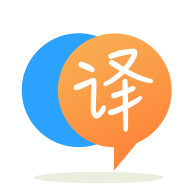
[英]How can i call a function, whose name is dynamically created by combining some variables in node.js?
[英]I can't call a function created inside another function using Node.JS
我使用此代碼的目標:此代碼應隨機生成一個數學方程式,用戶應在其中輸入結果(用戶認為正確的結果)。 然后計算機應將用戶輸入的輸入(結果)與真實結果進行比較。 如果結果正確,它應該控制台日志(“好”),如果它不正確,它應該控制台日志(“壞”),無論哪種方式,點擊提交按鈕后,網站應該刷新並隨機生成一個新方程(並且該過程再次重復)。
問題:我有 function equationGenerator
生成隨機方程並計算結果。 I need to call equationGenerator
inside the app.get
function in node.js so that every-time the website is refreshed a new function appears, unfortunately the function is not being called and I just get errors now when trying to load the website.
這是代碼:
////Installations (not the actual code)
const bodyParser = require("body-parser");
const express = require("express");
const ejs = require("ejs");
const app = express();
app.set('view engine', 'ejs');
app.use(bodyParser.urlencoded({extended: true}));
app.use(express.static("public"));
////The actual code
function equationGenerator(){
var random = Math.round(Math.random() * 1);
var firstNumber = random ? (Math.random() * (10000) - 5000).toFixed(2) : (Math.round(Math.random() * (100)) - 50);
var secondNumber = random ? (Math.random() * (10000) - 5000).toFixed(2) : (Math.round(Math.random() * (100)) - 50);
var operations = random ? ["+", "-"] : ["*", "/"];
var randomOperation = operations[Math.round(Math.random() * 1)];
var expression = firstNumber + randomOperation + secondNumber;
var resultNotInterger = eval(expression);
var result = Number(resultNotInterger).toFixed(2);
return {
firstNumber,
secondNumber,
randomOperation,
result,
};
}
app.get("/", function(request, response){
equationGenerator()
response.render("index.ejs", {
firstNumber: firstNumber,
secondNumber: secondNumber,
randomOperation: randomOperation,
result: result
});
});
app.post("/", function(request, response){
const clientResponse = Number(request.body.clientResponse).toFixed(2);
if (clientResponse === result){
console.log("Good");
}else{
console.log("Bad");
}
response.redirect("/");
});
/////Server start code
app.listen(2109, function(err){
if (err){
console.log(err + "JHGKGFKUYGLUYFLIFYGUFYOIY:GYP");
}else{
console.log("Running on port 2109");
}
});
這是ejs代碼:
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Math Ecercise</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous">
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>Math</h1>
<p><%= firstNumber %> <%= randomOperation %> <%= secondNumber %> = <%=
result %></p>
<form class="" action="/" method="post">
<div class="lediv">
<input class="form-control" type="text" name="clientResponse" value="" placeholder="result" autocomplete="off">
<button class="btn btn-primary btn-info" type="submit" name="button">Submit</button>
</div>
<p class="message">Haha</p>
</form>
</body>
</html>
如果您有任何困惑,我將非常樂意回答任何問題,因為我已經嘗試解決這個問題好幾天了。
太感謝了!
這是下載到 wetransfer 中完整文件的鏈接 - https://wetransfer.com/downloads/8f193e12b9505c086728d3d5e976e11f20200728000447/51fde6 。 確保您使用app1.1.js進行嘗試。我有很多版本,但這是當前版本。
如果您有任何困惑,我將非常樂意回答任何問題,因為我已經嘗試解決這個問題好幾天了。
太感謝了!
equationGenerator()
返回一個 object 並且您沒有將 object 分配給任何東西,所以它只是丟失了。 由於返回的 object 與您要傳遞的內容完全匹配response.render()
,您可以直接傳遞它:
app.get("/", function(request, response){
const data = equationGenerator()
response.render("index.ejs", data);
});
或者,甚至只是:
app.get("/", function(request, response){
response.render("index.ejs", equationGenerator());
});
如果返回的 object 與您想要傳遞的內容不完全匹配,那么您可以將 object 分配給一個變量並挑選出您想要傳遞的 object 的片段:
app.get("/", function(request, response){
const data = equationGenerator()
response.render("index.ejs", {
firstNumber: data.firstNumber,
secondNumber: data.secondNumber,
randomOperation: data.randomOperation,
result: data.result
});
});
或者,您可以使用解構賦值來完成同樣的任務,方法是分配給局部變量,然后使用這些值構建一個新的 object:
app.get("/", function(request, response){
const {
firstNumber,
secondNumber,
randomOperation,
result
} = equationGenerator();
response.render("index.ejs", {
firstNumber,
secondNumber,
randomOperation,
result
});
});
顯然,由於返回的數據 object 已經是您要傳遞給res.render()
的數據,所以上面的第一個選項是最簡單的。
關於在表單發布時比較結果的問題的第二部分(在評論中)
OP 想知道如何在生成頁面時“保存” result
,以便以后在提交包含用戶條目的表單時將其與提交的結果進行比較。
無狀態解決方案(沒有跨請求存儲在服務器上的中間 state)通常是可取的(如果可行)。 我可以想到兩種無狀態的解決方案。
將輸入數據與表單提交一起放入(作為隱藏字段,以便在提交表單時將它們發送回服務器)並在提交表單時在服務器上計算所需的結果。 然后,您可以將用戶結果與服務器計算的結果進行比較,無需在任何地方“保存”預先計算的結果。
Go 提前並像你一樣計算結果,但加密結果並將其放入表單(作為隱藏字段),以便將其與答案一起提交回服務器。 然后,當您收到 POST 表單時,您解密result
並與用戶的結果進行檢查。
也有非無狀態的解決方案。 您可以為每個問題頁面生成一個隨機表單 ID,並將其作為隱藏字段嵌入表單中,以便將其與表單一起提交回來。 然后,您可以使用express-session
保存用戶 state。 在該用戶中 state 可以是Map
object ,其中包含 formID 的鍵、值對formID,result
。 提交表單時,從表單中獲取formID,在用戶的session object中查找預期結果,然后將提交的結果與預期結果進行比較。 您將需要一些方案來老化用戶從未使用過的 session object 中的 formID(因為他們從未提交過表單,或者因為他們多次刷新生成一堆他們從未使用過的 formID)。
app.get("/", function(request, response){
equationGenerator()
response.render("index.ejs", {
firstNumber: firstNumber,
secondNumber: secondNumber,
randomOperation: randomOperation,
result: result
});
});
您沒有在那里使用 equationGenerator() 的返回值。
嘗試以下操作:
app.get("/", function(request, response){
response.render("index.ejs", equationGenerator());
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.