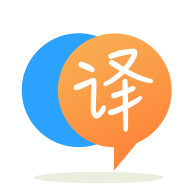
[英]ArrayList not displaying in RecyclerView but no errors shown
[英]RecyclerView not displaying ArrayList correctly
出於某種原因,我的 RecyclerView 中顯示的唯一內容是 com.stu54259.plan2cook.Model.Shopping_list@5cb7482 重復了各種結束代碼而不是 ArrayList 的內容。 任何建議都必須與 recylerview 適配器有關。 如果需要,可以添加 xml 等,但我確定我剛剛錯過了一些愚蠢的事情。
Shopping_List 類
package com.stu54259.plan2cook.Model;
public class Shopping_List {
private int id;
private String ingredient_type;
private String ingredient_name;
private Double quantity;
private String measurement_name;
public Shopping_List() {
}
public Shopping_List(String ingredient_type, String ingredient_name, Double quantity, String measurement_name) {
this.ingredient_type = ingredient_type;
this.ingredient_name = ingredient_name;
this.quantity = quantity;
this.measurement_name = measurement_name;
}
public Shopping_List(int id, String ingredient_type, String ingredient_name, Double quantity, String measurement_name) {
this.id = id;
this.ingredient_type = ingredient_type;
this.ingredient_name = ingredient_name;
this.quantity = quantity;
this.measurement_name = measurement_name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getIngredient_type() {
return ingredient_type;
}
public void setIngredient_type(String ingredient_type) {
this.ingredient_type = ingredient_type;
}
public String getIngredient_name() {
return ingredient_name;
}
public void setIngredient_name(String ingredient_name) {
this.ingredient_name = ingredient_name;
}
public Double getQuantity() {
return quantity;
}
public void setQuantity(Double quantity) {
this.quantity = quantity;
}
public String getMeasurement_name() {
return measurement_name;
}
public void setMeasurement_name(String measurement_name) {
this.measurement_name = measurement_name;
}
}
活動
public class ShoppingList extends MainActivity {
ShoppingListAdapter adapterRecipe;
List<Shopping_List> shopList = new ArrayList<>();
RecyclerView listIngredient;
SQLiteDatabase db;
Cursor c;
EditText edittext;
String search;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.shopping_list);
edittext = findViewById(R.id.editPlanName);
edittext.setOnKeyListener(new View.OnKeyListener() {
public boolean onKey(View v, int keyCode, KeyEvent event) {
search = edittext.getText().toString();
Log.d("Search value", search);
if ((event.getAction() == KeyEvent.ACTION_DOWN) &&
(keyCode == KeyEvent.KEYCODE_ENTER)) {
loadIngredient();
adapterRecipe.notifyDataSetChanged();
return true;
}
return false;
}
});
BottomNavigationView navigation = (BottomNavigationView) findViewById(R.id.navigation);
navigation.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()) {
case R.id.home:
Intent a = new Intent(ShoppingList.this,MainActivity.class);
startActivity(a);
break;
case R.id.recipes:
Intent b = new Intent(ShoppingList.this,RecipeSearch.class);
startActivity(b);
break;
case R.id.shoppingList:
Intent c = new Intent(ShoppingList.this, ShoppingList.class);
startActivity(c);
break;
case R.id.mealPlan:
Intent d = new Intent(ShoppingList.this, MenuPlan.class);
startActivity(d);
break;
case R.id.reminder:
Intent e = new Intent(ShoppingList.this, Reminders.class);
startActivity(e);
break;
}
return false;
}
});
adapterRecipe = new ShoppingListAdapter(this, shopList);
listIngredient = findViewById(R.id.listIngredient);
RecyclerView.LayoutManager mLayoutManager = new LinearLayoutManager(this,
LinearLayoutManager.VERTICAL, false);
listIngredient.setLayoutManager(mLayoutManager);
listIngredient.setItemAnimator(new DefaultItemAnimator());
listIngredient.setAdapter(adapterRecipe);
}
public void loadIngredient() {
shopList.clear();
db = (new DatabaseManager(this).getWritableDatabase());
String RECIPE_SEARCH =
"SELECT SUM(A.ingredient_quantity) quantity, A.ingredient ingredient_name, A.recipe, B.ingredient_type, B.measurement_name, C.id, D.plan_name " +
"FROM " + DatabaseManager.TABLE_QUANTITY + " AS A JOIN " + DatabaseManager.TABLE_INGREDIENTS + " AS B ON A.ingredient = B.ingredient_name " +
"JOIN " + DatabaseManager.TABLE_PLAN_RECIPES + " AS C ON A.recipe = C.recipe_name " +
"JOIN " + DatabaseManager.TABLE_MEAL_PLAN + " AS D ON C.id = D.plan_recipe " +
"WHERE D.plan_name LIKE ? GROUP BY A.ingredient";
Log.d("Search query", RECIPE_SEARCH);
c = db.rawQuery(RECIPE_SEARCH, new String[]{"%" + search + "%"});
if (c.moveToFirst()) {
do {
Shopping_List shopping_list = new Shopping_List();
shopping_list.setQuantity(c.getDouble(c.getColumnIndex("quantity")));
shopping_list.setIngredient_name(c.getString(c.getColumnIndex("ingredient_name")));
shopping_list.setIngredient_type(c.getString(c.getColumnIndex("ingredient_type")));
shopping_list.setMeasurement_name(c.getString(c.getColumnIndex("measurement_name")));
shopList.add(shopping_list);
} while (c.moveToNext());
}
c.close();
db.close();
}
}
適配器
public class ShoppingListAdapter extends RecyclerView.Adapter<com.stu54259.plan2cook.Adapters.ShoppingListAdapter.ViewHolder> {
private List<Shopping_List> shopList;
private LayoutInflater mInflater;
private com.stu54259.plan2cook.Adapters.RecyclerViewAdapter.ItemClickListener mClickListener;
// data is passed into the constructor
public ShoppingListAdapter(Context context, List<Shopping_List> data) {
this.mInflater = LayoutInflater.from(context);
this.shopList = data;
}
// inflates the row layout from xml when needed
@Override
public com.stu54259.plan2cook.Adapters.ShoppingListAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = mInflater.inflate(R.layout.fragment_item, parent, false);
return new com.stu54259.plan2cook.Adapters.ShoppingListAdapter.ViewHolder(view);
}
// binds the data to the TextView in each row
@Override
public void onBindViewHolder(com.stu54259.plan2cook.Adapters.ShoppingListAdapter.ViewHolder holder, int position) {
if(shopList.get(position) != null)
{
holder.myTextView.setText(shopList.get(position).toString());
}
}
// total number of rows
@Override
public int getItemCount() {
return shopList.size();
}
// stores and recycles views as they are scrolled off screen
public class ViewHolder extends RecyclerView.ViewHolder implements View.OnClickListener {
TextView myTextView;
ViewHolder(View itemView) {
super(itemView);
myTextView = itemView.findViewById(R.id.quantity);
itemView.setOnClickListener(this);
}
@Override
public void onClick(View view) {
if (mClickListener != null) mClickListener.onItemClick(view, getAdapterPosition());
}
}
// allows clicks events to be caught
void setClickListener(com.stu54259.plan2cook.Adapters.RecyclerViewAdapter.ItemClickListener itemClickListener) {
this.mClickListener = itemClickListener;
}
// parent activity will implement this method to respond to click events
public interface ItemClickListener {
void onItemClick(View view, int position);
}
}
在您的Shopping_List
類中添加此方法,因此當您對Shopping_List
實例使用toString()
,您將獲得其所有屬性以空格分隔:
public String toString() {
return ingredient_name + " " + ingredient_type + " " + quantity + " " + measurement_name;
}
您可以更改屬性的順序。
您在 onBindViewHolder 方法中有錯誤的代碼。 您應該使用 Shopping_List 對象中的某些字段設置文本:
@Override
public void onBindViewHolder(com.stu54259.plan2cook.Adapters.ShoppingListAdapter.ViewHolder holder, int position) {
if(shopList.get(position) != null)
{
holder.myTextView.setText(shopList.get(position).toString());
}
}
你還沒有把Shopping_List
對象放在這里,但如果你有這樣的東西:
public class Shopping_List {
public String title;
public String getTitle() {
return title;
}
}
那么你應該做這樣的事情:
@Override
public void onBindViewHolder(com.stu54259.plan2cook.Adapters.ShoppingListAdapter.ViewHolder holder, int position) {
if(shopList.get(position) != null)
{
holder.myTextView.setText(shopList.get(position).getTitle());
}
}
雖然它沒有給出直接的解決方案,但我建議您使用 groupie 庫。 它很可能會消除您的錯誤並減少樣板代碼和復雜性。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.