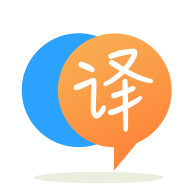
[英]whenever i run this it give me TypeError: can only concatenate str (not “bytes”) to str and i don't know how to fix it?
[英]Python Socket: Can only concatenate str not bytes to str. How to encode so it won't give me this error?
這是我的整個代碼:它使用用戶輸入作為主機和端口。
服務器端代碼:
import socket
import subprocess, os
print("#####################")
print("# Python Port Maker #")
print("# #")
print("#'To Go Boldy Where'#")
print("# No Other Python #")
print("# Has Gone #")
print("# By Riley #")
print("#####################")
print(' [*] Be Sure To use https://github.com/Thman558/Just-A-Test/blob/master/socket%20client.py
on the other machine')
host = input(" [*] What host would you like to use? ")
port = int(input(" [*] What port would you like to use? "))
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
server_socket.bind((host, port))
server_socket.listen(5)
print("\n[*] Listening on port " +str(port)+ ", waiting for connections.")
client_socket, (client_ip, client_port) = server_socket.accept()
print("[*] Client " +client_ip+ " connected.\n")
while True:
try:
command = input(client_ip+ "> ")
if(len(command.split()) != 0):
client_socket.send(b'command')
else:
continue
except(EOFError):
print("ERROR INPUT NOT FOUND. Please type 'help' to get a list of commands.\n")
continue
if(command == "quit"):
break
data = client_socket.recv(1024)
print(data + "\n")
client_socket.close()
再次報錯:
print(:"Recieved Command : ' +command)
TypeError: can't concat str to bytes
當用戶僅在給定命令發生此錯誤時才連接時,它工作正常,不確定它是否可能是data
變量,但此代碼只是不想工作,哈哈。 這是我用來連接客戶端的代碼:
import socket
import subprocess, os
print("######################")
print("# #")
print("# The Socket #")
print("# Connecter #")
print("# #")
print("# By Yo Boi #")
print("# Riley #")
print("######################")
host = input("What host would you like to connect to? ")
port = int(input("What port is the server using? "))
connection_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
connection_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
connection_socket.connect((host, port))
print("\n[*] Connected to " +host+ " on port " +str(port)+ ".\n")
while True:
command = connection_socket.recv(1024)
split_command = command.split()
print("Received command : " +command)
if command == "quit":
break
if(command.split()[0] == "cd"):
if len(command.split()) == 1:
connection_socket.send((os.getcwd()))
elif len(command.split()) == 2:
try:
os.chdir(command.split()[1])
connection_socket.send(("Changed directory to " + os.getcwd()))
except(WindowsError):
connection_socket.send(str.encode("No such directory : " +os.getcwd()))
else:
proc = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, stdin=subprocess.PIPE)
stdout_value = proc.stdout.read() + proc.stderr.read()
print(stdout_value + "\n")
if(stdout_value != ""):
connection_socket.send(stdout_value)
else:
connection_socket.send(command+ " does not return anything")
connection_socket.close()
如果你打印type(data)
你會注意到它不是一個字符串而是一個字節實例。 要連接換行符,您可以這樣寫:
print(data + b"\n")
socket.recv(size)
返回一個字節對象,您試圖將其附加到字符串中,這導致了錯誤。 您可以通過在附加換行符之前執行str(data , 'utf-8')
將套接字消息轉換為字符串
無論如何, print()
方法都會添加一個換行符,這樣您就可以只編寫print(data)
就可以了
因此,除了數據類型不匹配之外,您的腳本還有很多錯誤。 我會告訴你str
和byte
不匹配有什么問題。 每當您將字符串轉換為字節時,您必須指定編碼方法。 無論如何,我已經修復了你的腳本,剩下的事情就交給你去解決了。 ;)
服務器腳本
import socket
print("#####################")
print("# Python Port Maker #")
print("# #")
print("#'To Go Boldy Where'#")
print("# No Other Python #")
print("# Has Gone #")
print("# By Riley #")
print("#####################")
print(' [*] Be Sure To use https://github.com/Thman558/Just-A-Test/blob/master/socket%20client.py on the other machine')
host = input(" [*] What host would you like to use? ")
port = int(input(" [*] What port would you like to use? "))
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
server_socket.bind((host, port))
server_socket.listen(5)
print("\n[*] Listening on port " + str(port) + ", waiting for connections.")
client_socket, (client_ip, client_port) = server_socket.accept()
print("[*] Client " + client_ip + " connected.\n")
while True:
try:
command = input(client_ip + "> ")
if len(command.split()) != 0:
client_socket.send(bytes(command, 'utf-8'))
else:
continue
except EOFError:
print("ERROR INPUT NOT FOUND. Please type 'help' to get a list of commands.\n")
continue
if command == "quit":
break
data = client_socket.recv(1024).decode()
print(data + "\n")
client_socket.close()
客戶端腳本
import os
import socket
import subprocess
print("######################")
print("# #")
print("# The Socket #")
print("# Connecter #")
print("# #")
print("# By Yo Boi #")
print("# Riley #")
print("######################")
host = input("What host would you like to connect to? ")
port = int(input("What port is the server using? "))
connection_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
connection_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
connection_socket.connect((host, port))
print("\n[*] Connected to " + host + " on port " + str(port) + ".\n")
while True:
print('\nWaiting for a command....')
command = connection_socket.recv(1024).decode()
split_command = command.split()
print("Received command : ", command)
if command == "quit":
break
if command[:2] == "cd":
if len(command.split()) == 1:
connection_socket.send(bytes(os.getcwd(), 'utf-8'))
elif len(command.split()) == 2:
try:
os.chdir(command.split()[1])
connection_socket.send(bytes("Changed directory to " + os.getcwd(), 'utf-8'))
except WindowsError:
connection_socket.send(str.encode("No such directory : " + os.getcwd()))
else:
proc = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE,
stdin=subprocess.PIPE)
stdout_value = proc.stdout.read() + proc.stderr.read()
print(str(stdout_value) + "\n")
if stdout_value != "":
connection_socket.send(stdout_value)
else:
connection_socket.send(bytes(str(command) + ' does not return anything', 'utf-8'))
connection_socket.close()
提示:大多數錯誤都包含邏輯缺陷!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.