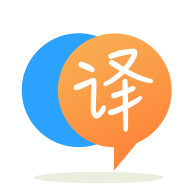
[英]How to increase array size during runtime to avoid garbage value at the end
[英]How to dynamically increase size of any structure objects during runtime?
我是 C 語言的初學者。 我正在嘗試在輸入通過時動態重新分配內存的任務(雖然對於這個測試我正在做一個正常的任務,稍后會嘗試擴大它)。 我面臨的問題是在寫入內存時無法訪問內存。 任何人都可以幫助我理解我在這段代碼中哪里出錯了。 提前致謝。
代碼:
#include<stdio.h>
#include<stdlib.h>
struct ai {
int* a;
char* b;
};
int main()
{
struct ai *test;
test = (struct ai*) malloc(sizeof(struct ai));
test[0].a = (int*)malloc(sizeof(int));
test[0].b = (char*)malloc(sizeof(char));
/// storing info
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 4; j++)
{
test[i].a[j] = j;
test[i].b[j] = 65 + j;
test[i].a = (int *) realloc(test[i].a, (j + 2) * sizeof(int));
test[i].b = (char*) realloc(test[i].b, (j + 2) * sizeof(char));
}
test = (struct ai*)realloc(test, (i + 2) * sizeof(struct ai));
}
// printing the block out
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 4; j++)
{
printf("%d , %c\n", test[i].a[j], test[i].b[j]);
}
}
return 0;
} ```
您缺少指針的初始化和其他一些問題...
int main()
{
struct ai *test;
test = (struct ai*) malloc(sizeof(struct ai));
test[0].a = (int*)malloc(sizeof(int));
test[0].b = (char*)malloc(sizeof(char));
在 C 中,您不應malloc
的結果。 在最好的情況下它是無用的。 在最壞的情況下,它隱藏了真正的問題。
/// storing info
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 4; j++)
{
test[i].a[j] = j;
test[i].b[j] = 65 + j;
這是您的分段錯誤的原因:除了第一個元素test[0]
之外,您沒有為a
和b
保留任何內存。 所有其他數組元素test[i]
不包含任何有用的數據。 取消引用這些指針是未定義的行為。
擴大數組后,您必須為每個字段分配內存。
test[i].a = (int *) realloc(test[i].a, (j + 2) * sizeof(int));
test[i].b = (char*) realloc(test[i].b, (j + 2) * sizeof(char));
永遠不要將realloc
的返回值分配給傳遞給函數的同一個變量。 如果出現錯誤,您將獲得NULL
返回值,然后您的初始指針丟失。
也在這里:不要為realloc
投射。
}
test = (struct ai*)realloc(test, (i + 2) * sizeof(struct ai));
此處相同:不要分配給相同的變量,也不要realloc
的結果。
最后:這是缺失的部分:
test[i+1].a = malloc(sizeof(*test[0].a));
test[i+1].b = malloc(sizeof(*test[0].b));
}
當然,您應該檢查所有返回值的錯誤結果。
與分段錯誤無關,但仍然值得修復:在使用最后一個元素后增加每個數組的大小。 這為在循環的下一次迭代中填充新元素做好了准備。 但是在最后一次迭代的情況下,它為內部指針a
和b
以及外部數組test
分配了一個未使用的元素。
您將有一些未使用的元素:
test[0].a[4]
test[0].b[4]
test[1].a[4]
test[1].b[4]
test[2].a[0]
test[2].b[0]
改進的解決方案可能如下所示(未經測試)
我從每個指針都為NULL
開始,並在使用新元素之前使用realloc
以避免額外的元素。
int main(void)
{
struct ai *test = NULL
void *tmp;
// storing info
for (int i = 0; i < 2; i++)
{
tmp = realloc(test, (i+1) * sizeof(*test))
if (tmp == NULL)
exit(1);
test = tmp;
test[i].a = NULL;
test[i].b = NULL;
for (int j = 0; j < 4; j++)
{
tmp = realloc(test[i].a, (j+1) * sizeof(test[i].a[0]));
if (tmp == NULL)
exit(1);
test[i].a = tmp;
tmp = realloc(test[i].b, (j+1) * sizeof(test[i].b[0]));
if (tmp == NULL)
exit(1);
test[i].b = tmp;
test[i].a[j] = j;
test[i].b[j] = 65 + j;
}
}
// printing the block out
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 4; j++)
{
printf("%d , %c\n", test[i].a[j], test[i].b[j]);
}
}
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.