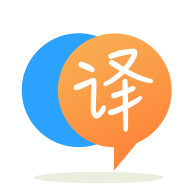
[英]How to render react element from a mapped array using object literal to get values
[英]How to operate on each individual rendered element when they are mapped from object array in React?
我正在嘗試制作一個重復同一張卡片的購物應用程序。 我沒有手動渲染它們,而是使用map
來渲染數組對象,如下所示:
父組件:
const Home = () => { const dummyData = [ { id: 1, title: tshirt, price: 10 }, { id: 2, title: hat, price: 20 } ] const [totalPrice, setTotalPrice] = useState(0); const itemNo = 2; const handleClick = (price) => { setTotalPrice(price * itemNo); } const RenderCards = () => { return ( dummyData.map( (d) => <Card key={d.id} title={d.title} price={d.price} totalPrice={totalPrice}/> ) ) } return( <> <RenderCards /> </> ) }
和子組件:
const Card = (id, title, price, totalPrice) => { return ( <> <div key={id}> <p>{title}</p> <p>{totalPrice}</p> <button onClick={() => handleClick(price)}>Click for total price</button> </div> </> ) }
單擊每張卡片上的按鈕時,我想顯示每張卡片的總價,即卡片 1 的總價格應為 10 * 2 = 20,卡片 2 的總價格應為 40。單擊卡片 1 應僅更改 {卡 1、卡 2 的 totalPrice} 不應受到影響,反之亦然。
但是,到目前為止,當單擊按鈕時,兩張卡都會顯示相同的總價。 我理解這種行為,因為將相同的數據傳遞給卡片組件,但是在這種情況下,當組件從數組映射呈現時,如何為每張卡片單獨設置數據?
const Home = () => {
const dummyData = [
{
id: 1,
title: tshirt,
price: 10
},
{
id: 2,
title: hat,
price: 20
}
]
const [totalPrice, setTotalPrice] = useState([]); //<-- an empty array for start
const handleClick = (id, qty) => {
let newState = [...totalPrice]; //<--- copy the state
if (newState.find(item => item.id === id) != undefined) { // find the item to add qty, if not exists, add one
newState.find(item => item.id === id).qty += qty
} else {
newState.push({id:id, qty:qty});
}
setTotalPrice(newState); //<-- set the new state
}
const RenderCards = () => {
return (
dummyData.map(
(d) => {
const stateItem = totalPrice.find(item=> item.id === d.id); // return the item or undefined
const qty = stateItem ? stateItem.qty : 0 // retreive qty from state by id or 0 if the product is not in the array
return (
<Card key={d.id} title={d.title} price={d.price} totalPrice={d.price * qty}/> //calculate the total
)
}
)
)
}
return(
<>
<RenderCards />
</>
)
}
和卡:
const Card = (id, title, price, totalPrice) => {
return (
<>
<div>
<p>{title}</p>
<p>{totalPrice}</p>
<button onClick={() => handleClick(id, 1)}>Click for add one</button> // add one, total price will be good on the next render
</div>
</>
)
}
也許有問題,但這個想法在這里
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.