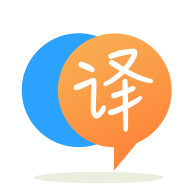
[英]How to write a unit test for custom Rest template interceptor in spring boot
[英]Spring boot : How to write unit test case for delete rest template
我正在嘗試為 HttpHandler class 編寫單元測試用例,其中有 rest 模板調用刪除。 我已經創建了一個用戶控制器 class 來進行 resttemplate 調用,以測試 HttpHandler class 中sendDelete
的功能。 有人可以幫助我理解在HtttpHandler
class 中為sendDelete
方法編寫單元測試用例的正確方法是什么?
我有一個 class HttpHandler。 它有一個 function sendDelete
它調用resttemplate.exchange
方法
@Service
public class HttpHandler {
public <T,R> ResponseEntity<Void> sendDelete(String url, HttpHeaders httpHeaders, R requestBody, Class<T> responseClass) {
//create an instance of rest template
RestTemplate restTemplate = new RestTemplate();
HttpEntity<R> entity = new HttpEntity<R>(requestBody, httpHeaders);
logger.info("DELETE request to " + url + " with body: " + JsonUtil.jsonizeExcludeNulls(requestBody));
//make an HTTP DELETE request with headers
ResponseEntity<Void> response = restTemplate.exchange(url, HttpMethod.DELETE, entity, Void.class);
logger.info("DELETE" + url + ": " + JsonUtil.jsonize(response));
return response;
}
}
我正在使用junit5。 下面是上面 class 中 sendDelete 方法的單元測試用例:
@LocalServerPort
private int port;
private String baseUrl;
@Autowired
private HttpHandler httpHandler;
@BeforeEach
public void setBaseUrl(){
this.baseUrl = "http://localhost:"+ port + "/users";
}
@Test
public void testSuccessDeleteUserById() throws Exception{
this.baseUrl = baseUrl + "/1";
//create headers
HttpHeaders httpHeaders = new HttpHeaders();
//set content type
httpHeaders.setContentType(MediaType.APPLICATION_JSON);
httpHeaders.setAccept(Collections.singletonList(MediaType.APPLICATION_JSON));
//make an HTTP DELETE request with headers
ResponseEntity<Void> actual = httpHandler.sendDelete(baseUrl, httpHeaders, null, Void.class);
assertEquals(404, actual.getStatusCodeValue());
}
下面是用戶 controller class
@RestController
public class UserController {
@DeleteMapping("/users/{userId}")
public ResponseEntity<Void> deleteUser(@PathVariable("userId") int userId){
return new ResponseEntity<Void>(HttpStatus.NOT_FOUND);
}
}
感謝您的時間!
有兩種方法可以做到這一點。
Mocking 休息模板。 為此,首先,您必須使 RestTemplate 成為一個字段,並通過構造函數(或任何其他方式)將其注入。 這允許您注入一個模擬 object。 那么,rest就是簡單明了的mocking。
您可以使用MockWebServer 。 這樣你就不需要改變任何東西。 您的方法會將請求發送到的只是 web 服務器。 方法調用完成后,您可以訪問記錄的請求並進行一些驗證。
這是一個粗略的例子。 如果您將有很多這些測試,那么您可以將 web 服務器初始化移動到@BeforeEach
方法並將銷毀移動到@AfterEach
方法。
public class HttpHandlerTest {
private final HttpHandler handler = new HttpHandler();
@Test
@SneakyThrows
public void testDelete() {
MockWebServer mockWebServer = new MockWebServer();
mockWebServer.start(9889);
mockWebServer.enqueue(
new MockResponse().setResponseCode(200)
);
String url = "http://localhost:9889";
Hello hello = new Hello("hello world");
final ResponseEntity<Void> entity = handler.sendDelete(url, null, hello, Hello.class);
assertNotNull(entity);
assertEquals(200, entity.getStatusCode().value());
final RecordedRequest recordedRequest = mockWebServer.takeRequest();
assertEquals("DELETE", recordedRequest.getMethod());
mockWebServer.close();
}
}
// just an example class to use as a payload
class Hello {
String text;
public Hello() {
}
public Hello(String text) {
this.text = text;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
}
筆記。 即使您不會選擇第一個解決方案,我建議您放棄為每個請求初始化 RestTemplate。 你最好改用 WebClient 。 如果這樣做,第一個解決方案將不再有效,而第二個解決方案將保持不變。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.