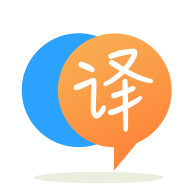
[英]Disable button click until all input fields have text in them (javascript & jquery)
[英]Javascript Disable button until 3 or more input fields have a value
我的 html 頁面中有 6 個輸入字段,如下所示:
<div class='inputBox'>
<form action="/" method="post">
<div>
<label>Angle of A = </label>
<input class='field' autocomplete="off" autofocus name="A" type="number">
</div>
<div>
<label>Angle of B = </label>
<input class='field' autocomplete="off" autofocus name="B" type="text">
</div>
<div>
<label>Angle of C = </label>
<input class='field' autocomplete="off" autofocus name="C" type="text">
</div>
<div>
<label>Side Length of a = </label>
<input class='field' autocomplete="off" autofocus name="a" type="text">
</div>
<div>
<label>Side Length of b = </label>
<input class='field' autocomplete="off" autofocus name="b" type="text">
</div>
<div>
<label>Side Length of c = </label>
<input class='field' autocomplete="off" autofocus name="c" type="text">
</div>
<div>
<button id='calculate' disabled="true" class='field' type="submit">Calculate</button>
</div>
</form>
</div>
我希望按鈕被禁用,直到 6 個字段中的任何 3 個具有值。 這是我的 javascript 代碼:
function enableButton()
{
var filled = 0;
var fields = [...document.getElementsByClassName("field")];
for (var i = 0; i < 6; i++)
{
if (fields[i].length() > 0)
{
filled += 1;
}
}
if (filled >= 3)
{
document.getElementById("calculate").disabled = false;
}
else
{
document.getElementById("calculate").disabled = true;
}
}
不幸的是,盡管具有值的字段數量很多,但按鈕仍處於禁用狀態。
嘗試對輸入字段使用更改事件,我使用onkeyup
函數來調用enableButton
函數。 而不是檢查fields[i].length()
嘗試檢查fields[i].value
是否存在。 這是一個工作示例。
function enableButton() { var filled = 0; var fields = [...document.getElementsByClassName("field")]; for (var i = 0; i < 6; i++) { if (fields[i].value) { filled += 1; } } if (filled >= 3) { document.getElementById("calculate").disabled = false; } else { document.getElementById("calculate").disabled = true; } }
<div class='inputBox'> <form action="/" method="post"> <div> <label>Angle of A = </label> <input class='field' autocomplete="off" autofocus name="A" type="number" onkeyup="enableButton()"> </div> <div> <label>Angle of B = </label> <input class='field' autocomplete="off" autofocus name="B" type="text" onkeyup="enableButton()"> </div> <div> <label>Angle of C = </label> <input class='field' autocomplete="off" autofocus name="C" type="text" onkeyup="enableButton()"> </div> <div> <label>Side Length of a = </label> <input class='field' autocomplete="off" autofocus name="a" type="text" onkeyup="enableButton()"> </div> <div> <label>Side Length of b = </label> <input class='field' autocomplete="off" autofocus name="b" type="text" onkeyup="enableButton()"> </div> <div> <label>Side Length of c = </label> <input class='field' autocomplete="off" autofocus name="c" type="text" onkeyup="enableButton()"> </div> <div> <button id='calculate' disabled="true" class='field' type="submit">Calculate</button> </div> </form> </div>
那 ?
我只是在form元素上加了一個id,把按鈕的Id屬性改成了name,這樣我就只有for聲明為const了
並刪除表單中所有不必要的div,輕量級代碼總是更容易閱讀
const myForm = document.getElementById('my-form') , inFields = [...myForm.querySelectorAll('input.field')] ; myForm.oninput=()=> { let count = inFields.reduce((a,c)=>a+=(c.value.trim().length >0)?1:0,0) myForm.calculate.disabled = (count <3) } myForm.onreset=()=> { myForm.calculate.disabled = true }
label, input, button { display: block; float: left; margin: .3em; } label, button:first-of-type { clear: both; } label { width: 10em; line-height: 2.3em; text-align: right; }
<form action="/" method="post" id="my-form"> <label>Angle of A = </label> <input class='field' autocomplete="off" autofocus name="A" type="number"> <label>Angle of B = </label> <input class='field' autocomplete="off" autofocus name="B" type="text"> <label>Angle of C = </label> <input class='field' autocomplete="off" autofocus name="C" type="text"> <label>Side Length of a = </label> <input class='field' autocomplete="off" autofocus name="a" type="text"> <label>Side Length of b = </label> <input class='field' autocomplete="off" autofocus name="b" type="text"> <label>Side Length of c = </label> <input class='field' autocomplete="off" autofocus name="c" type="text"> <button type="reset">reset</button> <button name='calculate' disabled="true" class='field' type="submit">Calculate</button> </form>
您可能在使用該函數時遇到了一些問題,但主要問題是您從未調用enableButton
函數。 只要其中一個字段發生更改,下面的代碼就會調用它。
function enableButton() { let filled = 0; let fields = document.getElementsByClassName("field"); for (let i = 0; i < fields.length; i++) { if (fields[i].value != "") { filled++; } } if (filled > 2) { document.getElementById("calculate").removeAttribute("disabled"); } else { document.getElementById("calculate").setAttribute("disabled", ""); } }
<div class='inputBox'> <form action="/" method="post"> <div> <label>Angle of A = </label> <input class='field' autocomplete="off" autofocus name="A" type="number" onchange="enableButton()"> </div> <div> <label>Angle of B = </label> <input class='field' autocomplete="off" autofocus name="B" type="text" onchange="enableButton()"> </div> <div> <label>Angle of C = </label> <input class='field' autocomplete="off" autofocus name="C" type="text" onchange="enableButton()"> </div> <div> <label>Side Length of a = </label> <input class='field' autocomplete="off" autofocus name="a" type="text" onchange="enableButton()"> </div> <div> <label>Side Length of b = </label> <input class='field' autocomplete="off" autofocus name="b" type="text" onchange="enableButton()"> </div> <div> <label>Side Length of c = </label> <input class='field' autocomplete="off" autofocus name="c" type="text" onchange="enableButton()"> </div> <div> <button id='calculate' type="submit" disabled>Calculate</button> </div> </form> </div>
你是如何調用函數的,還放了一個控制台日志來檢查函數是否被調用。 也正如@certainPerformance 所說的 .length 是一個屬性而不是一個函數
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.