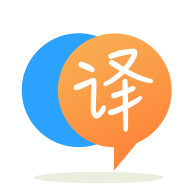
[英]Get current value of another widget in a custom (promoted) widget in Qt Designer using PyQt5
[英]Detect reload of PyQt5 script, due to promoted widget in QtDesigner?
很多時候,我需要在 QtDesigner 設計的 PyQt5 GUI 中完成一些任務,這些任務足夠小,我想將所有代碼保存在一個 .py 文件中。 因此,請考慮這個 PyQt5 示例:
test3.py
:
import sys
from PyQt5 import QtCore, QtWidgets, QtGui, uic
mod_name = vars(sys.modules[__name__])['__package__'] # SO:1389044
code_exec_as = 'module named {}'.format(mod_name) if mod_name else 'script'
cmdline = "{} {}".format( sys.executable, " ".join(sys.argv) )
try: # SO:6038898
reloading
except NameError:
reloading = False # means the module is being imported
else:
reloading = True # means the module is being reloaded
print("Starting: code executed as {}; reloading {}; command line '{}'".format(code_exec_as, reloading, cmdline))
class MyCustomButton(QtWidgets.QPushButton):
def __init__(self, parent=None):
super().__init__(parent)
print("MyCustomButton init!")
class MyMainWindow(QtWidgets.QMainWindow):
def __init__(self):
super(MyMainWindow, self).__init__()
uic.loadUi("test3.ui", self)
self.show()
def main():
app = QtWidgets.QApplication(sys.argv)
window = MyMainWindow()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
test3.ui
:
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>MainWindow</class>
<widget class="QMainWindow" name="MainWindow">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>382</width>
<height>232</height>
</rect>
</property>
<property name="windowTitle">
<string>MainWindow</string>
</property>
<widget class="QWidget" name="centralwidget">
<layout class="QHBoxLayout" name="horizontalLayout">
<item>
<widget class="QPushButton" name="pushButton">
<property name="text">
<string>PushButton</string>
</property>
</widget>
</item>
<item>
<widget class="MyCustomButton" name="pushButton_2">
<property name="text">
<string>CustomPushButton</string>
</property>
</widget>
</item>
</layout>
</widget>
<widget class="QMenuBar" name="menubar">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>382</width>
<height>21</height>
</rect>
</property>
</widget>
<widget class="QStatusBar" name="statusbar"/>
</widget>
<customwidgets>
<customwidget>
<class>MyCustomButton</class>
<extends>QPushButton</extends>
<header>test3</header>
</customwidget>
</customwidgets>
<resources/>
<connections/>
</ui>
當我從命令行運行它時,我得到:
$ python3 test3.py
Starting: code executed as script; reloading False; command line 'C:/msys64/mingw64/bin/python3.exe test3.py'
Starting: code executed as script; reloading False; command line 'C:/msys64/mingw64/bin/python3.exe test3.py'
MyCustomButton init!
請注意,“開始:...”打印輸出已運行兩次:顯然:
.ui
中的pushButton_2
被提升為MyCustomButton
類,該類被列為在test3.py
中定義,因此需要重新加載該文件,以便讀取MyCustomButton
類定義我能否從腳本內部(更具體地說,從導入之后的代碼部分,但在任何類定義和__main__
調用之前)以某種方式檢測腳本正在運行的時間?
正如你所看到的,我已經在test3.py
嘗試了一些東西,基於:
...但是這些方法為腳本的兩次“運行”提供了相同的輸出,因此我無法使用它們來區分腳本是在第一次運行還是第二次運行中。
好的,我想我明白了:看看如何列出導入的模塊? - 然后打印模塊並進行比較:結果是,腳本的第一次運行, sys.modules
模塊較少 - 更具體地說,在腳本的第二次運行中,第一次運行中不存在類,最重要的是PyQt5.uic.Loader
出現在第二次運行中,並且test3
(Python 腳本本身的名稱/類,在 QtDesigner 文件中使用)也存在。 因此,可以使用這兩個類中的任何一個來區分。
因此,我將腳本更改為:
import sys
from PyQt5 import QtCore, QtWidgets, QtGui, uic
mod_name = vars(sys.modules[__name__])['__package__'] # SO:1389044
code_exec_as = 'module named {}'.format(mod_name) if mod_name else 'script'
cmdline = "{} {}".format( sys.executable, " ".join(sys.argv) )
try: # SO:6038898
reloading
except NameError:
reloading = False # means the module is being imported
else:
reloading = True # means the module is being reloaded
first_time_run = not( 'PyQt5.uic.Loader' in sys.modules.keys() )
print("Starting: code executed as {}; reloading {}; command line '{}'; num modules {}; first_time_run {}".format(code_exec_as, reloading, cmdline, len(sys.modules.keys()), first_time_run))
#print("..in sys.modules.keys():\n {}".format( "\n ".join( sorted(sys.modules.keys()) ) ))
class MyCustomButton(QtWidgets.QPushButton):
def __init__(self, parent=None):
super().__init__(parent)
print("MyCustomButton init!")
class MyMainWindow(QtWidgets.QMainWindow):
def __init__(self):
super(MyMainWindow, self).__init__()
uic.loadUi("test3.ui", self)
self.show()
def main():
app = QtWidgets.QApplication(sys.argv)
window = MyMainWindow()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
...現在打印輸出是:
$ python3 test3.py
Starting: code executed as script; reloading False; command line 'C:/msys64/mingw64/bin/python3.exe test3.py'; num modules 113; first_time_run True
Starting: code executed as script; reloading False; command line 'C:/msys64/mingw64/bin/python3.exe test3.py'; num modules 117; first_time_run False
MyCustomButton init!
...這向我證實,第一次運行被正確檢測到。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.