quantities": [{
"quantityValue": "1kg",
"subGrpId": "V1"
},
{
"quantityValue": "2kg",
"subGrpId": "V1"
}
]
}]
},
// remove this complete object as grpTypeList is empty
{
"grp": "Fruits",
"grpTypeList": [
]
},
{
"grp": "Staples",
"grpTypeList": [{
"subGrpId": "S1",
"grpId": 3,
"subGrpName": "Dal",
"quantities": [{
"quantityValue": "1kg",
"subGrpId": "S1"
}]
}]
} ]
使用上面的列表,我正在繪制我的 html 以顯示如下圖:
您需要注意一些細微的更改,請使用以下幾行更新您的代碼:
import { Component, OnInit } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent implements OnInit {
groceryList = [];
groups = [
{ grpId: 1, grpName: "Vegetables" },
{ grpId: 2, grpName: "Fruits" },
{ grpId: 3, grpName: "Staples" }
];
subGrp = [
{ subGrpId: "V1", grpId: 1, subGrpName: "Carrot" },
{ subGrpId: "F1", grpId: 2, subGrpName: "Apple" },
{ subGrpId: "S1", grpId: 3, subGrpName: "Dal" }
];
quantity = [
{ quantityValue: "1kg", subGrpId: "V1" },
{ quantityValue: "2kg", subGrpId: "V1" },
{ quantityValue: "1kg", subGrpId: "S1" }
];
ngOnInit() {
this.groceryList = [];
const grpSet = new Set(
this.groups.map(i => ({ id: i.grpId, name: i.grpName }))
);
grpSet.forEach(g =>{
let element:any[];
element= this.subGrp.filter(el => {
if (el.grpId === g["id"] && this.quantity) {
el["quantities"] = this.quantity.filter(
v => v.subGrpId === el["subGrpId"]
);
if (el["quantities"].length > 0) {
return el;
}
}
})
if(element && element.length){
this.groceryList.push({
grp:g["name"],
grpTypeList:element
})
}
}
);
console.log(JSON.stringify(this.groceryList));
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.