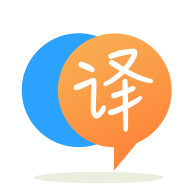
[英]How to properly pass array interface/type to a child component in TypeScript React?
[英]How to properly type a React ErrorBoundary class component in Typescript?
這是我目前關於如何在 Typescript 中正確鍵入 React ErrorBoundary
class 組件的嘗試:
import React from "react";
import ErrorPage from "./ErrorPage";
import type { Store } from "redux"; // I'M PASSING THE REDUX STORE AS A CUSTOM PROP
interface Props {
store: Store // THIS IS A CUSTOM PROP THAT I'M PASSING
}
interface State { // IS THIS THE CORRECT TYPE FOR THE state ?
hasError: boolean
}
class ErrorBoundary extends React.Component<Props,State> {
constructor(props: Props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error): State {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo: React.ErrorInfo): void {
// You can also log the error to an error reporting service
// logErrorToMyService(error, errorInfo);
console.log("error: " + error);
console.log("errorInfo: " + JSON.stringify(errorInfo));
console.log("componentStack: " + errorInfo.componentStack);
}
render(): React.ReactNode {
if (this.state.hasError) {
// You can render any custom fallback UI
return(
<ErrorPage
message={"Something went wrong..."}
/>
);
}
return this.props.children;
}
}
export default ErrorBoundary;
這個問題是關於這個ErrorBoundary
class 組件的類型的。 我將它分成幾部分以使其更容易。
A 部分:道具類型和 state
我做的對嗎?
interface Props {
store: Store // THIS IS A CUSTOM PROP THAT I'M PASSING
}
interface State { // IS THIS THE CORRECT TYPE FOR THE state ?
hasError: boolean
}
class ErrorBoundary extends React.Component<Props,State> {}
B 部分:getDerivedStateFromError(錯誤)
我應該為error
參數選擇什么類型? 返回類型應該是State
類型吧?
static getDerivedStateFromError(error): State {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
第 C 部分:componentDidCatch(錯誤,錯誤信息:React.React.ErrorInfo)
我應該為error
參數選擇什么類型? 對於errorInfo
,React 確實有一個似乎正確的ErrorInfo
類型。 是嗎? 返回類型應該是void
,對嗎?
componentDidCatch(error, errorInfo: React.ErrorInfo): void {
console.log("error: " + error);
console.log("errorInfo: " + JSON.stringify(errorInfo));
console.log("componentStack: " + errorInfo.componentStack);
}
D 部分:render() 方法
返回類型應該是ReactNode
嗎?
render(): React.ReactNode {
if (this.state.hasError) {
// You can render any custom fallback UI
return(
<ErrorPage
message={"Something went wrong..."}
/>
);
}
return this.props.children;
}
您可以使用以下代碼作為錯誤邊界:
import React, { Component, ErrorInfo, ReactNode } from "react";
interface Props {
children: ReactNode;
}
interface State {
hasError: boolean;
}
class ErrorBoundary extends Component<Props, State> {
public state: State = {
hasError: false
};
public static getDerivedStateFromError(_: Error): State {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
public componentDidCatch(error: Error, errorInfo: ErrorInfo) {
console.error("Uncaught error:", error, errorInfo);
}
public render() {
if (this.state.hasError) {
return <h1>Sorry.. there was an error</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
您將在來自@types/react
的文件index.d.ts
獲得所有答案。 如果你使用的是像 VSCode 這樣的 IDE,你可以在一個類型上按住 Ctrl 鍵並單擊以直接轉到它的定義。
這是您問題的准確答案,但在此之前,讓我建議您更喜歡使用 React hooks
而不是類。
OP 編輯:我從不使用類組件,總是更喜歡函數和鈎子,但來自關於錯誤邊界的 React文檔:
錯誤邊界的工作方式類似於 JavaScript catch {} 塊,但適用於組件。 只有類組件可以是錯誤邊界。
我給出的行是index.d.ts
版中 index.d.ts 中的行。
A 部分:是的,就是這樣做的。
B 部分:如您在第 658 行所見, error
類型為any
,返回類型為state
或null
。
C 部分:在第 641 行,您將看到錯誤類型為Error
並且返回類型為void
。
D 部分:在第 494 行,您可以看到 render 應該返回一個ReactNode
…
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.