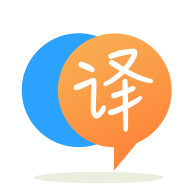
[英]how to reload a collectionview that is inside a tableviewcell
[英]Swift: How to display a CollectionView inside a TableViewCell Programmatically
此代碼在 tableViewCell 中顯示一個 collectionView,其中兩者都已添加到 StoryBoard 中:
class TableViewCell: UITableViewCell {
@IBOutlet weak var collectionView: UICollectionView!
override func awakeFromNib() {
super.awakeFromNib()
collectionView.delegate = self
collectionView.dataSource = self
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
}
extension TableViewCell: UICollectionViewDelegate, UICollectionViewDataSource, UICollectionViewDelegateFlowLayout {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 1
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "collectionViewCell", for: indexPath)
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: 139, height: 64)
}
在我的應用程序中,我以編程方式創建 CollectionView 和 TableView。
以編程方式創建 collectionView:
class collectionViews {
static func collectionViewOne() -> UICollectionView {
let flowLayout = CarouselFlowLayout()
let collectionViewOne = UICollectionView(frame: CGRect(x: 106, y: 313, width: 1708, height: 300), collectionViewLayout: flowLayout)
return collectionViewOne
}
}
在 tableViewCell 中實例化 collectionView:
class TableViewCell2: UITableViewCell {
var moviesItems: [movieItem] = []
let cellIdentifier = "movieCardCell"
let collectionViewOne = collectionViews.collectionViewOne()
private func setupCollectionView(){
collectionViewOne.delegate = self
collectionViewOne.dataSource = self
collectionViewOne.backgroundColor = UIColor (hex: "444A64")
collectionViewOne.register(UICollectionViewCell.self, forCellWithReuseIdentifier: "MyCell")
let nib = UINib(nibName: "movieCardCell", bundle: nil)
collectionViewOne.register(nib, forCellWithReuseIdentifier: cellIdentifier)
}
}
// These functions never get called
extension TableViewCell: UICollectionViewDelegate, UICollectionViewDataSource, UICollectionViewDelegateFlowLayout {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
print("INSIDE TableViewCell2.collectionView 1")
return 1
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "collectionViewCell", for: indexPath)
print("INSIDE TableViewCell2.collectionView 2")
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
// For some reason he chose the measures of collectionViewCell and substracted 2
print("INSIDE TableViewCell2.collectionView 3")
return CGSize(width: 139, height: 64)
}
}
注意:委托函數永遠不會被調用。 所以 collectionView 從未被添加到 tableViewCell 中?
以編程方式創建 TableView:
class sectionTableCell: TableViewCell2 {
}
class MoviesViewController4: UIViewController {
let tableView = UITableView()
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = UIColor (hex: "444A64")
tableView.delegate = self
tableView.dataSource = self
tableView.register(sectionTableCell.self, forCellReuseIdentifier: "tableViewCell")
displayTableView2()
}
func displayTableView2() {
self.view.addSubview(tableView)
tableView.translatesAutoresizingMaskIntoConstraints = false
tableView.topAnchor.constraint(equalTo: view.topAnchor).isActive = true
tableView.leftAnchor.constraint(equalTo: view.leftAnchor).isActive = true
tableView.bottomAnchor.constraint(equalTo: view.bottomAnchor).isActive = true
tableView.rightAnchor.constraint(equalTo: view.rightAnchor).isActive = true
}
}
extension MoviesViewController4: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 1
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell =
tableView.dequeueReusableCell(withIdentifier: "tableViewCell", for: indexPath) as? sectionTableCell
else {
fatalError("Unable to create explore table view cell")}
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 140
}
}
所以問題是:如何將我以編程方式創建的 collectionView 添加到我也以編程方式創建的 tableViewCell 中?
據我所知,您不會在代碼中的任何地方調用setupCollectionView()
方法。 您應該在 UITableViewCell 的init
方法中執行此操作。 將此代碼添加到您的單元格類:
override init(frame: CGRect) {
super.init(frame: frame)
setupCollectionView()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
setupCollectionView()
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.