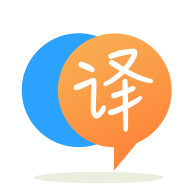
[英]How to create a new layer of sublists based on a common key within each sublist in order to categorize the sublists?
[英]How to sort sublists based on an object within each sublist
我有一個列表列表。 每個列表包含一個描述詞、一個詞或句子,然后是一個排序列表。
eg_list = [['sent', 'this is a sentence', [6, 1, 3]]
['word', 'abú', [5, 2, 9.5]]
['word', 'because', [5, 2, 11]]
['sent', 'this is not just one word', [6, 1, 1]]
['word', 'ol', [5, 2, 8]]
['word', 'magisteriums', [5, 1, 14]]]
我想根據每個子列表末尾的排序列表中的數字對子列表進行排序。 我需要它主要按排序列表中的第一個排序,然后是中間的數字,然后是最后一個,這樣排序的結果應該是這樣的:
['word', 'magisteriums', [5, 1, 14]]
['word', 'ol', [5, 2, 8]]
['word', 'abú', [5, 2, 9.5]]
['word', 'because', [5, 2, 11]]
['sent', 'this is not just one word', [6, 1, 1]]
['sent', 'this is a sentence', [6, 1, 3]]
我可以在 Python sorted()
函數中放入什么樣的鍵以這種方式進行排序?
因為您正在將排序列表與其元素從左到右進行比較,這是 python 在列表之間進行比較的普通順序,所以您需要做的就是提供一個關鍵函數來提取排序列表本身——這可以完成使用如下所示的lambda
(盡管operator.itemgetter(2)
將是一個替代方案)。 無需顯式引用排序列表中的元素。
為了提取排序列表,下面的示例在 lambda(即第三個元素)中使用lst[2]
,但它也可以使用lst[-1]
(即最后一個元素)。
from pprint import pprint # pprint used for output display only
eg_list = [['sent', 'this is a sentence', [6, 1, 3]],
['word', 'abú', [5, 2, 9.5]],
['word', 'because', [5, 2, 11]],
['sent', 'this is not just one word', [6, 1, 1]],
['word', 'ol', [5, 2, 8]],
['word', 'magisteriums', [5, 1, 14]]]
pprint(sorted(eg_list, key=lambda lst:lst[2]))
這給出:
[['word', 'magisteriums', [5, 1, 14]],
['word', 'ol', [5, 2, 8]],
['word', 'abú', [5, 2, 9.5]],
['word', 'because', [5, 2, 11]],
['sent', 'this is not just one word', [6, 1, 1]],
['sent', 'this is a sentence', [6, 1, 3]]]
更新- 您還詢問(在評論中)如果您想以不同的順序使用排序列表的元素,您會怎么做。 在這種情況下,您需要做的是編寫一個關鍵函數,以按所需順序構建一個新的排序列表或元組。 盡管可以將其壓縮為單行,但為它編寫顯式函數可能更方便。 例如,要按中、左、右順序排序,您可以使用:
def get_key(lst):
o_lst = lst[2]
# let's return a tuple, although list would also work
return (o_lst[1], o_lst[0], o_lst[2])
然后,您將使用key=get_key
進行排序。
等效的單行將使用:
key=lambda lst:(lst[2][1], lst[2][0], lst[2][2])
但在我看來,重復lst[2]
使得這不太可取。
無論哪種情況,您都會得到:
[['word', 'magisteriums', [5, 1, 14]],
['sent', 'this is not just one word', [6, 1, 1]],
['sent', 'this is a sentence', [6, 1, 3]],
['word', 'ol', [5, 2, 8]],
['word', 'abú', [5, 2, 9.5]],
['word', 'because', [5, 2, 11]]]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.