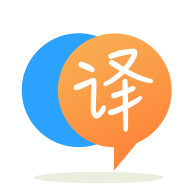
[英]Combine two nested dictionaries with same keys but different dictionaries as values
[英]Combine two same values with same keys in a list of dictionaries
完全是一個編程新手……需要一些幫助。
我所擁有的是以下格式的字典列表:
a_list = [
{'ID': 'a', 'Animal': 'dog', 'color': 'white', 'tail': 'yes'},
{'ID': 'a', 'Animal': 'cat', 'color': 'black', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'bird', 'color': 'black', 'tail': 'no'},
{'ID': 'b', 'Animal': 'cat', 'color': 'pink', 'tail': 'yes'}
{'ID': 'b', 'Animal': 'dog', 'color': 'yellow', 'tail': 'no'}
]
我要的是字典的字典如下:
a_dict =
{'a': {'dog': {'color': 'white', 'tail': 'yes'},
'cat': {'color': 'black', 'tail': 'yes'}},
'b': {'bird': {'color': 'black', 'tail': 'no'},
'cat': {'color': 'pink', 'tail': 'no'},
'dog': {'color': 'yellow', 'tail': 'no'}}}
創建了一個嵌套字典並從中刪除了以后不需要的所有鍵。
a_list = [
{'ID': 'a', 'Animal': 'dog', 'color': 'white', 'tail': 'yes'},
{'ID': 'a', 'Animal': 'cat', 'color': 'black', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'bird', 'color': 'black', 'tail': 'no'},
{'ID': 'b', 'Animal': 'cat', 'color': 'pink', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'dog', 'color': 'yellow', 'tail': 'no'}
]
a_dict = {}
for a in a_list:
if a['ID'] in a_dict:
a_dict[a['ID']][a['Animal']] = a
else:
a_dict[a['ID']] = {a['Animal']: a}
for id_ in a_dict:
for animal in a_dict[id_]:
del a_dict[id_][animal]['ID']
del a_dict[id_][animal]['Animal']
輸出 :
>> a_dict
{'a': {'dog': {'color': 'white', 'tail': 'yes'},
'cat': {'color': 'black', 'tail': 'yes'}},
'b': {'bird': {'color': 'black', 'tail': 'no'},
'cat': {'color': 'pink', 'tail': 'yes'},
'dog': {'color': 'yellow', 'tail': 'no'}}}
a_list = [
{'ID': 'a', 'Animal': 'dog', 'color': 'white', 'tail': 'yes'},
{'ID': 'a', 'Animal': 'cat', 'color': 'black', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'bird', 'color': 'black', 'tail': 'no'},
{'ID': 'b', 'Animal': 'cat', 'color': 'pink', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'dog', 'color': 'yellow', 'tail': 'no'}
]
a_dict = {}
for v in a_list:
a_dict.setdefault(v['ID'], {}).setdefault(v['Animal'], {}).update(color=v['color'], tail=v['tail'])
from pprint import pprint
pprint(a_dict)
印刷:
{'a': {'cat': {'color': 'black', 'tail': 'yes'},
'dog': {'color': 'white', 'tail': 'yes'}},
'b': {'bird': {'color': 'black', 'tail': 'no'},
'cat': {'color': 'pink', 'tail': 'yes'},
'dog': {'color': 'yellow', 'tail': 'no'}}}
使用defaultdict
的簡單解決方案
from collections import defaultdict
result = defaultdict(dict)
a_list = [
{'ID': 'a', 'Animal': 'dog', 'color': 'white', 'tail': 'yes'},
{'ID': 'a', 'Animal': 'cat', 'color': 'black', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'bird', 'color': 'black', 'tail': 'no'},
{'ID': 'b', 'Animal': 'cat', 'color': 'pink', 'tail': 'yes'},
{'ID': 'b', 'Animal': 'dog', 'color': 'yellow', 'tail': 'no'}
]
for item in a_list:
result[item['ID']][item['Animal']] = {'color':item['color'], 'tail':item['tail']}
defaultdict(dict,
{'a': {'dog': {'color': 'white', 'tail': 'yes'},
'cat': {'color': 'black', 'tail': 'yes'}},
'b': {'bird': {'color': 'black', 'tail': 'no'},
'cat': {'color': 'pink', 'tail': 'yes'},
'dog': {'color': 'yellow', 'tail': 'no'}}})
與此處提供的其他解決方案非常相似,它使用默認字典。
>>> from collections import defaultdict
>>> d = defaultdict(dict)
>>> for dict_ in a_list:
ID = dict_.pop('ID')
animal = dict_.pop('Animal')
d[ID][animal] = dict_
>>> from pprint import pprint
>>> pprint(dict(d))
{'a': {'cat': {'color': 'black', 'tail': 'yes'},
'dog': {'color': 'white', 'tail': 'yes'}},
'b': {'bird': {'color': 'black', 'tail': 'no'},
'cat': {'color': 'pink', 'tail': 'yes'},
'dog': {'color': 'yellow', 'tail': 'no'}}}
您可以構建字典,在每一步迭代創建內部字典。 以下是一般情況的建議實現:
def group_by_nested_keys(elements, keys):
root = {}
for element in elements:
inner_dict = root
# Create the inner dictionaries as we go through the keys
# and remove the key from element at the same time
for key in keys[:-1]:
inner_dict = inner_dict.setdefault(element.pop(key), {})
# When all the dictionaries for the elements are created,
# assign the element to the most inner dictionary
inner_dict[element.pop(keys[-1])] = element
return root
a_dict = group_by_nested_keys(a_list, ["ID", "Animal"])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.