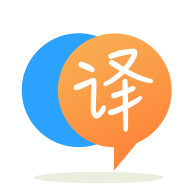
[英]How to check if mouse pressed and still pressed on a button in java swing app
[英]How to find what button was pressed last in Java Swing
我現在正在 java swing 項目上使用 Java JDK 14.0.2。 我正在使用 Eclipse (如果重要的話)。 我正在嘗試使用附加到我的 JButtons 的動作偵聽器來查看之前按下了哪個按鈕,然后根據之前按下的按鈕執行不同的操作。
我有以下項目:
TestFrame.java
package TestSwing;
import javax.swing.JFrame;
public class TestFrame extends JFrame {
public TestFrame() {
super();
this.add(new TestPanel());
this.pack();
this.setLocationRelativeTo(null);
this.setResizable(false);
this.setVisible(true);
}
}
TestFrame.java 在主要方法所在的不同文件中被實例化。
測試面板.Java
package TestSwing;
import javax.swing.JPanel;
public class TestPanel extends JPanel {
public TestPanel() {
super();
this.add(new TestButton1());
this.add(new TestButton2());
}
}
現在問題的重要部分兩個按鈕:
測試按鈕1.java
package TestSwing;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class TestButton1 extends JButton {
public TestButton1() {
super();
this.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (/*last button pressed before this one is TestButton2*/) {
System.out.println("Button 2 was pressed last");
}
else if (/*last button pressed before this one was itself*/) { // It is important that this is else if
System.out.println("Button 1 was pressed last");
}
}
});
this.setText("Button 1");
}
}
這是按鈕 2:
package TestSwing;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class TestButton2 extends JButton {
public TestButton2() {
super();
this.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
;
}
});
this.setText("Buttton 2");
}
}
我已經嘗試為此尋找解決方案,但找不到我正在尋找的東西,而且我不知道如何自己開始這樣做。 在此先感謝您的幫助。
e.getSource()
獲得)。 這將保持對最后按下按鈕的引用。例如:
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.event.ActionListener;
import javax.swing.*;
public class LastButtonPressed {
private JButton lastButton = null;
private JButton previousButton = null;
private JTextField lastButtonsText = new JTextField(10);
private JTextField previousButtonsText = new JTextField(10);
private JPanel mainPanel = new JPanel(new BorderLayout());
public LastButtonPressed() {
int sides = 8;
JPanel buttonGridPanel = new JPanel(new GridLayout(sides, sides));
ActionListener listener = e -> {
previousButton = lastButton;
lastButton = (JButton) e.getSource();
previousButtonsText.setText(lastButtonsText.getText());
lastButtonsText.setText(e.getActionCommand());
};
for (int i = 0; i < sides * sides; i++) {
String text = "Button " + (i + 1);
JButton button = new JButton(text);
button.addActionListener(listener);
buttonGridPanel.add(button);
}
JPanel topPanel = new JPanel();
topPanel.add(new JLabel("Previous Button:"));
topPanel.add(previousButtonsText);
topPanel.add(Box.createHorizontalStrut(20));
topPanel.add(new JLabel("Last Button:"));
topPanel.add(lastButtonsText);
mainPanel.add(topPanel, BorderLayout.PAGE_START);
mainPanel.add(buttonGridPanel);
}
public JPanel getMainPanel() {
return mainPanel;
}
public JButton getLastButton() {
return lastButton;
}
public JButton getPreviousButton() {
return previousButton;
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Last Button Pressed");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new LastButtonPressed().getMainPanel());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
});
}
}
保存最后按下的按鈕以及在最后一個按鈕之前按下的上一個按鈕的變量(字段):
private JButton lastButton = null;
private JButton previousButton = null;
JTextFields 顯示這些最后一個按鈕上的文本:
private JTextField lastButtonsText = new JTextField(10);
private JTextField previousButtonsText = new JTextField(10);
包含應用程序的主 JPanel:
private JPanel mainPanel = new JPanel(new BorderLayout());
創建一個包含 8x8 網格的 JButton 的 JPanel:
int sides = 8;
JPanel buttonGridPanel = new JPanel(new GridLayout(sides, sides));
創建一個添加到按鈕的 ActionListener。 在偵聽器內部,設置上一個按鈕以及最后一個按鈕,並更新 JTextField 保存的文本:
ActionListener listener = e -> {
previousButton = lastButton;
lastButton = (JButton) e.getSource();
previousButtonsText.setText(lastButtonsText.getText());
lastButtonsText.setText(e.getActionCommand());
};
在 for 循環中,創建 8x8 JButton,將 ActionListener 添加到每個按鈕,並將每個按鈕添加到 JPanel 網格:
for (int i = 0; i < sides * sides; i++) {
String text = "Button " + (i + 1);
JButton button = new JButton(text);
button.addActionListener(listener);
buttonGridPanel.add(button);
}
將所有內容添加到子 JPanel 和主 JPanel:
JPanel topPanel = new JPanel();
topPanel.add(new JLabel("Previous Button:"));
topPanel.add(previousButtonsText);
topPanel.add(Box.createHorizontalStrut(20));
topPanel.add(new JLabel("Last Button:"));
topPanel.add(lastButtonsText);
mainPanel.add(topPanel, BorderLayout.PAGE_START);
mainPanel.add(buttonGridPanel);
Create a JFrame in a Swing thread-safe manner, create our LastButtonPressed instance and add its main JPanel to the JFrame, and finally display the JFrame:
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Last Button Pressed");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new LastButtonPressed().getMainPanel());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.