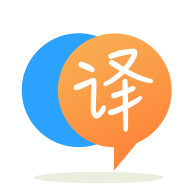
[英]Deref a Arc<RwLock<T>> to return RwLockReadGuard<T> by default
[英]Return a reference to a T inside a lazy static RwLock<Option<T>>?
我有一個懶惰的 static 結構,我希望能夠在程序執行開始時將其設置為某個隨機值,然后再獲取。 這個愚蠢的小片段可以用作示例:
use lazy_static::lazy_static;
use std::sync::RwLock;
struct Answer(i8);
lazy_static! {
static ref ANSWER: RwLock<Option<Answer>> = RwLock::new(None);
}
fn answer_question() {
*ANSWER.write().unwrap() = Some(Answer(42));
}
fn what_is_the_answer() -> &'static Answer {
ANSWER
.read()
.unwrap()
.as_ref()
.unwrap()
}
此代碼無法編譯:
error[E0515]: cannot return value referencing temporary value
--> src/lib.rs:15:5
|
15 | ANSWER
| _____^
| |_____|
| ||
16 | || .read()
17 | || .unwrap()
| ||_________________- temporary value created here
18 | | .as_ref()
19 | | .unwrap()
| |__________________^ returns a value referencing data owned by the current function
我知道您不能返回對臨時值的引用。 但我想返回對ANSWER
的引用,即 static - 與臨時相反! 我猜這是第一次調用RwLockReadGuard
返回的unwrap
問題?
我可以通過更改返回類型來編譯代碼:
fn what_is_the_answer() -> RwLockReadGuard<'static, Option<Answer>> {
ANSWER
.read()
.unwrap()
}
但是現在調用代碼變得非常不符合人體工程學 - 我必須進行兩次額外調用才能獲得實際值:
what_is_the_answer().as_ref().unwrap()
我可以從這個 function 以某種方式返回對 static ANSWER
的引用嗎? 我可以通過某種方式映射它來返回RwLockReadGuard<&Answer>
嗎?
once_cell
就是為此而設計的:在 answer_question 中使用.set(...).unwrap()
,在answer_question
中使用.get().unwrap()
what_is_the_answer
() 。
據我了解您的意圖, Answer
的值在lazy_static
中初始化時無法計算,但取決於僅在調用answer_question
時才知道的參數。 以下可能不是最優雅的解決方案,但它允許對一個值進行&'static
引用,該值取決於僅在運行時知道的參數。
基本方法是使用兩個lazy_static
,其中一個用作“代理”來進行必要的同步,另一個是值本身。 這避免了每次訪問ANSWER
時都必須訪問多層鎖和Option
值的展開。
ANSWER
值是通過等待CondVar
來初始化的,它會在計算出值時發出信號。 然后將該值放置在lazy_static
中,從那時起不可移動。 因此&'static
是可能的(參見get_the_answer()
)。 我選擇了String
作為示例類型。 請注意,在不調用generate_the_answer()
的情況下訪問ANSWER
將導致初始化永遠等待,從而使程序死鎖。
use std::{sync, thread};
lazy_static::lazy_static! {
// A proxy to synchronize when the value is generated
static ref ANSWER_PROXY: (sync::Mutex<Option<String>>, sync::Condvar) = {
(sync::Mutex::new(None), sync::Condvar::new())
};
// The actual value, which is initialized from the proxy and stays in place
// forever, hence allowing &'static access
static ref ANSWER: String = {
let (lock, cvar) = &*ANSWER_PROXY;
let mut answer = lock.lock().unwrap();
loop {
// As long as the proxy is None, the answer has not been generated
match answer.take() {
None => answer = cvar.wait(answer).unwrap(),
Some(answer) => return answer,
}
}
};
}
// Generate the answer and place it in the proxy. The `param` is just here
// to demonstrate we can move owned values into the proxy
fn generate_the_answer(param: String) {
// We don't need a thread here, yet we can
thread::spawn(move || {
println!("Generating the answer...");
let mut s = String::from("Hello, ");
s.push_str(¶m);
thread::sleep(std::time::Duration::from_secs(1));
let (lock, cvar) = &*ANSWER_PROXY;
*lock.lock().unwrap() = Some(s);
cvar.notify_one();
println!("Answer generated.");
});
}
// Nothing to see here, except that we have a &'static reference to the answer
fn get_the_answer() -> &'static str {
println!("Asking for the answer...");
&ANSWER
}
fn main() {
println!("Hello, world!");
// Accessing `ANSWER` without generating it will deadlock!
//get_the_answer();
generate_the_answer(String::from("John!"));
println!("The answer is \"{}\"", get_the_answer());
// The second time a value is generated, noone is listening.
// This is the flipside of `ANSWER` being a &'static
generate_the_answer(String::from("Peter!"));
println!("The answer is still \"{}\"", get_the_answer());
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.