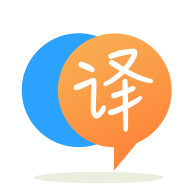
[英]Why do I keep getting a line 93 error saying TwitterClient not defined when it is defined in a class above?
[英]i keep getting the error saying that my class name is not defined in python
由於這個錯誤,我在我的學校計划中被困了一個多小時,我似乎無法弄清楚如何解決它。
導入系統
我的日期類:
#Constructer
def __init__(self, month, day, year):
self.day = day #Privet day
self.month = month #Privet month
self.year = year #privet year
#format for MDYYYY
def MDYYYY(self):
print("%i/%i/%i" %(myDate.month, myDate.day, myDate.year))
#format for MDYY
#format for YYYYMD
#mutator to set Day of the month
def setDay(self, a):
try:
day = int(a)
if day < 1 or day > 31:
raise Exception("invalid day")
except Exception as err:
print("Invalid day")
sys.exit( err )
else:
self.__day = day
#Mutator to set Month of the year
def setMonth(self, a):
try:
month = int(a)
if month < 1 or month > 12:
raise Exception("invalid month")
except Exception as err:
print("Invalid month")
sys.exit( err )
else:
self.__month = month
#Mutator to set the year of the date
def setYear(self, a):
try:
day = int(a)
if day < 1700:
raise Exception("invalid year")
except Exception as err:
print("Invalid year")
sys.exit( err )
else:
self.__year = year
# accessor to get day
def getDay(self):
return self.__day
# accessor to get the month
def getMonth(self):
return self.__month
# accessor to get the year
def getYear(self):
return self.__year
if __name__ == "__main__":
print()
print( "Instantiating object today and sorting value using .setMonth(6) .setDay(17) .setYear(2019)" )
setDay.day = 17
setMonth.month = 6
setYear.year = 2019
print(myDate.MDYYYY())
我在運行時得到這個結果:今天實例化對象並使用 .setMonth(6) .setDay(17) .setYear(2019) 回溯(最近一次調用):文件“D:\\RCCC\\Fall 2020\\Python” GUI\\Project 1\\dateformat_duxbury.py", line 8, in class myDate(): File "D:\\RCCC\\Fall 2020\\Python GUI\\Project 1\\dateformat_duxbury.py", line 80, in myDate print(myDate.MDYYYY.py) ()) NameError: name 'myDate' 未定義
從您帖子的格式來看,您的方法定義似乎與您對 myDate 類的聲明處於同一縮進級別。 這意味着它們不是該類的方法,而是全局空間的方法。 您應該將所有這些縮進,以便它們比類 myDate 的聲明更深一層。 這解釋了“名稱 'myDate' 未定義”錯誤。
還有幾個錯誤:
例子:
date = myDate()
date.setDay(17)
date.setMonth(6)
date.setYear(2019)
不要誤會,但聽起來您應該查看有關類如何在 Python 中工作的教程。
歡迎使用堆棧溢出。
您的問題似乎更像是一個概念上的問題,而不是實現上的問題。 我強烈建議您閱讀面向對象的原則,因為您似乎混淆了類和對象。
例如,您的具體錯誤是參考了這部分代碼:
#format for MDYYYY
def MDYYYY(self):
print("%i/%i/%i" %(myDate.month, myDate.day, myDate.year))
但是,您是否考慮過該函數中是否有 myDate 的任何實例? 實際上,您嘗試訪問的是您將從中調用 MDYYYY() 的特定對象的月、日和年。 在這種情況下,您需要訪問對象自己的月、日和年變量。 為此,您可以使用self
關鍵字訪問對象的內部變量。
#format for MDYYYY
def MDYYYY(self):
print("%i/%i/%i" %(self.month, self.day, self.year))
現在,第二個問題是在主代碼塊中實例化一個對象。
if __name__ == "__main__":
print()
print( "Instantiating object today and sorting value using .setMonth(6) .setDay(17) .setYear(2019)" )
# Instantiate a myDate object here:
myDateObject = myDate(4, 25, 2005) # You need to pass in the month, day and year values as part of your class init function
# You call instance functions on the object itself, such as
myDateObject.setDay(7)
myDateObject.setMonth(6)
myDateObject.setYear(2019)
# Call the MDYYYY function on the myDateObject instance and not the myDate class
print(myDateObject.MDYYYY())
同樣,我強烈建議您復習一些 OOP 基礎知識。 希望這會有所幫助。
目前尚不清楚為什么在您的main
部分中您訪問類方法而不先實例化類。 你的評論說你正在實例化這個類,但你真的不是。 它應該是這樣的:
if __name__ == "__main__":
md = myDate(1, 1, 1900)
md.setDay(17)
md.setMonth(6)
md.setYear(2019)
好的,那么MDYYYY()
方法以奇怪的方式定義。 這是myDate
類的一個方法,但出於某種原因,您指的是該類,而不是簡單地使用self
:
def MDYYYY(self):
print("%i/%i/%i" %(myDate.month, myDate.day, myDate.year))
#Should probably be defined instead like
def MDYYYY(self):
print(f'{self.month}/{self.day}/{self.year}')
此外,您有縮進問題,並且您的類/方法名稱不是 Pythonic(請參閱PEP 8 )。
因此,如果其他人遇到此問題,這里是我用來解決問題的最終代碼,感謝評論並幫助我的人。 另外我不知道為什么它會在代碼中顯示導入和類,但所有功能都被標記了。
`導入系統
我的日期類:
#Constructer
def __init__(self, month, day, year):
self.day = day
self.month = month
self.year = year
#format for MDYYYY
def MDYYYY():
print(f'{myDate.month}/{myDate.day}/{myDate.year}')
#format for MDYY
def MDYY():
print(f'{myDate.month}/{myDate.day}/{myDate.year}')
#format for YYYYMD
def YYYYMD():
print(f'{myDate.year}/{myDate.month}/{myDate.day}')
#mutator to set Day of the month
def setDay(self, a):
try:
day = int(a)
if day < 1 or day > 31:
raise Exception("invalid day")
except Exception as err:
print("Invalid day")
sys.exit( err )
else:
self.__day = day
#Mutator to set Month of the year
def setMonth(self, a):
try:
month = int(a)
if month < 1 or month > 12:
raise Exception("invalid month")
except Exception as err:
print("Invalid month")
sys.exit( err )
else:
self.__month = month
#Mutator to set the year of the date
def setYear(self, a):
try:
day = int(a)
if day < 1700:
raise Exception("invalid year")
except Exception as err:
print("Invalid year")
sys.exit( err )
else:
self.__year = year
# accessor to get day
def getDay(self):
return self.__day
# accessor to get the month
def getMonth(self):
return self.__month
# accessor to get the year
def getYear(self):
return self.__year
if name ==" main ": print() print( "今天實例化對象並使用 .setMonth(6) .setDay(17) .setYear(2019)" ) myDate.day = 17 myDate.month= 6 myDate.年 = 2019
# Print the date format for MM/DD/YYYY (01/01/2001)
print('Showing date in format- MM/DD/YYYY: ', end = '')
print(myDate.MDYYYY())
print("Instantiating object today and sorting value using .setMonth(7) .setDay(18) .setYear(2021)")
myDate.day = 18
myDate.month= 7
myDate.year = 2021
if myDate.year > 1699 and myDate.year < 1800:
myDate.year = myDate.year - 1700
elif myDate.year > 1799 and myDate.year < 1900:
myDate.year = myDate.year - 1800
elif myDate.year > 1899 and myDate.year < 2000:
myDate.year = myDate.year - 1900
elif myDate.year > 1999 and myDate.year < 2100:
myDate.year = myDate.year - 2000
# Print the date fomat for MM/DD/YY (01/01/01)
print('Showing date format - MM/DD/YY: ', end = '')
print(myDate.MDYY())
myDate.year = 2021
# Prints the date format for YYYY/MM/DD (2001/01/01)
print('Showing date format - YYYY/MM/DD: ', end ='')
print(myDate.YYYYMD())
print()`
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.