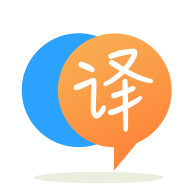
[英]I have written this code to convert an infix expression to a postfix expression using Stacks in CPP
[英]How do I convert an infix expression to a postfix expression and I am not able to get the expected output?
我正在嘗試編寫一個 C++ 程序來將中綴表達式轉換為前綴和后綴。 我哪里做錯了?
如何更改代碼以刪除最后一個表單(后綴)中的括號? 謝謝你的幫助!
輸入 :
a*(b-c+d)/e
輸出 :
abc-d+*e/
/*a+-bcde
約束/假設:
+
、 -
、 *
、 /
(
)
- 用於影響操作的優先級。+
和-
具有相同的優先級,小於*
和/
。 *
和/
也具有相同的優先級。#include <cstring>
#include <ctype.h>
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int precedence(char ch) {
if (ch == '+') {
return (1);
} else if (ch == '-') {
return (1);
} else {
return (2);
}
}
void preProcess(stack<string> &preS, char op) {
string val2 = preS.top();
preS.pop();
string val1 = preS.top();
preS.pop();
string prev;
prev = op + val1 + val2;
preS.push(prev);
}
void postProcess(stack<string> &postS, char op) {
string val2 = postS.top();
postS.pop();
string val1 = postS.top();
postS.pop();
string postv;
postv = val1 + val2 + op;
postS.push(postv);
}
void prefixPostfixEvaluation(string expr) {
stack<string> preS;
stack<string> postS;
stack<char> opS;
for (int i = 0; i < expr.length(); ++i) {
char ch = expr.at(i);
if (ch == '(') {
opS.push(ch);
} else if ((ch <= '0' && ch <= '9') || (ch >= 'a' && ch <= 'z') ||
(ch <= 'A' && ch >= 'Z')) {
string s;
s = ch;
preS.push(s);
postS.push(s);
} else if (ch == ')') {
while (opS.top() != '(') {
char op = opS.top();
preProcess(preS, op);
postProcess(postS, op);
opS.pop();
}
opS.pop();
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
while (opS.size() > 0 && precedence(ch) <= precedence(opS.top()) &&
opS.top() != '(') {
char op = opS.top();
preProcess(preS, op);
postProcess(postS, op);
opS.pop();
}
opS.push(ch);
}
}
while (opS.size() > 0) {
char op = opS.top();
opS.pop();
if (op == '(' || op == ')') {
continue;
} else {
preProcess(preS, op);
postProcess(postS, op);
}
}
cout << preS.top() << endl;
cout << postS.top();
}
int main() {
string expr;
getline(cin, expr);
prefixPostfixEvaluation(expr);
}
檢查字符是否為操作數的條件是錯誤的。 代替 :
(ch <= '0' && ch <= '9') || (ch >= 'a' && ch <= 'z') || (ch <= 'A' && ch >= 'Z')
它應該是
(ch >= '0' && ch <= '9') || (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')
更好的方法是在條件語句中直接使用isalnum(ch)
(您已經包含ctype
標頭)。
完整(更正)代碼可以在這里找到。
注意:更喜歡包含像<cctype>
這樣的頭文件的 C++ 變體,而不是<ctype.h>
。 此外,當包含<string>
時,不需要包含<cstring>
。 另外,請查看此線程: 為什么“使用命名空間 std;” 被認為是不好的做法?
您希望后綴版本出現在第 1 行,前綴版本出現在第 2 行。但在代碼中,您以相反的順序打印,如果順序對您很重要,請更改它。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.