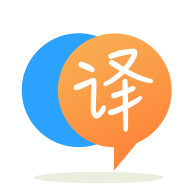
[英]How to return an array of character pointers from a function back to main?
[英]How to Return Values From thread Back to main()
我正在使用 pthread_create 創建一個線程,該線程檢查文件中的行數,然后將答案返回給主線程。 我曾嘗試使用 pthread_join 和 malloc(),但我對這兩者都不熟悉,一定是使用不當。 如果有人知道如何將整數從線程傳遞回主線程,請幫忙。 我的代碼如下。
#include <pthread.h>
#include <stdio.h>
void *count_lines(void *arg)
{
FILE *fh= (FILE *) arg;
int num_lines=0;
char ch;
for(ch=getc(fh); ch!=EOF; ch=getc(fh))
if(ch=='\n')
num_lines=num_lines+1;
fclose(fh);
int* value = (int *)malloc(sizeof(int));
*value=10;
pthread_exit(value);
}
int main()
{
FILE *fh;
fh=fopen("data.txt", "r");
pthread_t my_thread;
pthread_create(&my_thread, NULL, count_lines, &fh);
void *retval;
pthread_join(my_thread, &retval);
int i = *((int *)retval);
free(retval);
printf("%d\n", i);
}
如果有任何幫助,我正在運行 Ubuntu 虛擬機並使用 Visual Studio Code。 當我運行上面的代碼時,出現“核心轉儲(分段錯誤)”錯誤。 再次,非常感謝幫助。
你讓一切變得不必要地復雜。 制作一個像這樣的結構:
typedef struct
{
FILE* fp;
int ret_val;
} count_lines_type;
static count_lines_type cl;
cl.fp = fopen (...);
...
pthread_create(&my_thread, NULL, count_lines, &cl);
在線程完成之前填寫ret_val
。
我將結構實例static
,以防萬一調用線程在計數行線程完成之前超出范圍。 如果它從不這樣做,則不需要static
。
在創建線程之前,請檢查文件是否真的打開:
fh=fopen("data.txt", "r");
if (fh == NULL) exit(1);
另外 fh 已經是一個指針。 您不需要將 &fh (指向指針的指針)傳遞給 thred create(您在 count_lines 中期望 FILE* 而不是 FILE**)。 還要檢查線程創建是否成功:
if (pthread_create(&my_thread, NULL, count_lines, fh) != 0)
exit(2); //error -> contents of my_thread is undefined in this case
還要檢查 retval(僅當指針有效時才取消引用,否則會出現分段錯誤):
if (retval != NULL)
{
int i = *((int *)retval);
free(retval);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.