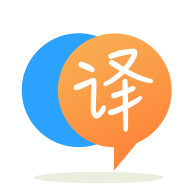
[英]HTML/Javascript - How to display text from input field as HTML code?
[英]How to display an image from input text in HTML JavaScript?
當用戶在文本字段中輸入“顯示鴨子圖像”並單擊“執行命令”按鈕時,我需要在我的網頁上顯示鴨子的圖片。
文本字段有許多命令,因此有“else if”語句。 這是我到目前為止所擁有的,當我試用duck 命令時,沒有顯示任何圖像。 任何幫助將不勝感激!
function theCommand(imageFile) { var commandInput = document.getElementById("commandInput"); var command = commandInput.value; var image = document.getElementById("imgResult"); image.src = imageFile; if (command == "make duck noise") { command = "QUACK QUACK"; } else if (command == "make rooster noise") { command = "COCK-A-DOODLE-DOO"; } else if (command == "show duck image") { command == imageFile; } else if (command != "make duck noise" || command != "make rooster noise") { command = "Invalid command"; } var commandSpan = document.getElementById("result"); commandSpan.innerHTML = command; }
<html> <body> Enter command: <input type="text" id="commandInput" /> <button onClick="theCommand('duck.png')">Execute command</button> <br /> <br /> <span id="result"></span> <img id="imgResult"/> </body> </html>
將 IMG 元素添加到您的命令幫助中。
function theCommand(imageFile) { var commandInput = document.getElementById("commandInput"); var command = commandInput.value; var image = document.getElementById("result"); image.src = imageFile; if (command == "make duck noise") { command = "QUACK QUACK"; } else if (command == "make rooster noise") { command = "COCK-A-DOODLE-DOO"; } else if (command == "show duck image") { command = "<img src='https://www.checkeins.de/sendungen/die-sendung-mit-der-maus/die-sendung-mit-dermaus-ente-100~_v-standard644_5fdf7b.jpg'></img>"; } else if (command != "make duck noise" || command != "make rooster noise") { command = "Invalid command"; } var commandSpan = document.getElementById("result"); commandSpan.innerHTML = command; }
<html> <body> Enter command: <input type="text" id="commandInput" /> <button onClick="theCommand('duck.png')">Execute command</button> <br /> <br /> <span id="result"></span> </body> </html>
您應該使用<img>
元素來顯示圖像。
<img id="imgResult"/>
var image = document.getElementById("imgResult");
image.src = imageFile;
您需要使用 HTML <img>
標記作為放入結果中的 HTML。 其他command
值是純文本,用作.innerHTML
。 為了正確顯示圖像,我們需要一個完整的<img src="duck.png">
字符串。
為了演示,我更改了 HTML 片段以引用公共鴨子圖像。
function theCommand(imageFile) { var commandInput = document.getElementById("commandInput"); // By default, set the result value to the form input's value (a string of text) var command = commandInput.value; if (command == "make duck noise") { command = "QUACK QUACK"; } else if (command == "make rooster noise") { command = "COCK-A-DOODLE-DOO"; } else if (command == "show duck image") { // For the image case, set result to be an HTML <img> element with src value passed in command = '<img src="' + imageFile + '">'; } else if (command != "make duck noise" || command != "make rooster noise") { command = "Invalid command"; } var commandSpan = document.getElementById("result"); // When command is "show duck image", `command` var will have a functional <img> tag in it // instead of plain text commandSpan.innerHTML = command; }
<html> <body> Enter command: <input type="text" id="commandInput" /> <button onClick="theCommand('https://images.unsplash.com/photo-1459682687441-7761439a709d?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&w=1000&q=80')">Execute command</button> <br /> <br /> <span id="result"></span> </body> </html>
條件語句的邏輯似乎可能無法按預期工作。 對else if (command != "make duck noise" || command != "make rooster noise") {
檢查是多余的,因為這些字符串已經在條件語句的更高層進行了檢查。 它可以被重構為簡單地使用else
作為一個包羅萬象的:
if (command == "make duck noise") {
command = "QUACK QUACK";
} else if (command == "make rooster noise") {
command = "COCK-A-DOODLE-DOO";
} else if (command == "show duck image") {
// For the image case, set result to be an HTML <img> element with src value passed in
command = '<img src="' + imageFile + '">';
} else {
command = "Invalid command";
}
當條件邏輯最終需要像這樣的大量特定情況( else if
s)時,進行一些重構可以使代碼更容易使用。 我強烈建議查看在 JavaScript 中編寫更好條件的 5 個技巧——Scotch.io 。 “偏好映射/對象文字而不是開關語句https://scotch.io/bar-talk/5-tips-to-write-better-conditionals-in-javascript#toc-4-favor-map-object-literal”部分-than-switch-statement”對您的示例特別有用:
function theCommand(imageFile) { var commandInput = document.getElementById("commandInput"); // By default, set the result value to the form input's value (a string of text) var command = commandInput.value; var commandMap = { 'make duck noise': "QUACK QUACK", 'make rooster noise': "COCK-A-DOODLE-DOO", 'show duck image': '<img src="' + imageFile + '">', } var commandSpan = document.getElementById("result"); // Set inner HTML of result element to the value returned by the command map // or use a default string if the command given is not in the map commandSpan.innerHTML = commandMap[command] || 'Invalid command'; }
<html> <body> Enter command: <input type="text" id="commandInput" /> <button onClick="theCommand('https://images.unsplash.com/photo-1459682687441-7761439a709d?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&w=1000&q=80')">Execute command</button> <br /> <br /> <span id="result"></span> </body> </html>
上面的代碼還有一個額外的好處,即不會改變原始command
變量,因此原始用戶輸入值在程序執行過程中永遠不會丟失。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.